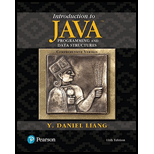
(Statistics: compute deviation)
To compute the standard deviation with this formula, you have to store the individual numbers using an array, so they can be used after the mean is obtained.
Your program should contain the following methods:
/** Compute the deviation of double values */
public static double deviation(double[] x)
/** Compute the mean of an array of double values */
public static double mean(double[] x)
Write a test program that prompts the user to enter 10 numbers and displays the mean and standard deviation, as presented in the following sample run:

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Starting Out with C++: Early Objects (9th Edition)
Modern Database Management
Database Concepts (7th Edition)
C Programming Language
Software Engineering (10th Edition)
- (IN C LANGUAGE) Cumulative Addition: Computer selects a number between 7 and 23 at random. User will only add 2, 3 or 5 numbers to reach that number.For example: To reach 14: User will enter 5 5 2 2 (4 input).Also he can enter 2 2 2 2 2 2 2 (7 input) or 3 3 3 3 2 (5 input). https://www.bartleby.com/questions-and-answers/in-c-language-cumulative-addition-computer-selects-a-number-between-7-and-23-at-random.-user-will-on/0509c740-d993-44ed-a468-7e02da552600arrow_forward(Sort three numbers) Write the following function to display three numbers in increasing order: def displaySortedNumbers(num1, num2, num3): Write a test program that prompts the user to enter three numbers and invokes the function to display them in increasing order. Here are some sample runs:arrow_forward(Algebra: solve 2 x 2 linear equations) You can use Cramer's rule to solve the following 2 x 2 system of linear equation: ax + by = e ed – bf af- ec ad - bc cx + dy = f ad – bc y = Write a program that prompts the user to enter a and f and display the result. If ad - bc is 0 b, c, d , e, , report that The equation has no solution.arrow_forward
- Thank youarrow_forward5. (Algebra: solve 2 X 2 linear equations) You can use Cramer's rule to solve the following 2 X 2 system of linear equation: ax + by = e cx + dy = f ● x = ed - bf bc ad y = af - ec ad bc - Write a program that prompts the user to enter a, b, c, d, e, and f and display the result. If ad- bc is 0, report that The equation has no solution. Enter a, b, c, d, e, f: 9.0, 4.0, 3.0, -5.0, -6.0, -21.0 Enter x is -2.0 and y is 3.0 Enter a, b, c, d, e, f: 1.0, 2.0, 2.0, 4.0, 4.0, 5.0 Enter The equation has no solutionarrow_forward(Same-number subsequence) JAVA Class Name: Exercise22_05 Write an O(n) program that prompts the user to enter a sequence of integers ending with 0 and finds the longest subsequence with the same number. Sample Run 1 Enter a series of numbers ending with 0:2 4 4 8 8 8 8 2 4 4 0The longest same number sequence starts at index 3 with 4 values of 8 Sample Run 2 Enter a series of numbers ending with 0: 34 4 5 4 3 5 5 3 2 0 The longest same number sequence starts at index 5 with 2 values of 5arrow_forward
- ) /₹3soos alspaarrow_forward1) Simple Calculator: In Python, implement a simple calculator that does the following operations: summation, subtraction, multiplication, division, sqrt, power, natural log and abs. a) Follow the instructions below: To work with the calculator, the user is asked to enter the first number, then the operation, and finally, a second number if required. Your code has to recognize the need for the second number and ask for it if required. After performing one operation, the calculator prints the output of the operation. After performing one operation, the calculator must not exit. It has to start again for the next operation. The calculator will be closed if the user writes 'e' as any input. Use functions to perform the operations and the appropriate conditions to prevent common errors such as entering characters as one of the numbers etc. b) Run your code and provide the results for at least one example per operation. - -arrow_forward4. Odd-Even-inator by CodeChum Admin My friends are geeking out with this new device I invented. It checks if a number is even or odd! ? Do you want to try it out? Instructions: In the code editor, you are provided with a function that checks whether a number is even or odd. Your task is to ask the user for the number of integer, n, they want to input and then the actual n number values. For each of the number, check whether it is even or odd using the function provided for you. Make sure to print the correct, required message. Input 1. Integer n 2. N integer valuesarrow_forward
- ▼ Part A - The effect of an arithmetic shift on signed numbers Let's look at see what happens to signed numbers during a shift operation. As with most problems with number representations, errors can be intermittent. Sometimes the code will work as expected, and other times it will behave in a manner that seems to be totally arbitrary. Consider the following code fragment that makes use of the fact that shifting a value left by one place multiplies the number by 2. By passing in the number to be multiplied and the power of 2 to multiply it by (for example 4 = 22) the correct answer should be passed back from the function after the results are printed for the user to examine. signed int mult_2_to_n (signed int num, int n) { signed int result; result = num << n; printf("%d multiplied by 2^%d %d\n", num, n, result); return result; } Using a signed number as the manipulated integer may cause an error in some cases. Several approaches may be used to fix the problem. Which solutions below…arrow_forward: Reduce the following Boolean expressions to their simplest form *: F = abc + (a + c) + bc (number of literals)arrow_forwardFactorial) The factorial of a nonnegative integer n is written n! (pronounced “n factorial”) and is defined as follows:For example, 5!= 5.4.3.2.1 , which is 120. Use while statements in each of the following:A. Write a program that reads a nonnegative integer and computes and prints its factorial.B. Write a program that estimates the value of the mathematical constant e by using the formula:Prompt the user for the desired accuracy of e (i.e., the number of terms in the summation).C. Write a program that computes the value of by using the formula Prompt the user for the desired accuracy of e (i.e., thenumber of terms in the summation).arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
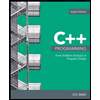
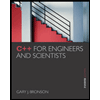
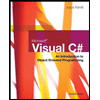