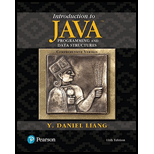
(Algebra: add two matrices) Write a method to add two matrices. The header of the method is as follows:
public static double [][] addMatrix(double[] [] a , double[][] b)
In order to be added, the two matrices must have the same dimensions and the same or compatible types of elements. Let c be the resulting matrix. Each element cij is aij + bij. For example, for two 3 × 3 matrices a and b, c is
Write a test program that prompts the user to enter two 3 × 3 matrices and displays their sum. Here is a sample run:

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Digital Fundamentals (11th Edition)
Starting Out with Python (3rd Edition)
Concepts of Programming Languages (11th Edition)
C How to Program (8th Edition)
- (Java in Eclipse) Write a method that returns the sum of all the elements in a specified column in a 2-D array using the following header: public static int sumCol(int[][] m, int colIdx) Included is a test class and a sample runarrow_forward(See attached photo) Please explain the exact running time of the program. The attached photo's answer is already correct only the explanation of getting the exact running time is needed.arrow_forward(Find the index of the largest element) Write a function that returns the index of the largest element in an array of integers. If there are more such elements than one, return the largest index. Use the following header: int indexOfLargestElement(double array[], int size)arrow_forward
- (Sort ArrayList) Write the following method that sorts an ArrayList: public static <E extends Comparable<E>> void sort(ArrayList<E> list) Write a test program that prompts the user to enter 10 integers, invokes this method to sort the numbers, and displays the numbers in increasing order. Sample Run Enter 10 integers: 3 4 12 7 3 4 5 6 4 7 The sorted numbers are 3 3 4 4 4 5 6 7 7 12 Class Name: Exercise19_09arrow_forward(Java) Q4 explain the answers to the below questions using step-by-step explanation. 4. Write the bubbleSort method for an array of integers The name of the method is bubbleSort It takes in one parameter - an array of integers It sorts the array according to the bubble sort algorithm It returns nothingarrow_forward(Compute total marks for each student) Suppose the marks obtained by all students are stored ina two-dimensional array. Each row records a student’s marks for five tests. For example, the followingarray stores the test marks for eight students. Write a program that displays students and the totalmarks they obtained in five tests, in decreasing order of the total marks.arrow_forward
- Method Details: public static void rotate(int[] array, boolean leftRotation, int positions) Rotates the provided array left if leftRotation is true; right otherwise. The number of positions to rotate is determined by positions. For example, rotating the array 10, 20, 7, 8 two positions to the left will update the array to 7, 8, 10, 20. Only arrays with 2 or more elements will be rotated. Hint: adding private methods that rotate an array one position to the left and one position to the right can help. Parameters: array - leftRotation - positions - Throws:java.lang.IllegalArgumentException - When a null array parameter is providedarrow_forward(Algebra: perfect square ) Write a program that prompts the user to enter an integer m and find the smallest integer n such that m * n is a perfect square. (Hint: Store all smallest factors of m into an array list. n is the product of the factors that appear an odd number of times in the array list. For example, consider m = 90, store the factors 2, 3, 3, 5 in an array list. 2 and 5 appear an odd number of times in the array list. So, n is 10.) Sample Run 1 Enter an integer m: 1500 The smallest number n for m x n to be a perfect square is 15 m x n is 22500 Sample Run 2 Enter an integer m: 63 The smallest number n for m x n to be a perfect square is 7 m x n is 441 Class Name: Exercise11_17 Answer is : import java.util.Scanner; public class Squares { public static void main(String[] args) { Scanner scan = new Scanner(System.in); //instantiation of Scanner that will take inputs from the keyboard //the try catch block below is for trapping error with the input try {…arrow_forwardWrite a code that will doarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
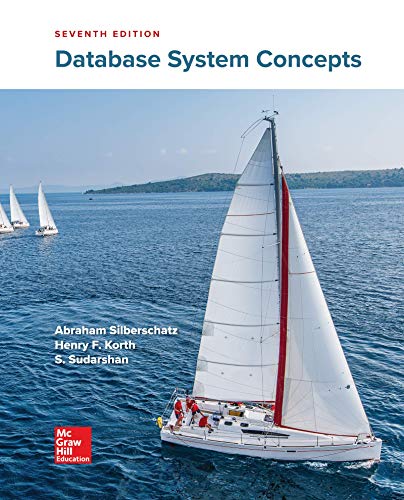
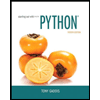
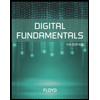
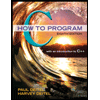
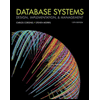
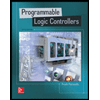