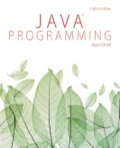
a.
Explanation of Solution
Program:
File name: “Die.java”
//Define a class named Die
public class Die
{
//Declare the private variables and initialize the value
private int value;
private static final int HIGHEST_DIE_VALUE = 6;
private static final int LOWEST_DIE_VALUE = 1;
//Define a Die method
public Die()
{
//Calculate the value
value = ((int)(Math.random() * 100) % HIGHEST_DIE_VALUE +
LOWEST_DIE_VALUE);
}
//Define a getValue method
public int getValue()
{
//Return the value
return value;
}
}
File name: “FiveDice2.java”
//Define a class named FiveDice2
public class FiveDice2
{
//Define a main method
public static void main(String[] args)
{
//Declare a variable and initialize the value
final int NUM = 5;
//Store the five dice rolled value for computer
Die[] comp = new Die[NUM];
//Store the five dice rolled value for player
Die[] player = new Die[NUM];
//Declare a variable
int x;
//For loop to be executed until x exceeds NUM
for(x = 0; x < NUM; ++x)
{
//Allocating memory for the array objects
comp[x] = new Die();
player[x] = new Die();
}
//Declare the variables
int compMatch, playerMatch;
//Get computer name
String computerName = "Computer";
//Get player name
String playerName = "You";
//Display the message
display(computerName, comp, NUM);
display(playerName, player, NUM);
//Calls the method to find same numbers for computer
compMatch = howManySame(comp, NUM);
//Calls the method to find same numbers for player
playerMatch = howManySame(player, NUM);
//If computer has one of a kind
if(compMatch == 1)
//Print the result
System.out.println("Computer has nothing");
//Else computer has a pair, three, four, or five of a //kind
else
//Print the result
System.out.println("Computer has " + compMatch + " of a kind");
//If player has one of a kind
if(playerMatch == 1)
//Print the result
System.out.println("You have nothing");
//Else player has a pair, three, four, or five of a kind
else
//Print the result
System.out.println("You have " + playerMatch + " of a kind");
//If any higher combination of computer beats a lower //one of player
if(compMatch > playerMatch)
//Print the result
System...
b.
Explanation of Solution
Program:
File name: “Die.java”
//Define a class named Die
public class Die
{
//Declare the private variables and initialize the value
private int value;
private static final int HIGHEST_DIE_VALUE = 6;
private static final int LOWEST_DIE_VALUE = 1;
//Define a Die method
public Die()
{
//Calculate the value
value = ((int)(Math.random() * 100) % HIGHEST_DIE_VALUE +
LOWEST_DIE_VALUE);
}
//Define a getValue method
public int getValue()
{
//Return the value
return value;
}
}
File name: “FiveDice3.java”
//Define a class named FiveDice3
public class FiveDice3
{
//Define a main method
public static void main(String[] args)
{
//Declare a variable and initialize the value
final int NUM = 5;
//Store the five dice rolled value for computer
Die[] comp = new Die[NUM];
//Store the five dice rolled value for player
Die[] player = new Die[NUM];
//Declare a variable
int x;
//For loop to be executed until x exceeds NUM
for(x = 0; x < NUM; ++x)
{
//Allocating memory for the array objects
comp[x] = new Die();
player[x] = new Die();
}
//Declare the variables
int compMatch, playerMatch;
int compHigh, playerHigh;
//Get computer name
String computerName = "Computer";
//Get player name
String playerName = "You";
//Display the message
display(computerName, comp, NUM);
display(playerName, player, NUM);
//Calls the method to find same numbers for computer
compMatch = howManySame(comp, NUM);
//Calls the method to find same numbers for player
playerMatch = howManySame(player, NUM);
//Compute the value
compHigh = compMatch / 10;
playerHigh = playerMatch / 10;
compMatch = compMatch % 10;
playerMatch = playerMatch % 10;
//If computer has one of a kind
if(compMatch == 1)
//Print the result
System.out.println("Computer has nothing");
//Else computer has a pair, three, four, or five of a //kind
else
//Print the result
System.out.println("Computer has " + compMatch + " of a kind");
//If player has one of a kind
if(playerMatch == 1)
//Print the result
System.out.println("You have nothing");
//Else player has a pair, three, four, or five of a kind
else
//Print the result
System.out.println("You have " + playerMatch + " of a kind");
//If any higher combination of computer beats a lower one of player
if(compMatch > playerMatch)
//Print the result
System.out.println("Computer wins");
//Else
else
//If any higher combination of player beats
//a lower one of computer
if(compMatch < playerMatch)
//Print the result
System.out.println("You win");
//Else
else
{
//If values of computer are greater than player's value
if(compHigh > playerHigh)
//Print the result
System...

Trending nowThis is a popular solution!

- EX:[AE00]=fa50h number of ones =1111 1010 0101 0000 Physical address=4AE00h=4000h*10h+AE00h Mov ax,4000 Mov ds,ax; DS=4000h mov ds,4000 X Mov ax,[AE00] ; ax=[ae00]=FA50h Mov cx,10; 16 bit in decimal Mov bl,0 *: Ror ax,1 Jnc ** Inc bl **:Dec cx Jnz * ;LSB⇒CF Cf=1 ; it jump when CF=0, will not jump when CF=1 HW1: rewrite the above example use another wayarrow_forwardEX2: Write a piece of assembly code that can count the number of ones in word stored at 4AE00harrow_forwardWrite a program that simulates a Magic 8 Ball, which is a fortune-telling toy that displays a random response to a yes or no question. In the student sample programs for this book, you will find a text file named 8_ball_responses.txt. The file contains 12 responses, such as “I don’t think so”, “Yes, of course!”, “I’m not sure”, and so forth. The program should read the responses from the file into a list. It should prompt the user to ask a question, then display one of the responses, randomly selected from the list. The program should repeat until the user is ready to quit. Contents of 8_ball_responses.txt: Yes, of course! Without a doubt, yes. You can count on it. For sure! Ask me later. I'm not sure. I can't tell you right now. I'll tell you after my nap. No way! I don't think so. Without a doubt, no. The answer is clearly NO. (You can access the Computer Science Portal at www.pearsonhighered.com/gaddis.)arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
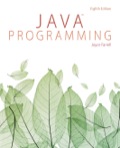
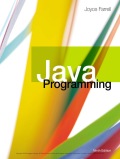
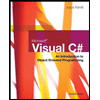
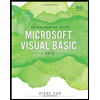
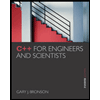