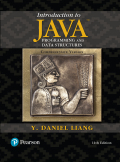
Concept explainers
(a)
Given Program:
public class Test {
//Main Method
public static void main(String[] args) {
//Initialize number
int number = 0;
int[] numbers = new int[1];
m(number, numbers);
//Display the number
System.out.println("number is " + number
+ " and numbers[0] is " + numbers[0]);
}
public static void m(int x, int[] y) {
x = 3;
y[0] = 3;
}
}
(b)
Given Program:
import java.util.Scanner;
public class Test{
//Main Method
public static void main(String[] args) {
//Initialize the numbers
int[] list = {1, 2, 3, 4, 5};
reverse(list);
//Display the list
for (int i = 0; i < list.length; i++)
System.out.print(list[i] + " ");
}
//Method to find the reverse
public static void reverse(int[] list) {
int[] newList = new int[list.length];
for (int i = 0; i < list.length; i++)
newList[i] = list[list.length - 1 - i];
list = newList;
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
- Consider the following expression in C: a/b > 0 && b/a > 0.What will be the result of evaluating this expression when a is zero? What will be the result when b is zero? Would it make sense to try to design a language in which this expression is guaranteed to evaluate to false when either a or b (but not both) is zero? Explain your answerarrow_forwardConsider the following expression in C: a/b > 0 && b/a > 0. What will be the result of evaluating this expression when a is zero? What will be the result when b is zero? Would it make sense to try to design a language in which this expression is guaranteed to evaluate to false when either a or b (but not both) is zero? Explain your answer.arrow_forwardWhat are the major threats of using the internet? How do you use it? How do children use it? How canwe secure it? Provide four references with your answer. Two of the refernces can be from an article and the other two from websites.arrow_forward
- Assume that a string of name & surname is saved in S. The alphabetical characters in S can be in lowercase and/or uppercase letters. Name and surname are assumed to be separated by a space character and the string ends with a full stop "." character. Write an assembly language program that will copy the name to NAME in lowercase and the surname to SNAME in uppercase letters. Assume that name and/or surname cannot exceed 20 characters. The program should be general and work with every possible string with name & surname. However, you can consider the data segment definition given below in your program. .DATA S DB 'Mahmoud Obaid." NAME DB 20 DUP(?) SNAME DB 20 DUP(?) Hint: Uppercase characters are ordered between 'A' (41H) and 'Z' (5AH) and lowercase characters are ordered between 'a' (61H) and 'z' (7AH) in the in the ASCII Code table. For lowercase letters, bit 5 (d5) of the ASCII code is 1 where for uppercase letters it is 0. For example, Letter 'h' Binary ASCII 01101000 68H 'H'…arrow_forwardWhat did you find most interesting or surprising about the scientist Lavoiser?arrow_forward1. Complete the routing table for R2 as per the table shown below when implementing RIP routing Protocol? (14 marks) 195.2.4.0 130.10.0.0 195.2.4.1 m1 130.10.0.2 mo R2 R3 130.10.0.1 195.2.5.1 195.2.5.0 195.2.5.2 195.2.6.1 195.2.6.0 m2 130.11.0.0 130.11.0.2 205.5.5.0 205.5.5.1 R4 130.11.0.1 205.5.6.1 205.5.6.0arrow_forward
- Analyze the charts and introduce each charts by describing each. Identify the patterns in the given data. And determine how are the data points are related. Refer to the raw data (table):arrow_forward3A) Generate a hash table for the following values: 11, 9, 6, 28, 19, 46, 34, 14. Assume the table size is 9 and the primary hash function is h(k) = k % 9. i) Hash table using quadratic probing ii) Hash table with a secondary hash function of h2(k) = 7- (k%7) 3B) Demonstrate with a suitable example, any three possible ways to remove the keys and yet maintaining the properties of a B-Tree. 3C) Differentiate between Greedy and Dynamic Programming.arrow_forwardWhat are the charts (with their title name) that could be use to illustrate the data? Please give picture examples.arrow_forward
- A design for a synchronous divide-by-six Gray counter isrequired which meets the following specification.The system has 2 inputs, PAUSE and SKIP:• While PAUSE and SKIP are not asserted (logic 0), thecounter continually loops through the Gray coded binarysequence {0002, 0012, 0112, 0102, 1102, 1112}.• If PAUSE is asserted (logic 1) when the counter is onnumber 0102, it stays here until it becomes unasserted (atwhich point it continues counting as before).• While SKIP is asserted (logic 1), the counter misses outodd numbers, i.e. it loops through the sequence {0002,0112, 1102}.The system has 4 outputs, BIT3, BIT2, BIT1, and WAITING:• BIT3, BIT2, and BIT1 are unconditional outputsrepresenting the current number, where BIT3 is the mostsignificant-bit and BIT1 is the least-significant-bit.• An active-high conditional output WAITING should beasserted (logic 1) whenever the counter is paused at 0102.(a) Draw an ASM chart for a synchronous system to providethe functionality described above.(b)…arrow_forwardS A B D FL I C J E G H T K L Figure 1: Search tree 1. Uninformed search algorithms (6 points) Based on the search tree in Figure 1, provide the trace to find a path from the start node S to a goal node T for the following three uninformed search algorithms. When a node has multiple successors, use the left-to-right convention. a. Depth first search (2 points) b. Breadth first search (2 points) c. Iterative deepening search (2 points)arrow_forwardWe want to get an idea of how many tickets we have and what our issues are. Print the ticket ID number, ticket description, ticket priority, ticket status, and, if the information is available, employee first name assigned to it for our records. Include all tickets regardless of whether they have been assigned to an employee or not. Sort it alphabetically by ticket status, and then numerically by ticket ID, with the lower ticket IDs on top.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
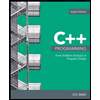
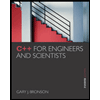
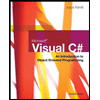