ValidatorString.java X Validator.java X Source 1 2 3 4 History ValidatorNumeric.java X mainValidatorA3.java x 88580 // ValidatorString.java import java.util.Scanner; public class ValidatorString implements Validator { 8 9 10 11 12 13 - 14 15 16 17 } 19 20 21 25 } 26 18 30 31 35 36 9=22±2927282232223223322-239327744 private Scanner scanner; private String prompt; private String[] choices; public ValidatorString(String prompt) { this.scanner = new Scanner (source: System.in); this.prompt = prompt; public ValidatorString(String prompt, String... choices) { this.scanner = new Scanner (source: System.in); this.prompt = prompt; this.choices = choices; public String getRequiredString() { String input; do { System.out.print (s: prompt); input = scanner.nextLine().trim(); while (input.isEmpty()); return input; public String getChoiceString() { String input; boolean validChoice; do { System.out.print (s: prompt); input = scanner.nextLine().trim(); validChoice = false; for (String choice: choices) { if (input.equalsIgnoreCase (anotherString: choice)) { validChoice = true; break; } } 40 41 45 } 46 } if (!validChoice) { } System.out.println(x: "Invalid choice. Please try again."); while (!validChoice); return input;
Complete the code and make it run sucessful by fixing errors
//MainValidatorA3
public class MainA3 {
public static void main(String[] args) {
System.out.println("Welcome to the Validation Tester application");
// Int Test
System.out.println("Int Test");
ValidatorNumeric intValidator = new ValidatorNumeric("Enter an integer between -100 and 100: ", -100, 100);
int num = intValidator.getIntWithinRange();
System.out.println("You entered: " + num + "\n");
// Double Test
System.out.println("Double Test");
ValidatorNumeric doubleValidator = new ValidatorNumeric("Enter a double value: ");
double dbl = doubleValidator.getDoubleWithinRange();
System.out.println("You entered: " + dbl + "\n");
// Required String Test
System.out.println("Required String Test:");
ValidatorString stringValidator = new ValidatorString("Enter a required string: ");
String requiredString = stringValidator.getRequiredString();
System.out.println("\nYou entered: " + requiredString + "\n");
// String Choice Test
System.out.println("String Choice Test");
ValidatorString choiceValidator = new ValidatorString("Select one (x/y): ", "x", "y");
String choice = choiceValidator.getChoiceString();
System.out.println("You entered: " + choice);
System.out.println("\nAll Done!");
}
}
//ValidatorNumeric
import java.util.Scanner;
class ValidatorNumeric {
private String prompt;
private int minInt, maxInt;
private double minDouble, maxDouble;
public ValidatorNumeric() {}
public ValidatorNumeric(String prompt, int min, int max) {
this.prompt = prompt;
this.minInt = min;
this.maxInt = max;
}
public ValidatorNumeric(String prompt, double min, double max) {
this.prompt = prompt;
this.minDouble = min;
this.maxDouble = max;
}
public int getInt() {
Scanner scanner = new Scanner(System.in);
System.out.print(prompt);
while (!scanner.hasNextInt()) {
System.out.println("Error! Invalid integer value. Try again.");
System.out.print(prompt);
scanner.next();
}
return scanner.nextInt();
}
public int getIntWithinRange() {
int num;
do {
num = getInt();
if (num < minInt) {
System.out.println("Error! Number must be greater than " + (minInt - 1));
} else if (num > maxInt) {
System.out.println("Error! Number must be less than " + (maxInt + 1));
}
} while (num < minInt || num > maxInt);
return num;
}
public double getDouble() {
Scanner scanner = new Scanner(System.in);
System.out.print(prompt);
while (!scanner.hasNextDouble()) {
System.out.println("Error! Invalid decimal value. Try again.");
System.out.print(prompt);
scanner.next();
}
return scanner.nextDouble();
}
public double getDoubleWithinRange() {
double num;
do {
num = getDouble();
if (num < minDouble) {
System.out.println("Error! Number must be greater than " + minDouble);
} else if (num > maxDouble) {
System.out.println("Error! Number must be less than " + maxDouble);
}
} while (num < minDouble || num > maxDouble);
return num;
}
}
Validator.Java
// Validator.java
import java.util.Scanner;
public interface Validator {
String getRequiredString();
String getChoiceString();
}
![ValidatorString.java X Validator.java X
Source
1
2
3
4
History
ValidatorNumeric.java X
mainValidatorA3.java x
88580
// ValidatorString.java
import java.util.Scanner;
public class ValidatorString implements Validator {
8
9
10
11
12
13
-
14
15
16
17
}
19
20
21
25
}
26
18
30
31
35
36
9=22±2927282232223223322-239327744
private Scanner scanner;
private String prompt;
private String[] choices;
public ValidatorString(String prompt) {
this.scanner = new Scanner (source: System.in);
this.prompt = prompt;
public ValidatorString(String prompt, String... choices) {
this.scanner = new Scanner (source: System.in);
this.prompt = prompt;
this.choices = choices;
public String getRequiredString() {
String input;
do {
System.out.print (s: prompt);
input = scanner.nextLine().trim();
while (input.isEmpty());
return input;
public String getChoiceString() {
String input;
boolean validChoice;
do {
System.out.print (s: prompt);
input = scanner.nextLine().trim();
validChoice = false;
for (String choice: choices) {
if (input.equalsIgnoreCase (anotherString: choice)) {
validChoice = true;
break;
}
}
40
41
45
}
46
}
if (!validChoice) {
}
System.out.println(x: "Invalid choice. Please try again.");
while (!validChoice);
return input;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa136d107-3f41-4dc0-bcbb-0f1a73f02f84%2Fd6362fab-d7cc-4fad-91da-fc8676e1609d%2Fpxsrfue_processed.png&w=3840&q=75)
Unlock instant AI solutions
Tap the button
to generate a solution
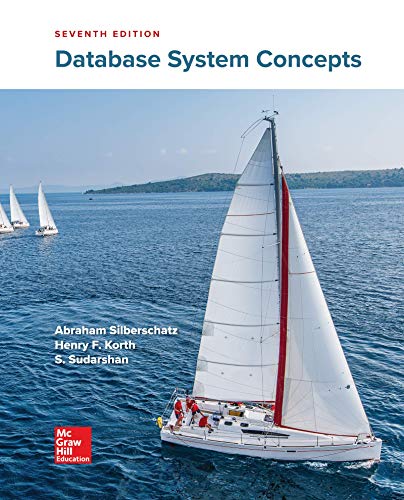
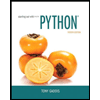
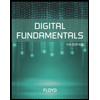
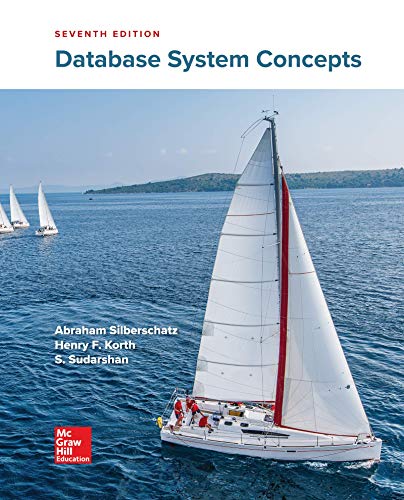
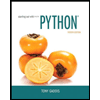
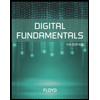
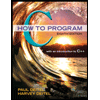
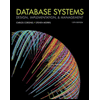
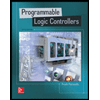