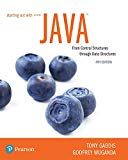
Two-dimensional array:
A two dimensional array is also called as a multi-dimensional array; a multidimensional array is that all the identical arrays are put together into a single array.
- This is useful for storing same type of multiple sets of data in same place.
- The main advantage is that one-dimensional array can hold only one set of value, whereas, two-dimensional array can hold multiple sets of data in the form of rows and columns.
- The structure of a multi-dimensional array is lookalike a table (that is combination of rows and columns); it contains same type of columns.
- The first size declarator in the two-dimensional array represents row and the second size declarator represents column.
- The two dimensional array can have multiple length fields, which holds the number of rows.
- Each row in the two dimensional array has a length field which holds the number of columns.
Syntax:
The syntax of two dimensional arrays is as follows:
datatype array_name [number_of_rows][number_of_columns];
From the given syntax, a two-dimensional array named “grades” of type “int” has been created with 30 rows and 10 columns.
Explanation of Solution
b. Statement that stores a number in last column of the last row of the array:
Syntax:
The syntax of two dimensional arrays is as follows:
datatype array_name [number_of_rows][number_of_columns];
Given array definition:
int[][] numberArray =new int[9][11];
There are 9 rows and 11 columns in the given two-dimensional array.
Index type of an array:
The index type of an array is an integer. An integer is a number without the fractional or decimal part...

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- Show all the workarrow_forwardShow all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forward
- Question 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forwardGenerate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forward
- I need help to solve the following, thank youarrow_forwardreminder it an exercice not a grading work GETTING STARTED Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website. Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”. If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically. With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet. If cell B6 does not display your name, delete the file and download a new copy from the SAM website. Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…arrow_forwardNeed help completing this algorithm here in coding! 2arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
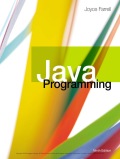
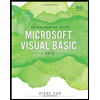
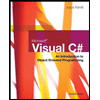
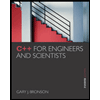
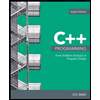