11.16 LAB: Course information (derived classes) Given main(), define a Course base class with methods to set and get private fields of the following types: • String to store the course number • String to store the course title Define Course's PrintInfo() method that outputs the course number and title. Then, define a derived class offered Course with methods to set and get private fields of the following types: • String to store the instructor's name • String to store the location • String to store the class time Ex. If the input is: ECE287 Digital Systems Design ECE387
I need help with creating a Java program described in the image below:
CourseInformation.java:
import java.util.Scanner;
public class CourseInformation {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
Course myCourse = new Course();
OfferedCourse myOfferedCourse = new OfferedCourse();
String courseNumber, courseTitle;
String oCourseNumber, oCourseTitle, instructorName, location, classTime;
courseNumber = scnr.nextLine();
courseTitle = scnr.nextLine();
oCourseNumber = scnr.nextLine();
oCourseTitle = scnr.nextLine();
instructorName = scnr.nextLine();
location = scnr.nextLine();
classTime = scnr.nextLine();
myCourse.setCourseNumber(courseNumber);
myCourse.setCourseTitle(courseTitle);
myCourse.printInfo();
myOfferedCourse.setCourseNumber(oCourseNumber);
myOfferedCourse.setCourseTitle(oCourseTitle);
myOfferedCourse.setInstructorName(instructorName);
myOfferedCourse.setLocation(location);
myOfferedCourse.setClassTime(classTime);
myOfferedCourse.printInfo();
System.out.println(" Instructor Name: " + myOfferedCourse.getInstructorName());
System.out.println(" Location: " + myOfferedCourse.getLocation());
System.out.println(" Class Time: " + myOfferedCourse.getClassTime());
}
}
Course.java:
public class Course{
// TODO: Declare private fields
// TODO: Define mutator methods -
// setCourseNumber(), setCourseTitle()
// TODO: Define accessor methods -
// getCourseNumber(), getCourseTitle()
// TODO: Define printInfo()
}
OfferedCourse.java:
public class OfferedCourse extends Course {
// TODO: Declare private fields
// TODO: Define mutator methods -
// setInstructorName(), setLocation(), setClassTime()
// TODO: Define accessor methods -
// getInstructorName(), getLocation(), getClassTime()
}


Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 5 images

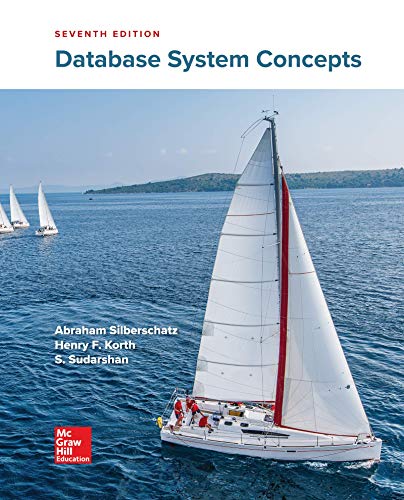
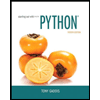
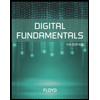
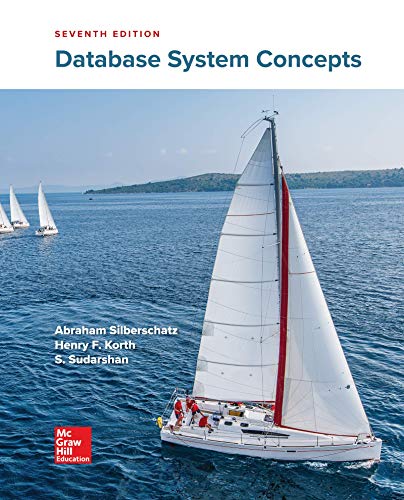
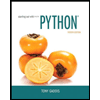
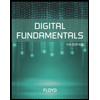
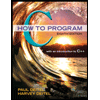
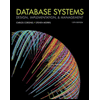
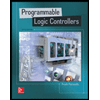