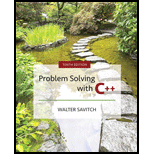
Concept explainers
Pointer in C++:
A pointer is a variable whose value will be another variable’s address. Generally, a pointer variable is declared as follows:
type *var-name;
Here, “type” is the pointer’s base type and “var-name” is the pointer variable name. The asterisk is used to designate a variable as a pointer.
The delete operator:
The delete operator is used to destruct the object that is created with new by deallocating the memory associated with the object.
The syntax of delete operator is as follows:
[] delete cast-expression
[] delete[] cast-expression
In the above statement, the “cast-expression” argument must be a pointer to a block of memory previously allocated for an object created with the new operator.
Given code:
The following code is used to create a dynamic array.
int *entry; //Initialise pointer
entry = new int[10]; //create a dynamic array

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Problem Solving with C++ (10th Edition)
- I need to render an image of a car continuously for a smooth visual experience in C# WinForms. It gets the location array (that has all the x,y of the tiles it should visit) from another function - assume it is already written.arrow_forwardwrite c program with features: Register a Bunny: Store the bunny's name, poem, and initialize the egg count to 0. Modify an Entry: Change the bunny's poem or update the egg count. Delete a Bunny: Remove a registered bunny from the list. List All Bunnies: Display all registered bunnies and their details. Save & Load Data: Store bunny data in a file to persist between runs. Use a struct to represent a bunny contestant. Store data in a binary file (bunnies.dat) for persistence. Use file I/O functions (fopen, fwrite, fread, etc.) to manage data. Implement a menu-driven interface for user interaction.arrow_forwardHelp, how do I write the pseudocode for the findMean function and flowchart for this?arrow_forward
- Need help drawing a flowchart for the findMax function herearrow_forwardNeed help writing the pseudocode for the findMin function with attachedarrow_forwardCreate a static function in C# where poachers appear and attempt to hunt animals. It gets the location of the closest animal to itself. Take account of that the animal also move too, so it should update the closest location (x, y) everytime it moves to a new location. Use winforms to show the movements of poachers.arrow_forward
- Create a static function in C# where poachers appear and attempt to hunt animals. It gets the location of the closest animal to itself. Take account of that the animal also moves too, so it should update the closest location (x, y) everytime it moves to a new location. Use winforms to show to movementsarrow_forwardI have to develop an efficient parallel numerical integration program on a 2-D mesh but I'm struggling. And it has to be in Cstararrow_forwardAn employee is departing from the company you work for. Explain why it could be best practice not to delete their user account but to lock it instead.arrow_forward
- the nagle algorithm, built into most tcp implementations, requires the sender to hold a partial segment's worth of data (even if pushed) until either a full segment accumulates or the most recent outstanding ack arrives. (a) suppose the letters abcdefghi are sent, one per second, over a tcp connection with an rtt of 4.1 seconds. draw a timeline indicating when each packet is sent and what it contains.arrow_forwardJust need some assistance with number 3 please, in C#arrow_forwardHow do we find the possible final values of variable x in the following program. Int x=0; sem s1=1, s2 =0; CO P(s2); P(s1); x=x*2; V(s1); // P(s1); x=x*x; V(s1); // P(s1); x=x+3; V(s2); V(s1); Ocarrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
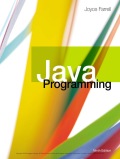
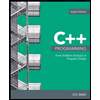
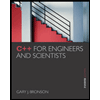
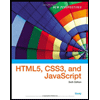
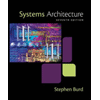