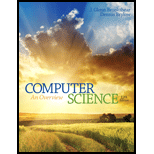
Explanation of Solution
Modified interface for Queue and its code:
The modified interface for queue and its corresponding queue of integer’s implementation in java and C# is shown below:
//Interface for QueueType
interface QueueType
{
//Function declaration to add a value to queue
public void insertQueue(int item);
//Function declaration to remove a value from queue
public int removeQueue();
//Function declaration for check if queue is empty
public boolean isEmpty();
//Function declaration for check if queue is Full
public boolean isFull();
}
//Class for QueueOfInteger
class QueueOfIntegers implements QueueType
{
//Initializes the size of queue
private int size = 20;
//Create an array for QueueEntries
private int[] QueueEntries = new int[size];
/* Declare the variable for front of queue, rear of queue and length of queue*/
private int frontQueue, rearQueue, queueLength;
//Function definition for insert Queue
public void insertQueue(int NewEntry)
{
//If the rearQueue is equal to '-1', then
if (rearQueue == -1)
{
//Assign the frontQueue to "0"
frontQueue = 0;
//Assign the rearQueue to "0"
rearQueue = 0;
/* Assign the Array of QueueEntries to NewEntry */
QueueEntries[rearQueue] = NewEntry;
}
/* If the rearQueue+1 is greater than or equal to "size" */
else if (rearQueue + 1 >= size)
System.out.println("Queue Overflow Exception");
//If the rearQueue+1 is less than "size"
else if ( rearQueue + 1 < size)
/* Assign the Array of QueueEntries to NewEntry */
QueueEntries[++rearQueue] = NewEntry;
//Increment the queue length
queueLength++ ;
}
//Function definition for remove a value from queue
public int removeQueue()
{
//If the queue is not empty, then
if(!isEmpty())
//Decrement the length of queue
queueLength--;
/* Assign the front of queue entries to element */
int element = QueueEntries[front];
//If the queue front is equal to rear
if(frontQueue == rearQueue)
{
/* Assign the value to front and rear of queue */
frontQueue = -1;
rear...

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Computer Science: An Overview (12th Edition)
- using r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardThe assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of…arrow_forwardI need help to solve the following case, thank youarrow_forward
- You will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forwardConstruct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
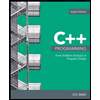
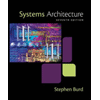
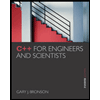
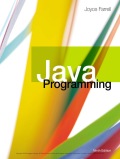
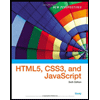