I have the following code in java, replit: //First class public class Main { public static void main(String[] args) { Scanner console = new Scanner(System.in); Random random = new Random(); Game game = new Game(console, random); game.startGame(); console.close(); } } //Second class import java.util.Scanner; public class Player { private String name; private int totalGames; private int totalGuesses; private int bestGame; public Player() { this.name = null; this.totalGames = 0; this.totalGuesses = 0; this.bestGame = Integer.MAX_VALUE; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getTotalGames() { return totalGames; } public void setTotalGames(int totalGames) { this.totalGames = totalGames; } public int getTotalGuesses() { return totalGuesses; } public void setTotalGuesses(int totalGuesses) { this.totalGuesses = totalGuesses; } public int getBestGame() { return bestGame; } public void setBestGame(int bestGame) { this.bestGame = bestGame; } } //Third class import java.util.Scanner; import java.util.Random; public class Game { public static final int MAX = 100; private final Scanner console; private final Random random; public Game(Scanner console, Random random) { this.console = console; this.random = random; } public void startGame() { int totalGames = 0; int totalGuesses = 0; int bestGame = Integer.MAX_VALUE; Player player = new Player(); while (true) { int answer = 1 + random.nextInt(MAX); int guesses = playGame(console, answer); totalGames++; totalGuesses += guesses; bestGame = Math.min(bestGame, guesses); player.setTotalGames(totalGames); player.setTotalGuesses(totalGuesses); player.setBestGame(bestGame); System.out.println("Play again y/n?"); String playAgain = console.next().toLowerCase(); if (!playAgain.startsWith("y")) { break; } } printOverallStatistics(player.getTotalGames(), player.getTotalGuesses(), player.getBestGame()); } public int playGame(Scanner console, int answer) { System.out.println("I'm thinking of a number between 1 and " + Game.MAX + "..."); int guesses = 0; while (true) { System.out.print("Your guess? "); if (console.hasNextInt()) { int guess = console.nextInt(); guesses++; if (guess == answer) { System.out.println("You got it right in " + guesses + " guess" + (guesses == 1 ? "!" : "es!")); break; } else if (guess < answer) { System.out.println("It's higher."); } else { System.out.println("It's lower."); } } else { System.out.println("Invalid input. Please enter a valid integer."); console.next(); } } return guesses; } private void printOverallStatistics(int totalGames, int totalGuesses, int bestGame) { System.out.println("Total games: " + totalGames); System.out.println("Total guesses: " + totalGuesses); System.out.println("Guesses/game: " + (totalGames == 0 ? 0 : totalGuesses / totalGames)); System.out.println("Best game: " + bestGame); } public static void main(String[] args) { Scanner console = new Scanner(System.in); Random random = new Random(); Game game = new Game(console, random); game.startGame(); console.close(); } } Now I've created a player_results.csv file. I want help with adding code to my existing class so that it reads from the csv file(which can be updated by just editing it, example in attachment below). Then, convert those strings into a player object you can use to resume a series of games in the console. In other words, update the players statistics as though they were resuming from the state they last left the guessing game. It should work for all players you add in the file. You must use these: java.io.File java.io.FileWriter java.util.Scanner java.util.Random java.util.UUID Must use FileWriter not bufferedfilewriter or anything else, and Arrays.
I have the following code in java, replit:
//First class
public class Main {
public static void main(String[] args) {
Scanner console = new Scanner(System.in);
Random random = new Random();
Game game = new Game(console, random);
game.startGame();
console.close();
}
}
//Second class
import java.util.Scanner;
public class Player {
private String name;
private int totalGames;
private int totalGuesses;
private int bestGame;
public Player() {
this.name = null;
this.totalGames = 0;
this.totalGuesses = 0;
this.bestGame = Integer.MAX_VALUE;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getTotalGames() {
return totalGames;
}
public void setTotalGames(int totalGames) {
this.totalGames = totalGames;
}
public int getTotalGuesses() {
return totalGuesses;
}
public void setTotalGuesses(int totalGuesses) {
this.totalGuesses = totalGuesses;
}
public int getBestGame() {
return bestGame;
}
public void setBestGame(int bestGame) {
this.bestGame = bestGame;
}
}
//Third class
import java.util.Scanner;
import java.util.Random;
public class Game {
public static final int MAX = 100;
private final Scanner console;
private final Random random;
public Game(Scanner console, Random random) {
this.console = console;
this.random = random;
}
public void startGame() {
int totalGames = 0;
int totalGuesses = 0;
int bestGame = Integer.MAX_VALUE;
Player player = new Player();
while (true) {
int answer = 1 + random.nextInt(MAX);
int guesses = playGame(console, answer);
totalGames++;
totalGuesses += guesses;
bestGame = Math.min(bestGame, guesses);
player.setTotalGames(totalGames);
player.setTotalGuesses(totalGuesses);
player.setBestGame(bestGame);
System.out.println("Play again y/n?");
String playAgain = console.next().toLowerCase();
if (!playAgain.startsWith("y")) {
break;
}
}
printOverallStatistics(player.getTotalGames(), player.getTotalGuesses(), player.getBestGame());
}
public int playGame(Scanner console, int answer) {
System.out.println("I'm thinking of a number between 1 and " + Game.MAX + "...");
int guesses = 0;
while (true) {
System.out.print("Your guess? ");
if (console.hasNextInt()) {
int guess = console.nextInt();
guesses++;
if (guess == answer) {
System.out.println("You got it right in " + guesses + " guess" + (guesses == 1 ? "!" : "es!"));
break;
} else if (guess < answer) {
System.out.println("It's higher.");
} else {
System.out.println("It's lower.");
}
} else {
System.out.println("Invalid input. Please enter a valid integer.");
console.next();
}
}
return guesses;
}
private void printOverallStatistics(int totalGames, int totalGuesses, int bestGame) {
System.out.println("Total games: " + totalGames);
System.out.println("Total guesses: " + totalGuesses);
System.out.println("Guesses/game: " + (totalGames == 0 ? 0 : totalGuesses / totalGames));
System.out.println("Best game: " + bestGame);
}
public static void main(String[] args) {
Scanner console = new Scanner(System.in);
Random random = new Random();
Game game = new Game(console, random);
game.startGame();
console.close();
}
}
Now I've created a player_results.csv file. I want help with adding code to my existing class so that it reads from the csv file(which can be updated by just editing it, example in attachment below). Then, convert those strings into a player object you can use to resume a series of games in the console. In other words, update the players statistics as though they were resuming from the state they last left the guessing game. It should work for all players you add in the file. You must use these:
- java.io.File
- java.io.FileWriter
- java.util.Scanner
- java.util.Random
- java.util.UUID
Must use FileWriter not bufferedfilewriter or anything else, and Arrays.


Trending now
This is a popular solution!
Step by step
Solved in 3 steps

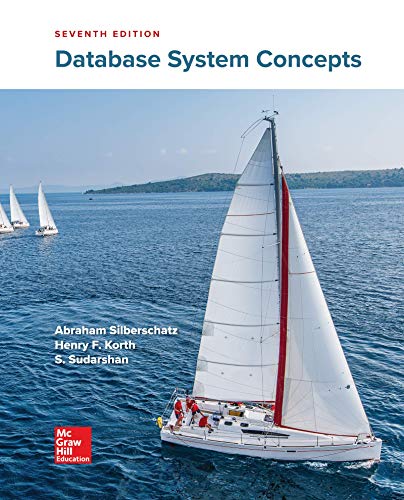
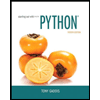
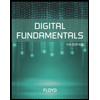
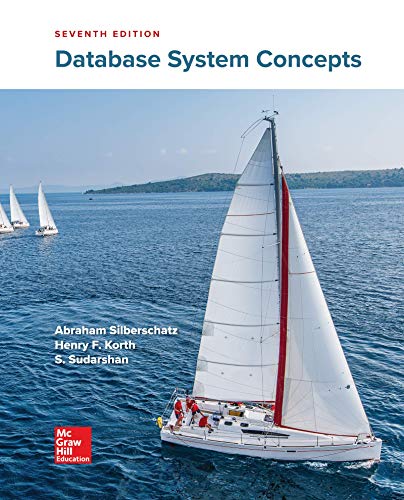
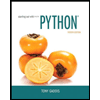
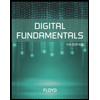
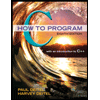
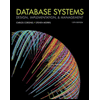
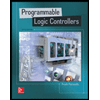