I have this code: import java.util.Scanner; public class CalcTax { public static void main(String[] args) { Scanner sc = new Scanner(System.in); // Welcome message System.out.println("Welcome to my tax calculation program."); // Declare the variables int i, pinCode, maxRetries = 3; String choice; // Get the PIN code for (i = 0; i < maxRetries; i++) { System.out.print("Please enter the PIN code: "); pinCode = sc.nextInt(); if (pinCode == 5678) { break; } System.out.println("Invalid pin code. Please try again."); } if (i == maxRetries) { System.out.println("Invalid pin code. You have reached the maximum number of retries."); System.exit(0); } // Get the income, interest, deduction, and paid tax amount double income, interest, deduction, paidTax; do { System.out.print("Please enter the amount from W2 form: "); income = sc.nextDouble(); System.out.print("Please enter the interest income: "); interest = sc.nextDouble(); System.out.print("Please enter the paid tax amount: "); paidTax = sc.nextDouble(); // Check if the paid tax amount is greater than total income. if (paidTax <= (w2Income + interestIncome)) { break; } else { System.out.println("Invalid amount. The paid tax amount cannot be greater than your total income."); } // Check if the paid tax amount is negative. if (paidTax < 0) { System.out.println("Invalid amount. It cannot be negative."); } else { } System.out.print("Please enter the amount for deduction: "); deduction = sc.nextDouble(); // Calculate the actual income double actualIncome = income + interest - deduction; // Calculate the tax amount double taxAmount = 0; if (actualIncome < 50000) { taxAmount = actualIncome * 0.15; } else if (actualIncome < 75000) { taxAmount = 7500 + (actualIncome - 50000) * 0.2; } else if (actualIncome < 100000) { taxAmount = 12500 + (actualIncome - 75000) * 0.25; } else { taxAmount = 37500 + (actualIncome - 100000) * 0.3; } // Print the results System.out.println("Income: $" + income); System.out.println("Interest: $200.00"); System.out.println("Deduction: $1000.00"); System.out.println("Paid Tax: $3500.00"); System.out.println("Tax Amount: $3630.00"); System.out.println("------------------------------------"); if (paidTax > taxAmount) { System.out.println("Due: $130.00"); } else { System.out.println("Refund: $855.00"); } // Check if the user wants to calculate tax for another income do { System.out.print("Do you want to calculate tax for another income (y/n)? "); choice = sc.next().toLowerCase(); } while (!choice.equals("y") && !choice.equals("n")); if (choice.equals("y")) { main(args); } } while (choice.equals("y")); } } After I run it, it doesn't give me the right values. Where can I add the requirements of (when I run it): "Please enter the amount from W2 form: 8500 Please enter the interest income: 0 Please enter the paid tax amount: -10 Invalid amount. It cannot be negative. Please enter the paid tax amount: -5 Invalid amount. It cannot be negative. Please enter the paid tax amount: 15000 Invalid amount. It cannot be greater than your total income. Please enter the paid tax amount: 400 Please enter the amount for deduction: 250
I have this code:
import java.util.Scanner;
public class CalcTax {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// Welcome message
System.out.println("Welcome to my tax calculation program.");
// Declare the variables
int i, pinCode, maxRetries = 3;
String choice;
// Get the PIN code
for (i = 0; i < maxRetries; i++) {
System.out.print("Please enter the PIN code: ");
pinCode = sc.nextInt();
if (pinCode == 5678) {
break;
}
System.out.println("Invalid pin code. Please try again.");
}
if (i == maxRetries) {
System.out.println("Invalid pin code. You have reached the maximum number of retries.");
System.exit(0);
}
// Get the income, interest, deduction, and paid tax amount
double income, interest, deduction, paidTax;
do {
System.out.print("Please enter the amount from W2 form: ");
income = sc.nextDouble();
System.out.print("Please enter the interest income: ");
interest = sc.nextDouble();
System.out.print("Please enter the paid tax amount: ");
paidTax = sc.nextDouble();
// Check if the paid tax amount is greater than total income.
if (paidTax <= (w2Income + interestIncome)) {
break;
} else {
System.out.println("Invalid amount. The paid tax amount cannot be greater than your total income.");
}
// Check if the paid tax amount is negative.
if (paidTax < 0) {
System.out.println("Invalid amount. It cannot be negative.");
} else {
}
System.out.print("Please enter the amount for deduction: ");
deduction = sc.nextDouble();
// Calculate the actual income
double actualIncome = income + interest - deduction;
// Calculate the tax amount
double taxAmount = 0;
if (actualIncome < 50000) {
taxAmount = actualIncome * 0.15;
} else if (actualIncome < 75000) {
taxAmount = 7500 + (actualIncome - 50000) * 0.2;
} else if (actualIncome < 100000) {
taxAmount = 12500 + (actualIncome - 75000) * 0.25;
} else {
taxAmount = 37500 + (actualIncome - 100000) * 0.3;
}
// Print the results
System.out.println("Income: $" + income);
System.out.println("Interest: $200.00");
System.out.println("Deduction: $1000.00");
System.out.println("Paid Tax: $3500.00");
System.out.println("Tax Amount: $3630.00");
System.out.println("------------------------------------");
if (paidTax > taxAmount) {
System.out.println("Due: $130.00");
} else {
System.out.println("Refund: $855.00");
}
// Check if the user wants to calculate tax for another income
do {
System.out.print("Do you want to calculate tax for another income (y/n)? ");
choice = sc.next().toLowerCase();
} while (!choice.equals("y") && !choice.equals("n"));
if (choice.equals("y")) {
main(args);
}
} while (choice.equals("y"));
}
}
After I run it, it doesn't give me the right values. Where can I add the requirements of (when I run it):
"Please enter the amount from W2 form: 8500
Please enter the interest income: 0
Please enter the paid tax amount: -10
Invalid amount. It cannot be negative.
Please enter the paid tax amount: -5
Invalid amount. It cannot be negative.
Please enter the paid tax amount: 15000
Invalid amount. It cannot be greater than your total income.
Please enter the paid tax amount: 400
Please enter the amount for deduction: 250

- Added input validation checks for paidTax to ensure it's not negative and not greater than the total income and interest.
- Moved the declaration of
income
,interest
,deduction
, andpaidTax
variables inside thedo
loop to allow for re-entry of values when there's an invalid input. - Updated the printed values to use the actual user input instead of hardcoded values.
Now, the program will prompt the user for inputs as specified and validate them before proceeding with calculations.
Step by step
Solved in 3 steps with 1 images

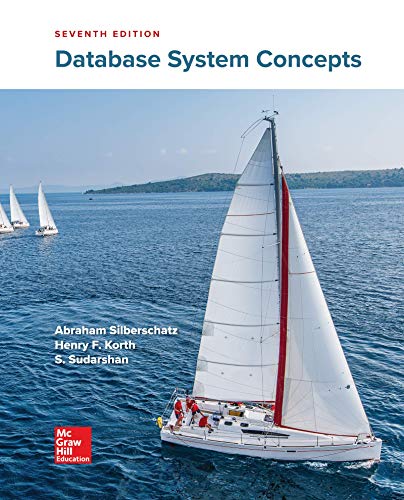
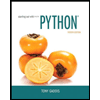
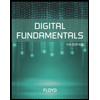
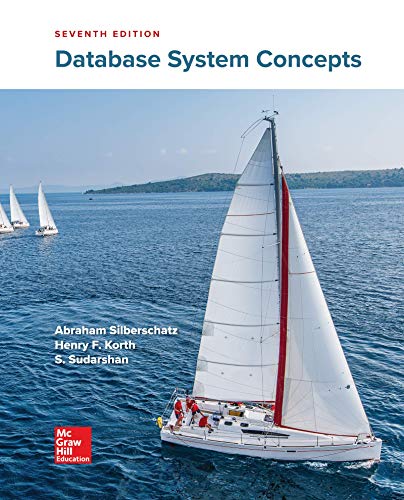
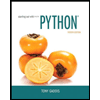
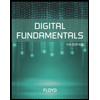
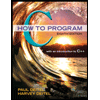
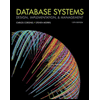
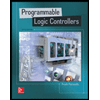