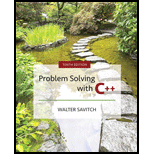
Pointer in C++:
A pointer is a variable whose value will be another variable’s address. Generally, a pointer variable is declared as follows:
type *var-name;
Here, type is the pointer’s base type and var-name is the pointer variable name. The asterisk is used to designate a variable as a pointer.
Given Program:
//Include libraries
#include <iostream>
//Use namespace
using namespace std;
//Define main method
int main()
{
// Declare array
int a[10];
// Declare variable
int *p = a;
// Declare variable
int i;
//Loop executes until the value of i exceeds 10
for (i = 0; i < 10; i++)
//Assign value i to a[i]
a[i] = i;
//Loop executes until the value of i exceeds 10
for (i = 0; i < 10; i++)
//Display value
cout << p[i] << " ";
cout << endl;
//Pause console window
system("pause");
//Return 0 value
return 0;
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Problem Solving with C++ (10th Edition)
- How to develop a C program that receives the message sent by the provided program and displays the name and email included in the message on the screen?Here is the code of the program that sends the message for reference: typedef struct { long tipo; struct { char nome[50]; char email[40]; } dados;} MsgStruct; int main() { int msg_id, status; msg_id = msgget(1000, 0600 | IPC_CREAT); exit_on_error(msg_id, "Creation/Connection"); MsgStruct msg; msg.tipo = 5; strcpy(msg.dados.nome, "Pedro Silva"); strcpy(msg.dados.email, "pedro@sapo.pt"); status = msgsnd(msg_id, &msg, sizeof(msg.dados), 0); exit_on_error(status, "Send"); printf("Message sent!\n");}arrow_forward9. Let L₁=L(ab*aa), L₂=L(a*bba*). Find a regular expression for (L₁ UL2)*L2. 10. Show that the language is not regular. L= {a":n≥1} 11. Show a derivation tree for the string aabbbb with the grammar S→ABλ, A→aB, B→Sb. Give a verbal description of the language generated by this grammar.arrow_forward14. Show that the language L= {wna (w) < Nь (w) < Nc (w)} is not context free.arrow_forward
- 7. What language is accepted by the following generalized transition graph? a+b a+b* a a+b+c a+b 8. Construct a right-linear grammar for the language L ((aaab*ab)*).arrow_forward5. Find an nfa with three states that accepts the language L = {a^ : n≥1} U {b³a* : m≥0, k≥0}. 6. Find a regular expression for L = {vwv: v, wЄ {a, b}*, |v|≤4}.arrow_forward15. The below figure (sequence of moves) shows several stages of the process for a simple initial configuration. 90 a a 90 b a 90 91 b b b b Represent the action of the Turing machine (a) move from one configuration to another, and also (b) represent in the form of arbitrary number of moves.arrow_forward
- 12. Eliminate useless productions from Sa aA BC, AaBλ, B→ Aa, C CCD, D→ ddd Cd. Also, eliminate all unit-productions from the grammar. 13. Construct an npda that accepts the language L = {a"b":n≥0,n‡m}.arrow_forwardYou are given a rope of length n meters and scissors that can cut the rope into any two pieces. For simplification, only consider cutting the rope at an integer position by the meter metric. Each cut has a cost associated with it, c(m), which is the cost of cutting the rope at position m. (You can call c(m) at any time to return the cost value.) The goal is to cut the rope into k smaller pieces, minimizing the total cost of cutting. B Provide the pseudo-code of your dynamic programming algorithm f(n,k) that will return the minimum cost of cutting the rope of length n into k pieces. Briefly explain your algorithm. What is the benefit of using dynamic programming for this problem? What are the key principles of dynamic programming used in your algorithm?arrow_forwardDetermine whether each of the problems below is NP-Complete or P A. 3-SAT B. Traveling Salesman Problem C. Minimum Spanning Tree D. Checking if a positive integer is prime or not. E. Given a set of linear inequalities with integer variables, finding a set of values for the variables that satisfies all inequalities and maximizes or minimizes a given linear objective function.arrow_forward
- 1. Based on our lecture on NP-Complete, can an NP-Complete problem not have a polynomial-time algorithm? Explain your answer. 2. Prove the conjecture that if any problem in NP is not polynomial-time solvable, then no NP-Complete problem is polynomial-time solvable. (You can't use Theorem 1 and 2 directly) 3. After you complete your proof in b), discuss how this conjecture can be used to solve the problem of whether P=NP.arrow_forwardBased on our lectures and the BELLMAN-FORD algorithm below, answer the following questions. BELLMAN-FORD (G, w, s) 1 INITIALIZE-SINGLE-SOURCE (G, s) 2 for i = 1 to |G. VI - 1 3 4 5 6 7 8 for each edge (u, v) = G.E RELAX(u, v, w) for each edge (u, v) = G.E if v.d> u.d+w(u, v) return FALSE return TRUE 1. What does the algorithm return? 2. Analyze the complexity of the algorithm.arrow_forward(Short-answer) b. Continue from the previous question. Suppose part of the data you extracted from the data warehouse is the following. Identify the missing values you think exist in the dataset. Use Column letter and Row number to refer to each missing value in the dataset. Please write down how you want to address each particular missing value (you can group them if they receive same treatment). For imputation, you do not need to calculate the exact imputed values but just describe what kind of value you want to use to impute.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
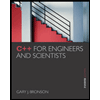
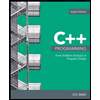