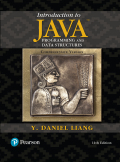
(Merge two sorted Lists) Write the following method that merges two sorted lists into a new sorted list:
public static int[] merge(int[] list1, int[] list2)
Implement the method in a way that takes at most list 1. length + list2.
length comparisons. See liveexample.pearsoncmg.com/dsanimation/ MergeSortNeweBook.html for an animation of the implementation. Write a test

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects (9th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Mechanics of Materials (10th Edition)
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Database Concepts (8th Edition)
- Do not copy froom outside sources as they have different instructions. In python. please include docstring. The Strawberry Stand methods are:* init method - takes one parameter, the name of the stand; initializes the name to that value, initializes current day to zero, initializes the menu to an empty dictionary, and initializes the sales record to an empty list* a get method for the name: get_name()* add_menu_item - takes as a parameter a MenuItem object and adds it to the menu dictionary. New items can be added to the menu on any day* enter_sales_for_today - takes as a parameter a dictionary where the keys are names of items sold and the corresponding values are how many of the item were sold. If an item in the menu doesn't appear in the dictionary, then there were no sales of that item on that day. If the name of any item sold doesn't match the name of any MenuItem in the dictionary of MenuItem objects, this method should do nothing except raise an **InvalidSalesItemError** (you'll…arrow_forwardremove_from_list(my_list, indices): Takes two lists as input. Creates a new list from the first input list by removing the elements at indices given by the integers in the second input list, then return the new list. The indices in the second input list may have any order. For example, if the first input list is [4, 8, 12, 161, and the second input list is [0, 2], then the new list returned from the function should have the 4 and the 12 removed, because they were at indices 0 and 2 of the original list. Note: You do not need to consider negative indices for this function. >> remove_from_list(['abc', 'def', 'ghi'], [1]) ['abc', 'ghi'] >>> strs = ['The', 'quick', 'brown', 'fox'] >>> remove_from_list(strs, [0, 3]) ['quick', 'brown'] >>> strs ['The', 'quick', 'brown', 'fox'] # original list is unchanged >> remove_from_list(['The', 'quick', 'brown', 'fox'], [3, 0]) ['quick', 'brown'] find_last(my_list, x): Takes two inputs: the first being a list and the second being any type. Returns the…arrow_forwardRestrictions: do not use imports, except for the ones given (if any). You may not use any lists or list methods, please do not use try-except statements. I included my is_palindrome functionarrow_forward
- How are ArrayLists and arrays different? (give three significant distinctions)arrow_forwardPlease help me with this using java and recursion. Please also comment the code. Please do not use hashtags when creating theses fractals. And provide an image of the output. (Just pick one) 1) create a sierpenski triangle fract 2) create a viscek fractalsarrow_forwardplease follow instructions correctly. You are required to complete the LinkedList class. This class is used as a linked list that has many methods to perform operations on the linked list. To create a linked list, create an object of this class and use the addFirst or addLast or add(must be completed) to add nodes to this linked list. Your job is to complete the empty methods. For every method you have to complete, you are provided with a header, Do not modify those headers(method name, return type or parameters). You have to complete the body of the method. package chapter02; public class LinkedList { protected LLNode list; public LinkedList() { list = null; } public void addFirst(T info) { LLNode node = new LLNode(info); node.setLink(list); list = node; } public void addLast(T info) { LLNode curr = list; LLNode newNode = new LLNode(info); if(curr == null) { list = newNode; } else…arrow_forward
- 7-Write a procedure that returns the list that contains everything except for the last element of the given (nonempty) list. For example, (last-element ‘(a (b c ) (d)) ) returns (a (b c)). For example, (last-element ‘(a (b c ) (d) ) ) returns (a (b c)) . Then, Manually trace your procedure with the provided example.arrow_forwardTASK 2 Write a Java method that merges two sorted lists into a new sorted list. public static int [] merge(int [] 1list1, int[] list2) Write a Java test Program that prompts the user to enter two sorted lists and displays the merged list. Here is a sample run. Note that the first number in the input indicates the number of the elements in the list. This number is not part of the list. Enter listl: 5 1 5 16 61 111 - Enter Enter list2: 4 2 4 5 6 JEnter The merged 1ist is 1 2 4 5 5 6 16 61 111arrow_forwardData Structure and algorithms ( in Java ) Please solve it urgent basis: Make a programe in Java with complete comments detail and attach outputs image: Question is inside the image also: a). Write a function to insert elements in the sorted manner in the linked list. This means that the elements of the list will always be in ascending order, whenever you insert the data. For example, After calling insert method with the given data your list should be as follows: Insert 50 List:- 50 Insert 40 List:- 40 50 Insert 25 List:- 25 40 50 Insert 35 List:- 25 35 40 50 Insert 40 List:- 25 35 40 40 50 Insert 70 List:- 25 35 40 50 70 b). Write a program…arrow_forward
- Python: Take your searching and sorting functions and put them into a class. The constructor should take a list as a parameter use the classes sort method and store the sorted list in a class property. A "search" method should return true or false if the item is found. A "count" method should return 0 or the number of times the item is found. For fun, you can create a method to generate a list of random numbers (and then sort it).arrow_forwardNEED HELP WITH 2-D list. (PYTHON) For e.g., the following is another example of 2-D multidimensional list. Each row contains student name followed by their grades in 5 subjects: students = [ ['Anna', 98.5, 77.5, 89, 93.5, 85.5], ['Bob', 77, 66.5, 54, 90, 85.5], ['Sam', 98, 97, 89.5, 92.5, 96.5] ] To access, a specific row, you would use students[row_number][column_number]. students[0][0] would print 'Anna' students[0][1] would print 98.5 Create your own 2-D list with at-least 5 students and their grades in 5 subjects like the example above. Write a nested for/while loop that would use find the total of 5 subjects for each student and stores that into a sepearate list. Display the Total for each student.arrow_forwardTry to use the Foreach method on the list and also use the Lambada print statement instead of simple one in Java programming language.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
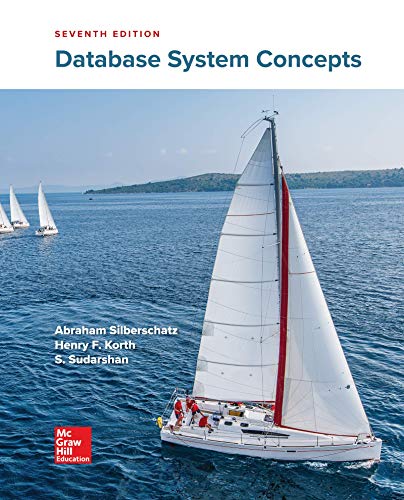
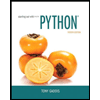
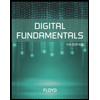
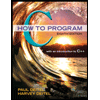
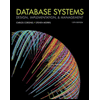
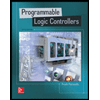