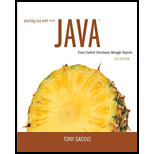
Starting Out with Java: From Control Structures through Objects (6th Edition)
6th Edition
ISBN: 9780133957051
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 8, Problem 8PC
Parking Ticket Simulator
For this assignment you will design a set of classes that work together to simulate a police officer issuing a parking ticket. You should design the following classes:
- The ParkedCar Class: This class should simulate a parked car. The class’s responsibilities are as follows:
- To know the car’s make, model, color, license number, and the number of minutes that the car has been parked.
- The ParkingMeter Class: This class should simulate a parking meter. The class’s only responsibility is as follows:
- To know the number of minutes of parking time that has been purchased.
- The ParkingTicket Class: This class should simulate a parking ticker. The class’s responsibilities are as follows:
- To report the make, model, color, and license number of the illegally parked car
- To report the amount of the fine, which is $25 for the first hour or part of an hour that the car is illegally parked, plus $10 for every additional hour or part of an hour that the car is illegally parked
- To report the name and badge number of the police officer issuing the ticket
- The PoliceOfficer Class: This class should simulate a police officer inspecting parked cars. The class’s responsibilities are as follows:
- To know the police officer’s name and badge number
- To examine a ParkedCar object and a ParkingMeter object, and determine whether the car’s time has expired
- To issue a parking ticket (generate a ParkingTicket object) if the car’s time has expired
Write a program that demonstrates how these classes collaborate.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
3. Problem Description: Define the Circle2D class that contains:
Two double data fields named x and y that specify the center of the circle with get
methods.
• A data field radius with a get method.
•
A no-arg constructor that creates a default circle with (0, 0) for (x, y) and 1 for radius.
•
A constructor that creates a circle with the specified x, y, and radius.
•
A method getArea() that returns the area of the circle.
•
A method getPerimeter() that returns the perimeter of the circle.
•
•
•
A method contains(double x, double y) that returns true if the specified point (x, y) is inside
this circle. See Figure (a).
A method contains(Circle2D circle) that returns true if the specified circle is inside this
circle. See Figure (b).
A method overlaps (Circle2D circle) that returns true if the specified circle overlaps with this
circle. See the figure below.
р
O
со
(a)
(b)
(c)<
Figure (a) A point is inside the circle. (b) A circle is inside another circle.
(c) A circle overlaps another…
1. Explain in detail with examples each of the following fundamental security design principles: economy of mechanism, fail-safe default, complete mediation, open design, separation of privilege, least privilege, least common mechanism, psychological acceptability, isolation, encapsulation, modularity, layering, and least astonishment.
Security in general means the protection of an asset. In the context of computer and network security, explore and explain what assets must be protected within an online university. What the threats are to the security of these assets, and what countermeasures are available to mitigate and protect the organization from such threats. For each of the assets you identify, assign an impact level (low, moderate, or high) for the loss of confidentiality, availability, and integrity. Justify your answers.
Chapter 8 Solutions
Starting Out with Java: From Control Structures through Objects (6th Edition)
Ch. 8.1 - What is the difference between an instance field...Ch. 8.1 - Prob. 8.2CPCh. 8.1 - Describe the limitation of static methods.Ch. 8.8 - Prob. 8.4CPCh. 8.9 - Look at the following statement, which declares an...Ch. 8.9 - Assume that the following enumerated data type has...Ch. 8.9 - Prob. 8.7CPCh. 8 - This type of method cannot access any non-static...Ch. 8 - Prob. 2MCCh. 8 - If you write this method for a class, Java will...
Ch. 8 - Making an instance of one class a field in another...Ch. 8 - This is the name of a reference variable that is...Ch. 8 - This enum method returns the position of an enum...Ch. 8 - Assuming the following declaration exists: enum...Ch. 8 - You cannot use the fully qualified name of an enum...Ch. 8 - The Java Virtual Machine periodically performs...Ch. 8 - If a class has this method, it is called...Ch. 8 - CRC stands for a. Class, Return value, Composition...Ch. 8 - True or False: A static member method may refer to...Ch. 8 - True or False: All static member variables are...Ch. 8 - Prob. 14TFCh. 8 - Prob. 15TFCh. 8 - Prob. 16TFCh. 8 - True or False: Enumerated data types are actually...Ch. 8 - True or False: enum constants have a toString...Ch. 8 - public class MyClass { private int x; private...Ch. 8 - Assume the following declaration exists : enum...Ch. 8 - Consider the following class declaration: public...Ch. 8 - Consider the following class declaration: public...Ch. 8 - A pet store sells dogs, cats, birds, and hamsters....Ch. 8 - Prob. 1SACh. 8 - Prob. 2SACh. 8 - Prob. 3SACh. 8 - Even if you do not write an equals method for a...Ch. 8 - A has a relationship can exist between classes....Ch. 8 - Prob. 6SACh. 8 - Is it advisable or not advisable to write a method...Ch. 8 - Prob. 8SACh. 8 - Look at the following declaration: enum Color {...Ch. 8 - Assuming the following enum declaration exists:...Ch. 8 - Under what circumstances does an object become a...Ch. 8 - Area Class Write a class that has three overloaded...Ch. 8 - BankAccount Class Copy Constructor Add a copy...Ch. 8 - Carpet Calculator The Westfield Carpet Company has...Ch. 8 - LandTract Class Make a LandTract class that has...Ch. 8 - Month Class Write a class named Month. The class...Ch. 8 - CashRegister Class Write a CashRegister class that...Ch. 8 - Sales Receipt File Modify the program you wrote in...Ch. 8 - Parking Ticket Simulator For this assignment you...Ch. 8 - Geometry Calculator Design a Geometry class with...Ch. 8 - Car Instrument Simulator For this assignment, you...Ch. 8 - First to One Game This game is meant for two or...Ch. 8 - Heads or TaiLs Game This game is meant for two or...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Describe some of the ways that structural adhesives might deteriorate over time.
Degarmo's Materials And Processes In Manufacturing
In Exercises 41 through 46, identify the errors.
Introduction To Programming Using Visual Basic (11th Edition)
List the five major hardware components of a computer system.
Starting Out With Visual Basic (8th Edition)
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
Use the following tables for your answers to questions 3.7 through 3.51 : PET_OWNER (OwnerID, OwnerLasst Name, ...
Database Concepts (8th Edition)
Find the no-load value of υo in the circuit shown.
Find υo when RL is 150 Ω.
How much power is dissipated in th...
Electric Circuits. (11th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please include comments and docs comments on the program. The two other classes are Attraction and Entertainment.arrow_forwardObject-Oriented Programming In this separate files. ent, you'll need to build and run a small Zoo in Lennoxville. All classes must be created in Animal (5) First, start by building a class that describes an Animal at a Zoo. It should have one private instance variable for the name of the animal, and one for its hunger status (fed or hungry). Add methods for setting and getting the hunger satus variable, along with a getter for the name. Consider how these should be named for code clarity. For instance, using a method called hungry () to make the animal hungry could be used as a setter for the hunger field. The same logic could be applied to when it's being fed: public void feed () { this.fed = true; Furthermore, the getter for the fed variable could be named is Fed as it is more descriptive about what it answers when compared to get Fed. Keep this technique in mind for future class designs. Zoo (10) Now we have the animals designed and ready for building a little Zoo! Build a class…arrow_forward1.[30 pts] Answer the following questions: a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 0 1 1 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report. 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisim in your report. 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB C…arrow_forward
- When using functions in python, it allows us tto create procedural abstractioons in our programs. What are 5 major benefits of using a procedural abstraction in python?arrow_forwardFind the error, assume data is a string and all variables have been declared. for ch in data: if ch.isupper: num_upper = num_upper + 1 if ch.islower: num_lower = num_lower + 1 if ch.isdigit: num_digits = num_digits + 1 if ch.isspace: num_space = num_space + 1arrow_forwardFind the Error: date_string = input('Enter a date in the format mm/dd/yyyy: ') date_list = date_string.split('-') month_num = int(date_list[0]) day = date_list[1] year = date_list[2] month_name = month_list[month_num - 1] long_date = month_name + ' ' + day + ', ' + year print(long_date)arrow_forward
- Find the Error: full_name = input ('Enter your full name: ') name = split(full_name) for string in name: print(string[0].upper(), sep='', end='') print('.', sep=' ', end='')arrow_forwardPlease show the code for the Tikz figure of the complex plane and the curve C. Also, mark all singularities of the integrand.arrow_forward11. Go to the Webinars worksheet. DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: a. Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. b. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. c. Determine and enter the constraints based on the information provided in Table 3. d. Use Simplex LP as the solving method to find a global optimal solution. e. Save the Solver model below the Maximum weekly profit model label. f. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and…arrow_forward
- Go to the Webinars DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. Determine and enter the constraints based on the information provided in Table 3. Use Simplex LP as the solving method to find a global optimal solution. Save the Solver model below the Maximum weekly profit model label. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and Thursday…arrow_forwardI want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)? When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene. Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency. To elaborate,…arrow_forwardI want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.To elaborate, what…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
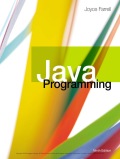
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
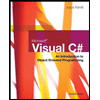
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
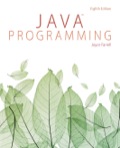
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
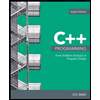
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
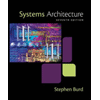
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY