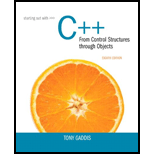
A)
Address of (&) operator:
The main purpose of address operator “&” operator in pointers is to return the memory address of variables.
When the address of operator “&” is placed before the pointer variable, it will hold address of the pointer variable.
Example
//include necessary header
#include <iostream>
#include <string>
using namespace std;
//main method
int main()
{
//variable declaration
int a;
//display the address of the variable a
cout << &a;
}
Representing array using pointer notation:
- In general, array will have a continuous allocation of memory bytes, to represent the values present in the array it can be represented using its subscript value.
- So, to represent the values using pointer notation with an indirection operator the name of the array plus the subscript representing the value needs to be specified.
- When we represent only the array name, it will indicate the first element of the array.
- To represent the next element we need to increment the values by one to find the proceeding elements of the array.
- When “&” operator is used before an array it will return the address of the corresponding value.
B)
Address of (&) operator:
The main purpose of address operator “&” operator in pointers is to return the memory address of variables.
When the address of operator “&” is placed before the pointer variable, it will hold address of the pointer variable.
Example Program:
//include necessary header
#include <iostream>
#include <string>
using namespace std;
//main method
int main()
{
//variable declaration
int a;
//display the address of the variable a
cout << &a;
}
Representing array using pointer notation:
- In general, array will have a continuous allocation of memory bytes, to represent the values present in the array it can be represented using its subscript value.
- So, to represent the values using pointer notation with an indirection operator the name of the array plus the subscript representing the value needs to be specified.
- When we represent only the array name, it will indicate the first element of the array.
- To represent the next element we need to increment the values by one to find the proceeding elements of the array.
- When “&” operator is used before an array it will return the address of the corresponding value.
C)
Address of (&) operator:
The main purpose of address operator “&” operator in pointers is to return the memory address of variables.
When the address of operator “&” is placed before the pointer variable, it will hold address of the pointer variable.
Example Program:
//include necessary header
#include <iostream>
#include <string>
using namespace std;
//main method
int main()
{
//variable declaration
int a;
//display the address of the variable a
cout << &a;
}
Representing array using pointer notation:
- In general, array will have a continuous allocation of memory bytes, to represent the values present in the array it can be represented using its subscript value.
- So, to represent the values using pointer notation with an indirection operator the name of the array plus the subscript representing the value needs to be specified.
- When we represent only the array name, it will indicate the first element of the array.
- To represent the next element we need to increment the values by one to find the proceeding elements of the array.
- When “&” operator is used before an array it will return the address of the corresponding value.
D)
Address of (&) operator:
The main purpose of address operator “&” operator in pointers is to return the memory address of variables.
When the address of operator “&” is placed before the pointer variable, it will hold address of the pointer variable.
Example Program:
//include necessary header
#include <iostream>
#include <string>
using namespace std;
//main method
int main()
{
//variable declaration
int a;
//display the address of the variable a
cout << &a;
}
Representing array using pointer notation:
- In general, array will have a continuous allocation of memory bytes, to represent the values present in the array it can be represented using its subscript value.
- So, to represent the values using pointer notation with an indirection operator the name of the array plus the subscript representing the value needs to be specified.
- When we represent only the array name, it will indicate the first element of the array.
- To represent the next element we need to increment the values by one to find the proceeding elements of the array.
- When “&” operator is used before an array it will return the address of the corresponding value.

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
- Using XML, design a simple user interface for a fictional app. Your UI should include at least three different UI components (e.g., TextView, Button, EditText). Explain the purpose of each component in your design-you need to add screenshots of your work with your name as part of the code to appear on the interface-. Screenshot is needed.arrow_forwardQ4) A thin ring of radius 5 cm is placed on plane z = 1 cm so that its center is at (0,0,1 cm). If the ring carries 50 mA along a^, find H at (0,0,a).arrow_forward4. [15 pts] A logic function F of four variables a; b; c; d is described by the following K-map. Derive the fully minimized SOP logic expression form of F. cd ab 00 01 11 10 00 0 0 0 1 01 1 0 0 1 11 1 0 1 1 10 0 0 1 1arrow_forward
- 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisimarrow_forwardHow would I go about creating this computer database in MariaDB with sql? Create a database name "dbXXXXXX" Select the database using the "use [database name]" command. Now you are in the database. Based on the above schema from Enrolment System database, create all the tables with the last 6 digits of "123456", then the table name for table Lecturer should be "123456_Lecturer". Refer to basic SQL lecture note to create table that has primary keys and Foreign Keys. Provide the datatype of each attributes. Add a column called "Department" with datatype "VARCHAR(12)" to the table "Lecturer". Shows the metadata of the updated "Lecturer" table. (Use Describe command) Drop the "Department" column from the table "Lecturer", and show the metadata of the updated "Lecturer" table. Insert three (3) data to each of the table in the tables created. Note: If you have foreign key issues, please disable foreign key constraints before inserting the data, see below SET FOREIGN_KEY_CHECKS=0;…arrow_forwardCSE330 Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. 3. Consider the real number x = (3.395) 10 (a) (b) Convert the decimal number x into binary format up to 7 binary places (7 binary digits after decimal) Convert the calculated value into denormalized form and calculate fl(x) for m=4 Don't use any Al tool show answer in pen a nd paper then take pi ctures and sendarrow_forward
- Simplify the following expressions by means of a four-variable K-Map. AD+BD+ BC + ABDarrow_forwardCSE330 Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. 2. Let ẞ 2, m = 6, emin = -3 and emax = 3. Answer the following questions: Compute the minimum of |x| for General and Normalized form (a) Compute the Machine Epsilon value for the General and Denormalized form. If we change the value of emax to 6 then how will it affect the value of maximum scale invariant error for the case of Normalized form? Explain your answer. show answer in pen a Don't use any Al tool nd paper then take pi ctures and sendarrow_forwardCSE330: Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. Now, let's take, ẞ = 2, m = 3, emin = -2 and emax = 3. Based on these, answer the following: (a) (b) (c) (d) What are the maximum/largest numbers that can be stored in the system by these three forms defined above? (express your answer in decimal values) What are the non-negative minimum/smallest numbers that can be stored in the system by the denormalized form? (express your answer in decimal values) How many numbers (both non-negative and negative) can be represented in the above mentioned system using the general form? Explain your answer. Find all the decimal numbers for e = 3 and e = 2 in denormalized form, plot them on a real line and prove that all the numbers are not equally spaced. Write the equally spaced sets for the number line you drew. show your answer in Don't use any Al tool pen…arrow_forward
- 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB 0 f(A,B,C,D)arrow_forwardSuppose your computer is responding very slowly to information requests from the Internet. You observe that your network gateway shows high levels of network activity even though you have closed your e-mail client, Web browser, and all other programs that access the Internet. What types of malwares could cause such symptoms? What steps can you take to check whether malware has gained access to your system? What tools can you use at each step? If you identify malware, what ways might it have entered your system? How can you restore your PC to safe operation, including the special software tools you may use?arrow_forwardR languagearrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
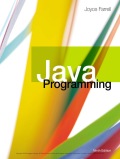
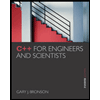
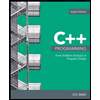
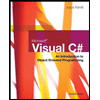
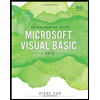