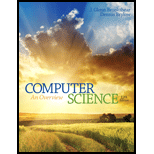
Explanation of Solution
The algorithm for printing a linked list in reverse order using a stack is shown below:
Procedure ReverseLinkedListPrint(List)
CurrentPtr ← Head pointer of list
While (CurrentPtr is not equal to NULL) do
Push the entry pointed to by CurrentPtr on to stack;
CurrentPtr ← Value of next pointer in entry pointed to by CurrentPtr.
While (Stack is not empty) do
Print the top value in the stack
Pop an entry from the stack.
Algorithm explanation:
- The given algorithm is used to print a linked list in reverse order using a stack.
- From the above algorithm, first define the procedure “ReverseLinkedListPrint” with argument “List”...
Explanation of Solution
Recursive function for printing the linked list in reverse order:
The recursive function for printing the linked list in reverse order is shown below:
Function ReverseLinkedListPrint(List)
If the head pointer of List is not NULL, then
Recursively call the function "ReverseLinkedListPrint" with the first entry of given List;
Print the first entry in List
Function Explanation:
- The given function is used to print the linked list in reverse order using recursive function.
- First define the function “ReverseLinkedListPrint”...

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Computer Science: An Overview (12th Edition)
- What are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forwardDiscuss with appropriate examples the types of relationships in a database. Give one reference with your answer.arrow_forwardDetermine the velocity error constant (k,) for the system shown. + R(s)- K G(s) where: K=1.6 A(s+B) G(s) = as²+bs C(s) where: A 14, B =3, a =6. and b =10arrow_forward
- • Solve the problem (pls refer to the inserted image)arrow_forwardWrite .php file that saves car booking and displays feedback. There are 2 buttons, which are <Book it> <Select a date>. <Select a date> button gets an input from the user, start date and an end date. Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, it books cars for specific dates, with bookings saved. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. In the booking.json file, if the Car ID and start date and end date matches, it fails Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert).arrow_forwardWrite .php file with the html that saves car booking and displays feedback. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. There are 2 buttons, which are <Book it> <Select a date> Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, book cars for specific dates, with bookings saved. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert). And then add an additional feature that only free dates are selectable (e.g., calendar view).arrow_forward
- • Solve the problem (pls refer to the inserted image) and create line graph.arrow_forwardwho started the world wide webarrow_forwardQuestion No 1: (Topic: Systems for collaboration and social business The information systems function in business) How does Porter's competitive forces model help companies develop competitive strategies using information systems? • List and describe four competitive strategies enabled by information systems that firms can pursue. • Describe how information systems can support each of these competitive strategies and give examples.arrow_forward
- Data communıcatıon digital data is transmitted via analog ASK and PSK are used together to increase the number of bits transmitted a)For m=8,suggest a solution and define signal elements , and then draw signals for the following sent data data = 0 1 0 1 1 0 0 0 1 0 1 1arrow_forwardDatacommunicationData = 1 1 0 0 1 0 0 1 0 1 1 1 1 0 0a) how many bıts can be detected and corrected by this coding why prove?b)what wıll be the decision of the reciever if it recieve the following codewords why?arrow_forwardpattern recognitionPCA algor'thmarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
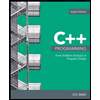