A new Java project InheritanceDemo, and two additional classes: Box.java and KleenexBox.java. InheritanceDemo.java: // StudentName Today's Date public class InheritanceDemo { public static void main(String[] args) { KleenexBox kb1 = new KleenexBox(); KleenexBox kb2 = new KleenexBox(100); System.out.println("kb1 has " + kb1.numTissues + " tissues and is " + kb1.length + " by " + kb1.width); System.out.println("kb2:" + kb2); } } Box.java: // Student Name Today's Date public class Box { public double width; public double length; public String label; } KleenexBox.java: // Student's Name Today's Date public class KleenexBox extends Box { public int numTissues; //A new Kleenex box public KleenexBox() { numTissues = 50; length = 8.0; width = 5.0; } //A new Kleenex box with a specific number of tissues public KleenexBox(int numberOfTissues) { numTissues = numberOfTissues; length = 8.0; width = 5.0; } public String toString() { return ("This box is " + length + " by " + width + " and has " + numTissues + " tissues."); } } Questions: 4.1. What type of variables are kb1 and kb2: Box or KleenexBox? 4.2. How does the programmer specify that KleenexBox is a type of Box? 4.3. Notice how KleenexBox objects have a length and a width, but those fields are not declared in the KleenexBox class. Where do the length and width fields come from? 4.4. What additional fields and/or methods does a KleenexBox have that a Box does not? 4.5. Suppose you wanted a new type of box called ShippingBox. Which of the following would you use to declare that class? (Choose one.) 4.5.a. public class ShippingBox extends Box {} 4.5.b public class Box extends ShippingBox {} 4.5.c. public class ShippingBox IS_A Box {} 4.5.d. public class ShippingBox { box A; }
A new Java project InheritanceDemo, and two additional classes: Box.java and KleenexBox.java.
InheritanceDemo.java:
// StudentName Today's Date
public class InheritanceDemo {
public static void main(String[] args) {
KleenexBox kb1 = new KleenexBox();
KleenexBox kb2 = new KleenexBox(100);
System.out.println("kb1 has " + kb1.numTissues + " tissues and is " + kb1.length + " by " + kb1.width);
System.out.println("kb2:" + kb2);
}
}
Box.java:
// Student Name Today's Date
public class Box {
public double width;
public double length;
public String label;
}
KleenexBox.java:
// Student's Name Today's Date
public class KleenexBox extends Box {
public int numTissues;
//A new Kleenex box
public KleenexBox() {
numTissues = 50;
length = 8.0;
width = 5.0;
}
//A new Kleenex box with a specific number of tissues
public KleenexBox(int numberOfTissues) {
numTissues = numberOfTissues;
length = 8.0;
width = 5.0;
}
public String toString() {
return ("This box is " + length + " by " + width + " and has " + numTissues + " tissues.");
}
}
Questions:
4.1. What type of variables are kb1 and kb2: Box or KleenexBox?
4.2. How does the programmer specify that KleenexBox is a type of Box?
4.3. Notice how KleenexBox objects have a length and a width, but those fields are not declared in the KleenexBox class. Where do the length and width fields come from?
4.4. What additional fields and/or methods does a KleenexBox have that a Box does not?
4.5. Suppose you wanted a new type of box called ShippingBox. Which of the following would you use to declare that class? (Choose one.)
4.5.a. public class ShippingBox extends Box {}
4.5.b public class Box extends ShippingBox {}
4.5.c. public class ShippingBox IS_A Box {}
4.5.d. public class ShippingBox { box A; }
Rubric:
Student name is a comment on the first line of each .class file: -5 points if failed
Questions: 5 points, 1 point per question
Screenshot and source code: 5 points
Please paste a screenshot of a successful

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

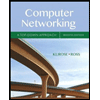
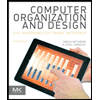
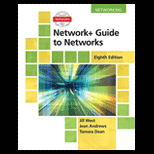
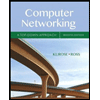
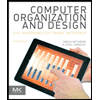
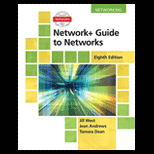
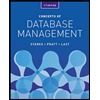
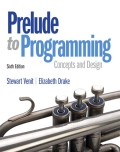
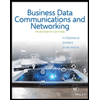