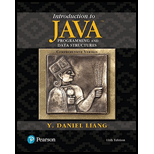
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 7.11, Problem 7.11.1CP
Program Plan Intro
Array:
An array is defined as a group that consists of similar data types.
- The array size must be specified by an “int” value and not long or short.
- In Java, all arrays are allocated dynamically.
- An array is always indexed, starting from 0.
Selection sort
- Sorting is a process to place the elements in order.
- Selection sort is used to sort the group of data.
- Selection sort finds the smallest element in the array by comparing all the elements.
- The element values in the array are exchanged to place the smallest element in the first position.
- Continuously, it finds the next smallest element and exchanges it in the successive positions.
- The exchange process may place one or more elements in its permanent place.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Given an array arg[] below, use the incremental approach to sort it in assending order
11
42
35
38
22
45
18
39
3
Please do it in python
Sort the following array of numbers using counting sort and quick sort. Show all necessary steps of sorting
in your run.
[23,9,10,34,7,21,18,24,15]
Chapter 7 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 7.2 - Prob. 7.2.1CPCh. 7.2 - Prob. 7.2.2CPCh. 7.2 - What is the output of the following code? int x =...Ch. 7.2 - Indicate true or false for the following...Ch. 7.2 - Which of the following statements are valid? a....Ch. 7.2 - Prob. 7.2.6CPCh. 7.2 - What is the array index type? What is the lowest...Ch. 7.2 - Write statements to do the following: a. Create an...Ch. 7.2 - What happens when your program attempts to access...Ch. 7.2 - Identify and fix the errors in the following code:...
Ch. 7.2 - What is the output of the following code? 1....Ch. 7.4 - Will the program pick four random cards if you...Ch. 7.5 - Use the arraycopy method to copy the following...Ch. 7.5 - Prob. 7.5.2CPCh. 7.7 - Suppose the following code is written to reverse...Ch. 7.8 - Prob. 7.8.1CPCh. 7.8 - Prob. 7.8.2CPCh. 7.9 - Prob. 7.9.1CPCh. 7.9 - Prob. 7.9.2CPCh. 7.10 - If high is a very large integer such as the...Ch. 7.10 - Prob. 7.10.2CPCh. 7.10 - Prob. 7.10.3CPCh. 7.11 - Prob. 7.11.1CPCh. 7.11 - How do you modify the selectionSort method in...Ch. 7.12 - What types of array can be sorted using the...Ch. 7.12 - To apply java.util.Arrays.binarySearch (array,...Ch. 7.12 - Show the output of the following code: int[] list1...Ch. 7.13 - This book declares the main method as public...Ch. 7.13 - Show the output of the following program when...Ch. 7 - (Assign grades) Write a program that reads student...Ch. 7 - (Reverse the numbers entered) Write a program that...Ch. 7 - (Count occurrence of numbers) Write a program that...Ch. 7 - (Analyze scores) Write a program that reads an...Ch. 7 - (Print distinct numbers) Write a program that...Ch. 7 - (Revise Listing 5.1 5, PrimeNumber.java) Listing...Ch. 7 - (Count single digits) Write a program that...Ch. 7 - (Average an array) Write two overloaded methods...Ch. 7 - (Find the smallest element) Write a method that...Ch. 7 - Prob. 7.10PECh. 7 - (Statistics: compute deviation) Programming...Ch. 7 - (Reverse an array) The reverse method in Section...Ch. 7 - Prob. 7.13PECh. 7 - Prob. 7.14PECh. 7 - 7 .15 (Eliminate duplicates) Write a method that...Ch. 7 - (Execution time) Write a program that randomly...Ch. 7 - Prob. 7.17PECh. 7 - (Bubble sort) Write a sort method that uses the...Ch. 7 - (Sorted?) Write the following method that returns...Ch. 7 - (Revise selection sort) In Listing 7 .8, you used...Ch. 7 - (Sum integers) Write a program that passes an...Ch. 7 - (Find the number of uppercase letters in a string)...Ch. 7 - (Game: locker puzzle) A school bas 100 lockers and...Ch. 7 - (Simulation: coupon collectors problem) Coupon...Ch. 7 - (Algebra: solve quadratic equations) Write a...Ch. 7 - (Strictly identical arrays) The arrays 1ist1 and...Ch. 7 - (Identical arrays) The arrays 1ist1 and 1ist2 are...Ch. 7 - (Math: combinations) Write a program that prompts...Ch. 7 - (Game: pick four cards) Write a program that picks...Ch. 7 - (Pattern recognition: consecutive four equal...Ch. 7 - (Merge two sorted Lists) Write the following...Ch. 7 - (Partition of a list) Write the following method...Ch. 7 - Prob. 7.33PECh. 7 - (Sort characters in a string) Write a method that...Ch. 7 - (Game: hangman) Write a hangman game that randomly...Ch. 7 - (Game: Eight Queens) The classic Eight Queens...Ch. 7 - Prob. 7.37PE
Knowledge Booster
Similar questions
- Given an array A= {12, 11, 13, 5, 6}, sort it out using a technique illustrated in insertion sortarrow_forward1. Please work on a piece of paper. Use insertion sort to sort the following array {6, 2, 5, 9, 4, 2, 3, 7, 1, 8, 5). Any observations here? Is insertion sort stable or not? Is insertion sort in-place or not?arrow_forwardSO You have been given two integer arrays/lists (ARR1 and ARR2) of size N and M, respectively. You need to print their intersection; An intersection for this problem can be defined when both the arrays/lists contain a particular value or to put it in other words, when there is a common value that exists in both the arrays/lists.Note :Input arrays/lists can contain duplicate elements.The intersection elements printed would be in the order they appear in the first sorted array/list (ARR1).Input format :The first line of input contains an integer 'N' representing the size of the first array/list.The second line contains 'N' single space separated integers representing the elements of the first the array/list.The third line contains an integer 'M' representing the size of the second array/list.The fourth line contains 'M' single space separated integers representing the elements of the second array/list.Output format :Print the intersection elements. Each element is printed in a separate…arrow_forward
- Q:During each iteration of Quick Sort algorithm, the first element of array is selected as a pivot. The algorithm for Quick Sort is given below. Modify it in such a way that last element of array should be selected as a pivot at each iteration. Also explain the advantages.arrow_forwardConsider the following array of int values. [17, 11, 6, 22, -3, 8, 4, 22, 2, 35, -4, 10] Write the contents of the array after 4 passes of the outermost loop of selection sort. Assume selection sort is sorting slots from low-to-high Write the contents of the array during each of the the recursive calls of merge sort.arrow_forwardFollowing is the function for interpolation search. This searching algorithm estimates the position (index) of a key in array based on the elements in the first position and last position in the array, and the length of array. The array must be sorted in ascending order. Suppose array A contains the following 15 elements: A = [1, 3, 3, 10, 17, 22, 22, 22, 24, 25, 26, 27, 27, 28, 28] At first iteration, at which position (index) the element of 24 is estimated in array A? In which part of array (starting index and ending index) the searching should continue? How many iterations the searching are performed until the element of 24 is found? int InterpolationSearch(int x[], int key, int n) { int mid, min = 0, max = n-1; while(x[min] < key && x[max] > key) { mid = min + ((key-x[min])*(max-min)) / (x[max]-x[min]); if(x[mid] < key) min = mid + 1; else if(x[mid] > key) max = mid - 1; else return mid; } if…arrow_forward
- Using the C language, Write a program that to perform an insertion sort for the following array. Please provide the time complexity of the insertion sort. 4 12 7 8 2 5 15 Use the same array to write a program using merge sort and provide a time complexity of merge sortarrow_forwardSort the following array in decreased manner using insertion sort [12, 2, 5, 10, 15, 27,4], then sort the same array another time in increased manner and compare between the number of swaps in the two casesarrow_forwardFollowing is the given python code for Merge Sort. Dry run all the steps on your register for the following given array and fill the missing blanks in the figure given below If you run the following lines: random_list_of_nums = [12, 45, 6, 25, 17, 60, 8] random_list_of_nums = merge_sort(random_list_of_nums) print(random_list_of_nums)arrow_forward
- Modify the given code according to what it ask you to do Show your modified code in a picturearrow_forwardPlease work on a piece of paper. Use insertion sort to sort the following array {6, 2, 5,9, 4, 2, 3, 7, 1, 8, 5}. Any observations here? Is insertion sort stable or not? Is insertion sort in place or not?arrow_forwardIn an array-based implementation of a List, why does the add operation take O(n) time in the average case? Select one: a. The time it takes to shift entries over to make room in the array depends on the number of entries in the List b. The time to copy the current entries into a newly allocated, larger array depends on the number of entries in the List c. The time to access the position in the array to add the new entry depends on the number of entries in the List d. The time to access the position in the array to add the new entry depends on the capacity of the arrayarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
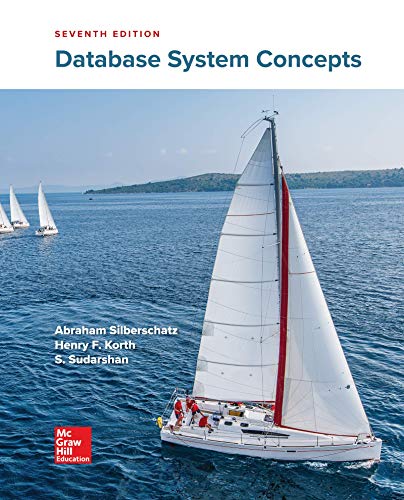
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
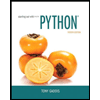
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
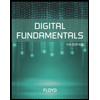
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
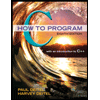
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
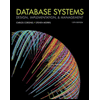
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
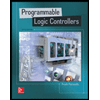
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education