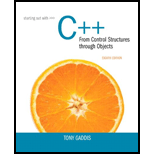
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 7.7, Problem 7.12CP
Program Plan Intro
Output of the program:
Program:
//Header file
#include <iostream>
#include <iomanip>
using namespace std;
//Main function
int main()
{
//Declare and initialize the array
double balance[5] = {100.0, 250.0, 325.0 , 500.0,
1100.0};
//Declare the constant value
const double INTRATE = 0.1;
//Set up the numeric output formatting
cout <<fixed<< showpoint << setprecision(2) ;
//Execute the for loop until the count is less than 5
for (int count = 0; count < 5; count++)
//Calculate the balance
cout << (balance[count] * INTRATE ) << endl;
//Pause the system
system("pause");
//Return the statement
return 0;
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Exercise
docID document text
docID document text
1
hot chocolate cocoa beans
7
sweet sugar
2345
9
cocoa ghana africa
8
sugar cane brazil
beans harvest ghana
9
sweet sugar beet
cocoa butter
butter truffles
sweet chocolate
10
sweet cake icing
11
cake black forest
Clustering by k-means, with preprocessing tokenization, term weighting TFIDF.
Manhattan Distance. Number of cluster is 2. Centroid docID 2 and docID 9.
Change the following code so that there is always at least one way to get from the left corner to the top right, but the labyrinth is still randomized.
The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. Take care that the player and the dragon cannot start off on walls. Also the dragon starts off from a randomly chosen position
public class Labyrinth { private final int size; private final Cell[][] grid;
public Labyrinth(int size) { this.size = size; this.grid = new Cell[size][size]; generateLabyrinth(); }
private void generateLabyrinth() { Random rand = new Random(); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { // Randomly create walls and paths grid[i][j] = new Cell(rand.nextBoolean()); } } // Ensure start and end are…
Change the following code so that it checks the following 3 conditions:
1. there is no space between each cells (imgs)
2. even if it is resized, the components wouldn't disappear
3. The GameGUI JPanel takes all the JFrame space, so that there shouldn't be extra space appearing in the frame other than the game.
Main():
Labyrinth labyrinth = new Labyrinth(10);
Player player = new Player(9, 0); Dragon dragon = new Dragon(9, 9);
JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon);
frame.add(gui); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true);
public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; //labyrinth, player, dragon are just public classes
private final ImageIcon playerIcon = new ImageIcon("data/images/player.png");…
Chapter 7 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 7.3 - Define the following arrays: A) empNums, a...Ch. 7.3 - Prob. 7.2CPCh. 7.3 - Prob. 7.3CPCh. 7.3 - Prob. 7.4CPCh. 7.3 - What is array bounds checking? Does C++ perform...Ch. 7.3 - What is the output of the following code? int...Ch. 7.3 - The following program skeleton contains a...Ch. 7.7 - Given the following array definition: int values[]...Ch. 7.7 - Given the following array definition: int nums[5]...Ch. 7.7 - Prob. 7.12CP
Ch. 7.7 - What is the output of the following code? (You may...Ch. 7.8 - Prob. 7.14CPCh. 7.8 - Prob. 7.15CPCh. 7.8 - When used as function arguments, are arrays passed...Ch. 7.8 - What is the output of the following program? (You...Ch. 7.8 - The following program skeleton, when completed,...Ch. 7.10 - Prob. 7.19CPCh. 7.10 - How many elements are in the following array?...Ch. 7.10 - Write a statement that assigns the value 56893.12...Ch. 7.10 - Prob. 7.22CPCh. 7.10 - Prob. 7.23CPCh. 7.10 - Fill in the table below so that it shows the...Ch. 7.10 - Write a function called displayArray7. The...Ch. 7.10 - A video rental store keeps DVDs on 50 racks with...Ch. 7.12 - Prob. 7.27CPCh. 7.12 - Write a definition statement for a vector named...Ch. 7.12 - Prob. 7.29CPCh. 7.12 - Write a definition statement for a vector named...Ch. 7.12 - Prob. 7.31CPCh. 7.12 - snakes is a vector of doubles, with 10 elements....Ch. 7 - Prob. 1RQECh. 7 - Look at the following array definition: int...Ch. 7 - Why should a function that accepts an array as an...Ch. 7 - Prob. 4RQECh. 7 - Prob. 5RQECh. 7 - Prob. 6RQECh. 7 - Prob. 7RQECh. 7 - Assuming that numbers is an array of doubles, will...Ch. 7 - Prob. 9RQECh. 7 - Prob. 10RQECh. 7 - How do you establish a parallel relationship...Ch. 7 - Prob. 12RQECh. 7 - When writing a function that accepts a...Ch. 7 - What advantages does a vector offer over an array?Ch. 7 - Prob. 15RQECh. 7 - The size declarator must be a(n) ________ with a...Ch. 7 - Prob. 17RQECh. 7 - Prob. 18RQECh. 7 - The number inside the brackets of an array...Ch. 7 - C++ has no array ________ checking, which means...Ch. 7 - Starting values for an array may be specified with...Ch. 7 - If an array is partially initialized, the...Ch. 7 - If the size declarator of an array definition is...Ch. 7 - By using the same _________ for multiple arrays,...Ch. 7 - Prob. 25RQECh. 7 - Prob. 26RQECh. 7 - To pass an array to a function, pass the ________...Ch. 7 - A(n) _______ array is like several arrays of the...Ch. 7 - Its best to think of a two-dimensional array as...Ch. 7 - Prob. 30RQECh. 7 - Prob. 31RQECh. 7 - When a two-dimensional array is passed to a...Ch. 7 - The ________________ is a collection of...Ch. 7 - The two types of containers defined by the STL are...Ch. 7 - The vector data type is a(n) ____________...Ch. 7 - Prob. 36RQECh. 7 - To store a value in a vector that docs nor have a...Ch. 7 - To determine the number of elements in a vector,...Ch. 7 - Use the _______________ member function to remove...Ch. 7 - To completely clear the contents of a vector, use...Ch. 7 - Prob. 41RQECh. 7 - Prob. 42RQECh. 7 - In a program, you need to store the identification...Ch. 7 - Prob. 44RQECh. 7 - In a program, you need to store the populations of...Ch. 7 - The following code totals the values in two...Ch. 7 - Prob. 47RQECh. 7 - Prob. 48RQECh. 7 - Prob. 49RQECh. 7 - Prob. 50RQECh. 7 - Prob. 51RQECh. 7 - T F The individual elements of an array are...Ch. 7 - T F The first element in an array is accessed by...Ch. 7 - Prob. 54RQECh. 7 - Prob. 55RQECh. 7 - T F Subscript numbers may be stored in variables.Ch. 7 - T F You can write programs that use invalid...Ch. 7 - Prob. 58RQECh. 7 - T F The values in an initialization list are...Ch. 7 - T F C++ allows you to partially initialize an...Ch. 7 - T F If an array is partially initialized, the...Ch. 7 - T F If you leave an element uninitialized, you do...Ch. 7 - T F If you leave out the size declarator of an...Ch. 7 - T F The uninitialized elements of a string array...Ch. 7 - T F You cannot use the assignment operator to copy...Ch. 7 - Prob. 66RQECh. 7 - T F To pass an array to a function, pass the name...Ch. 7 - T F When defining a parameter variable to hold a...Ch. 7 - T F When an array is passed to a function, the...Ch. 7 - T F A two-dimensional array is like several...Ch. 7 - T F Its best to think of two-dimensional arrays as...Ch. 7 - T F The first size declarator (in the declaration...Ch. 7 - T F Two-dimensional arrays may be passed to...Ch. 7 - T F C++ allows you to create arrays with three or...Ch. 7 - Prob. 75RQECh. 7 - T F To use a vector, you must include the vector...Ch. 7 - T F vectors can report the number of elements they...Ch. 7 - T F You can use the [ ] operator to insert a value...Ch. 7 - T F If you add a value to a vector that is already...Ch. 7 - int sixe; double values [size];Ch. 7 - Prob. 81RQECh. 7 - Prob. 82RQECh. 7 - Prob. 83RQECh. 7 - int numbers[8] ={1,2, , ,4, , 5};Ch. 7 - float ratings[] ;Ch. 7 - Prob. 86RQECh. 7 - Prob. 87RQECh. 7 - Prob. 88RQECh. 7 - void showValues(int nums [4][]) { For (rows = 0;...Ch. 7 - Prob. 90RQECh. 7 - Largest/Smallest Array Values Write a program that...Ch. 7 - Rainfall Statistics Write a program that lets the...Ch. 7 - Chips and Salsa Write a program that lets a maker...Ch. 7 - Larger than n In a program, write a function that...Ch. 7 - Monkey Business A local zoo wants to keep track of...Ch. 7 - Rain or Shine An amateur meteorologist wants to...Ch. 7 - Number Analysis Program Write a program that asks...Ch. 7 - Lo Shu Magic Square The Lo Shu Magic Square is a...Ch. 7 - Payroll Write a program that uses the following...Ch. 7 - Drivers License Exam The local Drivers License...Ch. 7 - Prob. 11PCCh. 7 - Grade Book A teacher has five students who have...Ch. 7 - Lottery Application Write a program that simulates...Ch. 7 - vector Modification Modify the National Commerce...Ch. 7 - World Series Champions If you have downloaded this...Ch. 7 - Name Search If you have downloaded this books...Ch. 7 - Tic-Tac-Toe Game Write a program that allows two...Ch. 7 - 2D Array Operations Write a program that creates a...Ch. 7 - Prob. 20PC
Knowledge Booster
Similar questions
- Make the following game user friendly with GUI, with some simple graphics. The GUI should be in another seperate class, with some ImageIcon, and Game class should be added into the pane. The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return…arrow_forwardDiscuss the negative and positive impacts or information technology in the context of your society. Provide two references along with with your answerarrow_forwardA cylinder of diameter 10 cm rotates concentrically inside another hollow cylinder of inner diameter 10.1 cm. Both cylinders are 20 cm long and stand with their axis vertical. The annular space is filled with oil. If a torque of 100 kg cm is required to rotate the inner cylinder at 100 rpm, determine the viscosity of oil. Ans. μ= 29.82poisearrow_forward
- Make the following game user friendly with GUI, with some simple graphics The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return isWall; } public void setWall(boolean isWall) { this.isWall = isWall; } @Override public String toString() {…arrow_forwardPlease original work What are four of the goals of information lifecycle management think they are most important to data warehousing, Why do you feel this way, how dashboards can be used in the process, and provide a real life example for each. Please cite in text references and add weblinksarrow_forwardThe following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forward
- In this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forwardHow do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forwardYou are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forward
- Distributed Systems: Consistency Models fer to page 45 for problems on data consistency. structions: Compare different consistency models (e.g., strong, eventual, causal) for distributed databases. Evaluate the trade-offs between availability and consistency in a given use case. Propose the most appropriate model for the scenario and explain your reasoning. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardOperating Systems: Deadlock Detection fer to page 25 for problems on deadlock concepts. structions: • Given a system resource allocation graph, determine if a deadlock exists. If a deadlock exists, identify the processes and resources involved. Suggest strategies to prevent or resolve the deadlock and explain their trade-offs. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardArtificial Intelligence: Heuristic Evaluation fer to page 55 for problems on Al search algorithms. tructions: Given a search problem, propose and evaluate a heuristic function. Compare its performance to other heuristics based on search cost and solution quality. Justify why the chosen heuristic is admissible and/or consistent. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
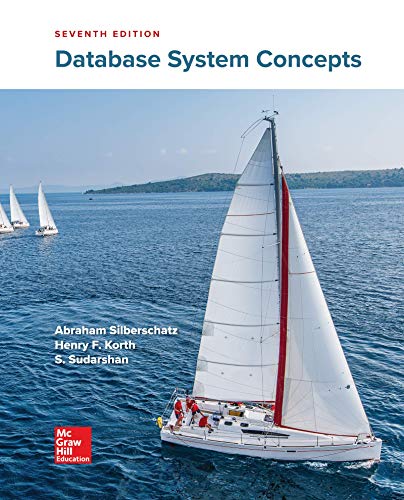
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
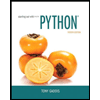
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
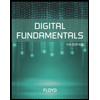
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
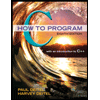
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
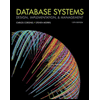
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
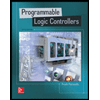
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education