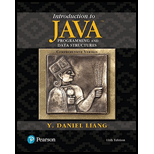
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 8, Problem 8.26PE
(Row sorting) Implement the following method to sort the rows in a two-dimensional array. A new array is returned and the original array is intact.
public static double[][] sortRows(double[][] m)
Write a test
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
2:21 m
Ο
21%
AlmaNet
WE ARE
HIRING
Experienced Freshers
Salesforce
Platform
Developer
APPLY NOW
SEND YOUR CV:
Email: hr.almanet@gmail.com
Contact: +91 6264643660
Visit: www.almanet.in
Locations: India, USA, UK, Vietnam
(Remote & Hybrid Options Available)
Provide a detailed explanation of the architecture on the diagram
hello please explain the architecture in the diagram below. thanks you
Chapter 8 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 8.2 - Declare an array reference variable for a...Ch. 8.2 - Prob. 8.2.2CPCh. 8.2 - What is the output of the following code? int[] []...Ch. 8.2 - Which of the following statements are valid? int...Ch. 8.3 - Show the output of the following code: int[][]...Ch. 8.3 - Show the output of the following code: int[][]...Ch. 8.4 - Show the output of the following code: public...Ch. 8.5 - Prob. 8.5.1CPCh. 8.6 - What happens if the input has only one point?Ch. 8.7 - What happens if the code in line 51 in Listing 8.4...
Ch. 8.8 - Declare an array variable for a three-dimensional...Ch. 8.8 - Assume char[][][] x =new char[12][5][2], how many...Ch. 8.8 - Show the output of the following code: int[][][]...Ch. 8 - (Sum elements column by column) Write a method...Ch. 8 - (Sum the major diagonal in a matrix) Write a...Ch. 8 - (Sort students on grades) Rewrite Listing 8.2,...Ch. 8 - (Compute the weekly hours for each employee)...Ch. 8 - (Algebra: add two matrices) Write a method to add...Ch. 8 - (Algebra: multiply two matrices) Write a method to...Ch. 8 - (Points nearest to each other) Listing 8.3 gives a...Ch. 8 - (All closest pairs of points) Revise Listing 8.3,...Ch. 8 - Prob. 8.9PECh. 8 - (Largest row and column) Write a program that...Ch. 8 - (Game: nine heads and tails) Nine coins are placed...Ch. 8 - (Financial application: compute tax) Rewrite...Ch. 8 - (Locate the largest element) Write the following...Ch. 8 - (Explore matrix) Write a program that prompts the...Ch. 8 - (Geometry: same line ?) Programming Exercise 6.39...Ch. 8 - (Sort two-dimensional array) Write a method to...Ch. 8 - (Financial tsunami) Banks lend money to each...Ch. 8 - (Shuffle rows) Write a method that shuffles the...Ch. 8 - (Pattern recognition: four consecutive equal...Ch. 8 - Prob. 8.20PECh. 8 - (Central city) Given a set of cities, the central...Ch. 8 - (Even number of 1s) Write a program that generates...Ch. 8 - (Game: find the flipped cell) Suppose you are...Ch. 8 - (Check Sudoku solution) Listing 8.4 checks whether...Ch. 8 - Prob. 8.25PECh. 8 - (Row sorting) Implement the following method to...Ch. 8 - (Column sorting) Implement the following method to...Ch. 8 - (Strictly identical arrays) The two-dimensional...Ch. 8 - (Identical arrays) The two-dimensional arrays m1...Ch. 8 - (Algebra: solve linear equations) Write a method...Ch. 8 - (Geometry: intersecting point) Write a method that...Ch. 8 - (Geometry: area of a triangle) Write a method that...Ch. 8 - (Geometry: polygon subareas) A convex four-vertex...Ch. 8 - (Geometry: rightmost lowest point) In...Ch. 8 - (Largest block) Given a square matrix with the...Ch. 8 - (Latin square) A Latin square is an n-by-n array...Ch. 8 - (Guess the capitals) Write a program that...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
The current source in the circuit shown generates the current pulse
Find (a) v (0); (b) the instant of time gr...
Electric Circuits. (11th Edition)
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
In Exercises 39 through 44, write a program to carry out the stated task. Cost of Electricity The cost of the e...
Introduction To Programming Using Visual Basic (11th Edition)
What is an object?
Starting Out With Visual Basic (8th Edition)
Fill in the blanks in each of the following statements: A relation that has no partial functional dependencies ...
Modern Database Management
Translate the following instructions from English into Vole. a. LOAD register 0x6 with the value 0x77. b. LOAD ...
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
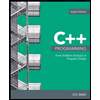
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
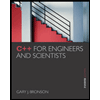
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
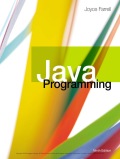
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
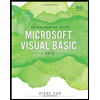
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
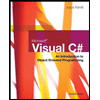
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
1.1 Arrays in Data Structure | Declaration, Initialization, Memory representation; Author: Jenny's lectures CS/IT NET&JRF;https://www.youtube.com/watch?v=AT14lCXuMKI;License: Standard YouTube License, CC-BY
Definition of Array; Author: Neso Academy;https://www.youtube.com/watch?v=55l-aZ7_F24;License: Standard Youtube License