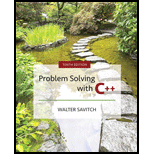
Pointer in C++:
A pointer is a variable whose value will be another variable’s address. Generally, a pointer variable is declared as follows:
type *var-name;
Here, type is the pointer’s base type and var-name is the pointer variable name. The asterisk is used to designate a variable as a pointer.
Given Program:
//Include libraries
#include <iostream>
//Use namespace
using namespace std;
//Define main method
int main()
{
// Declare pointer variables
int *p1, *p2;
//Assign value
p1 = new int;
//Assign value
p2 = new int;
//Assign value
*p1 = 10;
//Assign value
*p2 = 20;
//Display value
cout << *p1 << " " << *p2 << endl;
//Assign value
p1 = p2;
//Display value
cout << *p1 << " " << *p2 << endl;
//Assign value
*p1 = 30;
//Display value
cout << *p1 << " " << *p2 << endl;
//Pause console window
system("pause");
//Return 0 value
return 0;
}
Pointer in C++:
A pointer is a variable whose value will be another variable’s address. Generally, a pointer variable is declared as follows:
type *var-name;
Here, type is the pointer’s base type and var-name is the pointer variable name. The asterisk is used to designate a variable as a pointer.
Given Program:
//Include libraries
#include <iostream>
//Use namespace
using namespace std;
//Define main method
int main()
{
// Declare pointer variables
int *p1, *p2;
//Assign value
p1 = new int;
//Assign value
p2 = new int;
//Assign value
*p1 = 10;
//Assign value
*p2 = 20;
//Display value
cout << *p1 << " " << *p2 << endl;
//Assign value
p1 = p2;
//Display value
cout << *p1 << " " << *p2 << endl;
//Assign value
*p2 = 30;
//Display value
cout << *p1 << " " << *p2 << endl;
//Pause console window
system("pause");
//Return 0 value
return 0;
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Problem Solving with C++ (10th Edition)
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
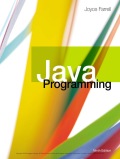
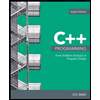
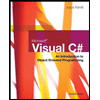
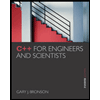
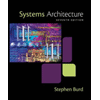