. /Math.java:1: error: class MathUtils is public, should be declared in a file named Mathutils.jav public class MathUtils BankServise.java:153: error: cannot access Math int newCustomers = (int) (Math.random() * 10) + 1; bad source file: ./Math.java file does not contain class Math Please remove or make sure it appears in the correct subdirectory of the sourcepath. 2 errors
How to fix the error?
import java.util.*;
import java.util.Iterator;
import java.util.NoSuchElementException;
class LinkedQueue<Item> implements Iterable<Item> {
private int n;
private Node first;
private Node last;
private class Node {
private Item item;
private Node next;
}
public LinkedQueue() {
first = null;
last = null;
n = 0;
assert check();
}
public boolean isEmpty() {
return first == null;
}
public int size() {
return n;
}
public Item peek() {
if (isEmpty()) throw new NoSuchElementException("Queue underflow");
return first.item;
}
public void enqueue(Item item) {
Node oldlast = last;
last = new Node();
last.item = item;
last.next = null;
if (isEmpty()) first = last;
else oldlast.next = last;
n++;
assert check();
}
public Item dequeue() {
if (isEmpty()) throw new NoSuchElementException("Queue underflow");
Item item = first.item;
first = first.next;
n--;
if (isEmpty()) last = null;
assert check();
return item;
}
public String toString() {
StringBuilder s = new StringBuilder();
for (Item item : this)
s.append(item + " ");
return s.toString();
}
private boolean check() {
if (n < 0) {
return false;
}
else if (n == 0) {
if (first != null) return false;
if (last != null) return false;
}
else if (n == 1) {
if (first == null || last == null) return false;
if (first != last) return false;
if (first.next != null) return false;
}
else {
if (first == null || last == null) return false;
if (first == last) return false;
if (first.next == null) return false;
if (last.next != null) return false;
int numberOfNodes = 0;
for (Node x = first; x != null && numberOfNodes <= n; x = x.next) {
numberOfNodes++;
}
if (numberOfNodes != n) return false;
Node lastNode = first;
while (lastNode.next != null) {
lastNode = lastNode.next;
}
if (last != lastNode) return false;
}
return true;
}
public Iterator<Item> iterator() {
return new LinkedIterator();
}
private class LinkedIterator implements Iterator<Item> {
private Node current = first;
public boolean hasNext() { return current != null; }
public void remove() { throw new UnsupportedOperationException(); }
public Item next() {
if (!hasNext()) throw new NoSuchElementException();
Item item = current.item;
current = current.next;
return item;
}
}
}
class Customer {
private static int custId = 0;
private String name;
public Customer() {
name = "Customer " + custId;
custId++;
}
public String getName() {
return name;
}
public String toString() {
return name;
}
}
public class BankServise{
public static void main(String[] args) {
LinkedQueue<Customer> customerQueue = new LinkedQueue<Customer>();
int counter = 0;
while (counter < 10) {
int newCustomers = (int) (Math.random() * 10) + 1;
for (int i = 0; i < newCustomers; i++) {
Customer c = new Customer();
customerQueue.enqueue(c);
System.out.println(c + " is waiting in line");
}
int servingCustomers = (int) (Math.random() * 10) + 1;
for (int i = 0; i < servingCustomers; i++) {
if (customerQueue.isEmpty()) {
break;
}
System.out.println(customerQueue.dequeue() + " at the teller window");
}
counter++;
}
while (!customerQueue.isEmpty()) {
System.out.println(customerQueue.dequeue() + " is being served");
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

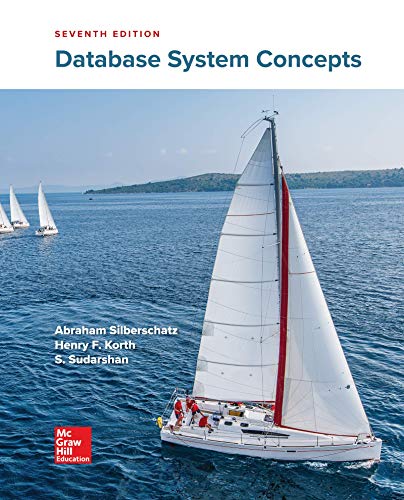
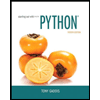
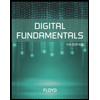
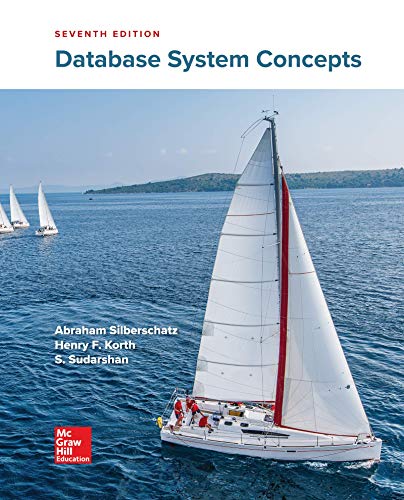
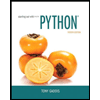
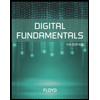
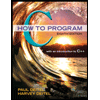
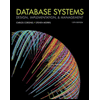
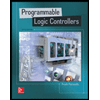