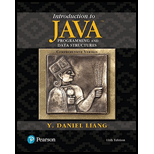
(Largest block) Given a square matrix with the elements 0 or l, write a
Your program should implement and use the following method to find the maximum square submatrix:
public static int[] findLargestBlock(int[] [] m)
The return value is an array that consists of three values. The first two values are the row and column indices for the first element in the submatrix, and the third value is the number of the rows in the submatrix.

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Starting Out with Python (4th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Starting Out With Visual Basic (8th Edition)
- I need help in explaining how I can demonstrate how the Laplace & Inverse transformations behaves in MATLAB transformation (ex: LIke in graph or something else)arrow_forwardYou have made the Web solution with Node.js. please let me know what problems and benefits I would experience while making the Web solution here, as compared to any other Web solution you have developed in the past. what problems and benefits/things to keep in mind as someone just learningarrow_forwardPHP is the server-side scripting language. MySQL is used with PHP to store all the data. EXPLAIN in details how to install and run the PHP/MySQL on your computer. List the issues and challenges I may encounter while making this set-up? why I asked: I currently have issues logging into http://localhost/phpmyadmin/ and I tried using the command prompt in administrator to reset the password but I got the error LOCALHOST PORT not found.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
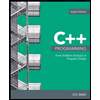
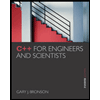
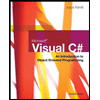
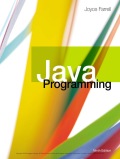
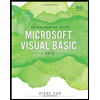