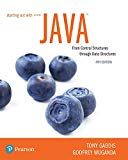
Charge Account Validation
Create a class with a method that accepts a charge account number as its argument. The method should determine whether the number is valid by comparing it to the following list of valid charge account numbers:
These numbers should be stored in an array or an ArrayList object. Use a sequential search to locate the number passed as an argument. If the number is in the array, the method should return true, indicating the number is valid. If the number is not in the array, the method should return false, indicating the number is invalid.
Write a

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Modern Database Management (12th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Modern Database Management
C How to Program (8th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
- 17. Phone Book ArrayList Write a class named PhoneBookEntry that has fields for a person's name and phone number. The class should have a constructor and appropriate accessor and mutator methods. Then write a program that creates at least five PhoneBookEntry objects and stores them in an ArrayList. Use a loop to display the contents of each object in the ArrayList.arrow_forward10. Lottery Application Write a Lottery class that simulates a lottery. The class should have an array of five integers named lotteryNumbers. The constructor should use the Random class (from the Java API) to generate a random number in the range of 0 through 9 for each element in the array. The class should also have a method that accepts an array of five integers that represent a per- son's lottery picks. The method is to compare the corresponding elements in the two arrays and return the number of digits that match. For example, the following shows the lotteryNumbers array and the user's array with sample numbers stored in each. There are two matching digits (elements 2 and 4). lotteryNumbers array: User's array: 7 4 4 2 1 3 7 3 In addition, the class should have a method that returns a copy of the lotteryNumbers array. Demonstrate the class in a program that asks the user to enter five numbers. The program should display the number of digits that match the randomly generated…arrow_forwardWrite code for PersonManagement class. This class contains the array of Person class (code is given below) and also following methods: set: This method receives the array of person class and set the attribute. displayAll: This method will display values of all persons. sort: This method will sort the array according to age values of the persons with ascending order using Selection Sort. reverse: This method will reverse the order of the array. getPersons: This method will return the array of the persons (attribute). displayEvenAgePerson: This method will display all those persons values who have even age. displayOddAgePerson: This method will display all those persons values who have odd age. displayPrimeAgePerson: This method will display only those persons values who have prime number age. Following is the class of Person: public class Person { private String Name; public int age; public void set(String Name, int age) { this.Name =…arrow_forward
- Write code for PersonManagement class. This class contains the array of Person class (code is given below) and also following methods: set: This method receives the array of person class and set the attribute. displayAll: This method will display values of all persons. sort: This method will sort the array according to age values of the persons with ascending order using Selection Sort. reverse: This method will reverse the order of the array. getPersons: This method will return the array of the persons (attribute). displayEvenAgePerson: This method will display all those persons values who have even age. displayOddAgePerson: This method will display all those persons values who have odd age. displayPrimeAgePerson: This method will display only those persons values who have prime number age.arrow_forwardSultan Qaboos University Department of Computer Science COMP2202: Fundamentals of Object Oriented Programming Assignment # 2 (Due 5 November 2022 @23:59) The purpose of this assignment is to practice with java classes and objects, and arrays. Create a NetBeans/IntelliJ project named HW2_YourId to develop a java program as explained below. Important: Apply good programming practices: 1- Provide comments in your code. 2- Provide a program design 3- Use meaningful variables and constant names. 4- Include your name, university id and section number as a comment at the beginning of your code. 5- Submit to Moodle the compressed file of your entire project (HW1_YourId). 1. Introduction: In crowded cities, it's very crucial to provide enough parking spaces for vehicles. These are usually multistory buildings where each floor is divided into rows and columns. Drivers can park their cars in exchange for some fees. A single floor can be modeled as a two-dimensional array of rows and columns. A…arrow_forwardHomework - identical arrays for one-dimensional: Write a test program which prompts a user to enter the size of two arrays as well as to fill their elements. After that, the program checks the same contents of the elements of the two arrays. If these arrays are equal, the program displays message which is "The two arrays are identical". If not equal, displaying "The two arrays are not identical". Write an equals method that reads two arrays and displays these messages above.arrow_forward
- JavaDriver Program Write a driver program that contains a main method and two other methods. Create an Array of Objects In the main method, create an array of Food that holds at least two foods and at least two vegetables. You can decide what data to use. Method: Print Write a method that prints the text representation of the foods in the array in reverse order (meaning from the end of the array to the beginning). Invoke this method from main. Method: Highest Sugar Food Write a method that takes an array of Food objects and finds the Food that has the most sugar. Note that the method is finding the Food, not the grams of sugar. Hint: in looping to find the food, keep track of both the current highest index and value. Invoke this method from main.arrow_forwardWrite code for PersonManagement class. This class contains the array of Person class (code is given below) and also following methods:1. set: This method receives the array of person class and set the attribute.2. displayAll: This method will display values of all persons.3. sort: This method will sort the array according to age values of the persons with ascending order using Selection Sort.4. reverse: This method will reverse the order of the array.5. getPersons: This method will return the array of the persons (attribute).6. displayEvenAgePerson: This method will display all those persons values who have even age.7. displayOddAgePerson: This method will display all those persons values who have odd age.8. displayPrimeAgePerson: This method will display only those persons values who have prime number age.Following is the class of Person: public class Person {private String Name;public int age;public void set(String Name, int age){this.Name = Name;if(age > 0 && age <=…arrow_forward8. Grade Book A teacher has five students who have taken four tests. The teacher uses the following grading scale to assign a letter grade to a student, based on the average of his or her four test scores: Test Score Letter Grade 90–100 A 80-89 B 70-79 C 60-69 D 0-59 Write a class that uses a String array or an ArrayList object to hold the five students' names, an array of five characters to hold the five students' letter grades, and five arrays of four doubles each to hold each student's set of test scores. The class should have methods that return a specific student's name, the average test score, and a letter grade based on the average. Demonstrate the class in a program that allows the user to enter each student's name and his or her four test scores. It should then display each student's average test score and letter grade. Input Validation: Do not accept test scores less than zero or greater than 100.arrow_forward
- 1. Square Roots Create a class with a method that, given an integer, returns an array of double-precision floating point numbers (known in Java as double), each of which is a square root of a number between 2 and the parameter to the method. For example, if the parameter is 5, the method should return an array of 4 doubles, with approximate values 1.4142135623, 1.7320508075, 2.0, and 2.2360679774. Your method should check the validity of the parameter, and take appropriate action if the parameter is invalid. 2. Reading Files Create a class with a method that, given a string representing a file name, returns an integer with the number of characters in the file. For example, if the file has 10 characters, your method must return the number 10. 3. Main Class Create a class HW1.java with a main method that does both of the following: calls the method from part 1 with a random integer between 0 and 10, and prints each of the numbers in the result. The numbers should all be printed on the…arrow_forwardJAVA ARRAY MANIPULATION QUESTION: make a method called manipulator that takes a double array as parameter and modifies it. The method will modify the array then print the array. The method must modify the existing array, it CAN NOT create a different array as the solution. The manipulator method will be a void method. Remember: To get an average of 2 digits you add them together then divide by 2. Average of x and y = (x+y)/2 Modifications the method will do: For every 2 consecutive elements in the array, both the elements are replaced by their average. If the number of elements in an array is odd don't modify the last number of the array. Basically, you find the average of 2 consecutive elements then replace both of the elements with that value. Examples: Array = {2.0,3.0} will be changed to {2.5,2.5} when passed to the manipulator method Array = {2.0,3.0,45} will be changed to {2.5,2.5,45} when passed to the manipulator method Array = {2.0,3.0,45,55} will be changed to…arrow_forwardUsing oop in java Write code for PersonManagement class. This class contains the array of Person class (code is given below) and also following methods: set: This method receives the array of person class and set the attribute. displayAll: This method will display values of all persons. sort: This method will sort the array according to age values of the persons with ascending order using Selection Sort. reverse: This method will reverse the order of the array. getPersons: This method will return the array of the persons (attribute). displayEvenAgePerson: This method will display all those persons values who have even age. displayOddAgePerson: This method will display all those persons values who have odd age. displayPrimeAgePerson: This method will display only those persons values who have prime number age. Following is the class of Person: publicclass Person { private String Name; publicintage; publicvoid set(String Name, intage) {…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
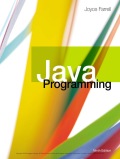
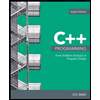
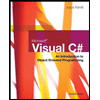