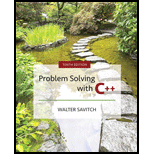
Create a C-string variable that contains a name, age, and title. Each field is separated by a space. For example, the string might contain “Bob 45 Programmer” or any other name/age/title in the same format. Assume the name, age, and title have no spaces themselves. Write a

Program plan:
- Include the necessary header files.
- Declare the namespace.
- Define the “main()” function.
- Declare the necessary variables.
- Initialize the character array.
- Use the functions “strtok()” to split the string into tokens.
- Use the function “strcpy()” to copy the source string into the destination string.
- Print the result.
Program to display name, age and title into separate variables using the function “cstring”.
Explanation of Solution
Program:
//Include the header file iostream
#include<iostream>
//Include the header file cstring
#include<cstring>
//Declare the namespace
using namespace std;
//Define the main() function
int main()
{
//Declare the necessary variables
char name[20];
char age[4];
char title[50];
//Initialize the char array
char res[] = "Bob 45 Programmer";
/*Call the function strtok() to split str into tokens and assign the result in *pch*/
char *pch = strtok(res," ");
/*Call the function strcpy to copy the value in pch into name*/
strcpy(name,pch);
/*Call the function strtok() to split str into tokens and assign the result in *pch*/
pch = strtok(NULL," ");
/*Call the function strcpy to copy the value in pch into age*/
strcpy(age,pch);
/*Call the function strtok() to split str into tokens and assign the result in *pch*/
pch = strtok(NULL," ");
/*Call the function strcpy to copy the value in pch into title*/
strcpy(title,pch);
//print the name
cout<<"Name: "<<name<<" ";
//print the age
cout<<"Age: "<<age<<" ";
//Print the title
cout<<"Title: "<<title;
//Return zero
return 0;
}
Output:
Name: Bob Age: 45 Title: Programmer
Want to see more full solutions like this?
Chapter 8 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Concepts Of Programming Languages
Starting Out With Visual Basic (8th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
- It is possible to sort an array of n values using pipeline of n filter processes.The first process inputs all the values one at a time, keep the minimum, and passes the others on to the next process. Each filter does the same thing; it receives a stream of values from the previous process, keep the smallest, and passes the others to the next process. Assume each process has local storage for only two values--- the next input value and the minimum it has seen so far. (a) Developcode for filter processes. Declare the channels and use asynchronous message passing. Hint:Define an array of channels value[n] (int), and a set of filter processes Filter[i = 0 ton-1]. Each process Filter[i] (where 0 <= i <= n-2) receives a stream of integers through channelvalue[i], keeps the smallest, and sends all other integers to channel value[i+1]. The last processFilter[n-1] receives only one integer through channel value[n-1] and does not need to send anyinteger further.arrow_forwardIt is possible to sort an array of n values using pipeline of n filter processes.The first process inputs all the values one at a time, keep the minimum, and passes the others on to the next process. Each filter does the same thing; it receives a stream of values from the previous process, keep the smallest, and passes the others to the next process. Assume each process has local storage for only two values--- the next input value and the minimum it has seen so far. (a) Developcode for filter processes. Declare the channels and use asynchronous message passing. Hint:Define an array of channels value[n] (int), and a set of filter processes Filter[i = 0 ton-1]. Each process Filter[i] (where 0 <= i <= n-2) receives a stream of integers through channelvalue[i], keeps the smallest, and sends all other integers to channel value[i+1]. The last processFilter[n-1] receives only one integer through channel value[n-1] and does not need to send anyinteger further.arrow_forwardIt is possible to sort an array of n values using pipeline of n filter processes.The first process inputs all the values one at a time, keep the minimum, and passes the others on to the next process. Each filter does the same thing; it receives a stream of values from the previous process, keep the smallest, and passes the others to the next process. Assume each process has local storage for only two values--- the next input value and the minimum it has seen so far. (a) Developcode for filter processes. Declare the channels and use asynchronous message passing. Hint:Define an array of channels value[n] (int), and a set of filter processes Filter[i = 0 ton-1]. Each process Filter[i] (where 0 <= i <= n-2) receives a stream of integers through channelvalue[i], keeps the smallest, and sends all other integers to channel value[i+1]. The last processFilter[n-1] receives only one integer through channel value[n-1] and does not need to send anyinteger further.arrow_forward
- It is possible to sort an array of n values using pipeline of n filter processes.The first process inputs all the values one at a time, keep the minimum, and passes the others on to the next process. Each filter does the same thing; it receives a stream of values from the previous process, keep the smallest, and passes the others to the next process. Assume each process has local storage for only two values--- the next input value and the minimum it has seen so far. (a) Developcode for filter processes. Declare the channels and use asynchronous message passing. Hint:Define an array of channels value[n] (int), and a set of filter processes Filter[i = 0 ton-1]. Each process Filter[i] (where 0 <= i <= n-2) receives a stream of integers through channelvalue[i], keeps the smallest, and sends all other integers to channel value[i+1]. The last processFilter[n-1] receives only one integer through channel value[n-1] and does not need to send anyinteger further.arrow_forwardI need help: Challenge: Assume that the assigned network addresses are correct. Can you deduce (guess) what the network subnet masks are? Explain while providing subnet mask bits for each subnet mask. [Hint: Look at the addresses in binary and consider the host ids]arrow_forwardI would like to know if my answer statment is correct? My answer: The main difference is how routes are created and maintained across different networks. Static routing establishes router connections to different networks from the far left and far right. The Dynamic routing focus emphasizes immediate connection within the router while ignoring the other connections from different networks. Furthermore, the static routing uses the subnet mask to define networks such as 25.0.0.0/8, 129.60.0.0/16, and 200.100.10.0/30, which correspond to 255.0.0.0, 255.255.0.0, and 255.255.255.252. On the other hand, dynamic routing uses the wildcard mask to inverse the subnet mask, where network bits become 0 and host bits become 1, giving us 0.0.0.255, 0.0.255.255, and 0.0.0.3. Most importantly, the CLI commands used for Static and Dynamic routing are also different. For static routing, the “IP route” corresponds with the network, subnet mask, and next-hop IP address. In contrast, dynamic routing uses…arrow_forward
- 1. [30 pts] Match the items on the left with the memory segment in which they are stored. Answers may be used more than once, and more than one answer may be required. 1. Static variables 2. Local variables 3. Global variables 4. Constants 5. Machine Instructions 6. malloc() 7. String Literals Answer A. Code B. Static C. Heap D. Stackarrow_forwardBuild an Android App that shows a list of your favorite books' details. The App should have a set of java files that implement an adapter with RecyclerView. The details of the java files you need to create are described below. RecycleViewExample RHONDA BYRNE 2016 Listen to Your Heart: The London Adventure Ruskin Bond 2010 Business of Sports: The Winning Formula for Success Vinit Karnik 2022 A Place Called Home Preeti Shenoy 2018 Vahana Masterclass Alfredo Covelli 2016 The Little Book of Encouragement Dalai Lama 2021 Platform Scale: For A Post-Pandemic World Sangeet Paul Choudary 2021 Unfinished Priyanka Chopra Jonas 2018 1. The first java file should contain a data field which is in this case: Book_name, Author_name, Publication_year. 2. The second java file contains BookViewHolder. 3. The third java file contains the BookAdapter which is act as a bridge between the data items and the View inside of RecycleView. 4. The Mainactivity java file will contain some samples data to display.…arrow_forwardmodule : java Question3: (30 MARKS) Passenger Rail Agency for South Africa Train Scheduling System Problem Statement Design and implement a train scheduling system for Prasa railway network. The system should handle the following functionalities: 1. Scheduling trains: Allow the addition of train schedules, ensuring that no two trains use the same platform at the same time at any station. 2. Dynamic updates: Enable adding new train schedules and canceling existing ones. 3. Real-time simulation: Use multithreading to simulate the operation of trains (e.g., arriving, departing). 4. Data management: Use ArrayList to manage train schedules and platform assignments. Requirements 1. Add Train Schedule, Cancel Scheduled Train, View Train Schedules and Platform Management 2. Concurrency Handling with Multithreading i.e Use threads to simulate train operations, Each…arrow_forward
- Question D.4: FIFO Page Replacement Consider the following page reference string: e, c, b, e, a, g, d, c, e, g, d, a Considering 4 frames, fill in the following table and then answer how many page faults would occur with the FIFO page replacement algorithm. RS: reference string; FO: frame 0, F1: frame 1, etc. Hint: all frames are initially empty, so your first unique pages will all cost one fault each. Time 1234567891011 12 RS e cb e agd ce g d a FO F1 F2 F3 Page fault? b) Total # page faults: c) Briefly (1-2 sentences) explain Belady's Anomaly that can occur in FIFO Page Replacement.arrow_forwardConsider a system that uses a fixed-partition scheme, with equal partitions of size 2" bytes, and the main memory has 2¹8 bytes. A process table is maintained with a pointer to the resident partition for each resident process. How many bits are required for the pointer in the process table? Show all your steps.arrow_forwardUse the same semaphore notation shown above to describe how we can ensure the execution order of the following process execution graph: P6 P2 P7 P1 P3 P4 P5 Use all of the following semaphores in your answer: s1=0; s2=0; s3=0; s4=0; s5=0; s6=0;arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
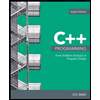
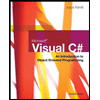
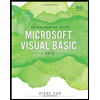
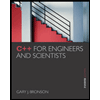
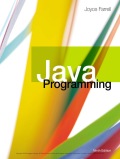
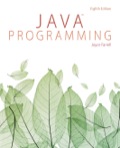