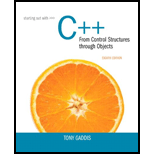
The Standard Template Library (STL) includes the collection of data types and
- A special data type called vector is offered by STL which is useful for standard arrays.
A vector stores the elements or values in sequence order and the values are stored in continuous memory locations.
- The subscript operator “[]” in the vector helps to read the content of each element.
Initialization of vector:
In C++, the vector is initialized with the respective value as like the array but it does not use the “=” operator before the list and uses the keyword “vector”.
- The initialization list values are enclosed with the braces.
Consider the following statement:
//Declaring a vector with four values
vector <int> values {40, 32, 15, 17};
In the above line, the keyword “vector” represents the vector declaration, “<int>” represents that the vector holds integer type values, “values” represents the name of the vector, and the values inside the braces refers the values of the vector “values”.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
- Please include comments and docs comments on the program. The two other classes are Attraction and Entertainment.arrow_forwardObject-Oriented Programming In this separate files. ent, you'll need to build and run a small Zoo in Lennoxville. All classes must be created in Animal (5) First, start by building a class that describes an Animal at a Zoo. It should have one private instance variable for the name of the animal, and one for its hunger status (fed or hungry). Add methods for setting and getting the hunger satus variable, along with a getter for the name. Consider how these should be named for code clarity. For instance, using a method called hungry () to make the animal hungry could be used as a setter for the hunger field. The same logic could be applied to when it's being fed: public void feed () { this.fed = true; Furthermore, the getter for the fed variable could be named is Fed as it is more descriptive about what it answers when compared to get Fed. Keep this technique in mind for future class designs. Zoo (10) Now we have the animals designed and ready for building a little Zoo! Build a class…arrow_forward1.[30 pts] Answer the following questions: a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 0 1 1 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report. 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisim in your report. 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB C…arrow_forward
- When using functions in python, it allows us tto create procedural abstractioons in our programs. What are 5 major benefits of using a procedural abstraction in python?arrow_forwardFind the error, assume data is a string and all variables have been declared. for ch in data: if ch.isupper: num_upper = num_upper + 1 if ch.islower: num_lower = num_lower + 1 if ch.isdigit: num_digits = num_digits + 1 if ch.isspace: num_space = num_space + 1arrow_forwardFind the Error: date_string = input('Enter a date in the format mm/dd/yyyy: ') date_list = date_string.split('-') month_num = int(date_list[0]) day = date_list[1] year = date_list[2] month_name = month_list[month_num - 1] long_date = month_name + ' ' + day + ', ' + year print(long_date)arrow_forward
- Find the Error: full_name = input ('Enter your full name: ') name = split(full_name) for string in name: print(string[0].upper(), sep='', end='') print('.', sep=' ', end='')arrow_forwardPlease show the code for the Tikz figure of the complex plane and the curve C. Also, mark all singularities of the integrand.arrow_forward11. Go to the Webinars worksheet. DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: a. Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. b. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. c. Determine and enter the constraints based on the information provided in Table 3. d. Use Simplex LP as the solving method to find a global optimal solution. e. Save the Solver model below the Maximum weekly profit model label. f. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and…arrow_forward
- Go to the Webinars DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. Determine and enter the constraints based on the information provided in Table 3. Use Simplex LP as the solving method to find a global optimal solution. Save the Solver model below the Maximum weekly profit model label. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and Thursday…arrow_forwardI want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)? When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene. Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency. To elaborate,…arrow_forwardI want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.To elaborate, what…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
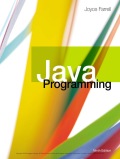
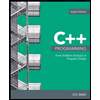
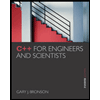
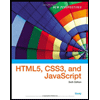
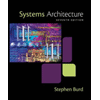