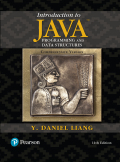
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134700144
Author: Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 6.8, Problem 6.8.3CP
Given two method definitions,
public static double m(double x, double y)
public static double m(int x, double y)
tell which of the two methods is invoked for:
- a. doub1e z = m (4, 5) ;
- b. double z = m(4, 5.4) ;
- c. double z = m(4.5, 5.4) ;
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Analyze the following code.
public class Test {
public static void main(String[] args){
System.out.printlin( m(2) );
public static int m( int num ) {
return num;
}
public static void m( int num ) {
System.out.printin( num );
}
The program has a compile error because the second m method is def
method.
The program runs and prints 2 once.
21. Consider the following method:
public static void sketch(int x1, int y1, int x2, int y2, int n)
(
if (n <= 0)
O
else
(
}
drawLine(x1, y1, x2, y2);
Assume that the screen looks like a Cartesian coordinate
system with the origin at the center, and that drawLine
connects (x1,y1) to (x2,y2). Assume also that x1, y1, x2,
and y2 are never too large or too small to cause errors.
Which picture best represents the sketch drawn by the
method call
int xm
(x1 + x2 + y1 - y2) / 2;
int ym (y1 + y2 + x2-x1) / 2;
sketch(x1, y1, xm, ym, n - 1);
sketch (xm, ym, x2, y2, n - 1);
sketch(a, 0, -a, 0, 2)
where a is a positive integer?
(A)
<-a
y
-a
y
a
X
(B)
(D)
-a
-a
y
+
-a
-a
a
Write a java program method that accepts a year as a parameter, the method then returns true or false if the entered year is a leap year or not.
You may calculate the leap year as follows:
1. If the year is evenly divisible by 4, go to step 2. Otherwise go to step 5.
2. If the year is evenly divisible by 100, go to step 3. Otherwise go to step 4.
3. If the year is evenly divisible by 400, go to step 4. Otherwise go to step 5.
4. The year is a leap year (it has 366 days).
5. The year is not a leap year (it has 366 days).
Chapter 6 Solutions
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
Ch. 6.4 - What are the benefits of using a method?Ch. 6.4 - Prob. 6.4.2CPCh. 6.4 - How do you simplify the max method in Listing 6.1...Ch. 6.4 - Prob. 6.4.4CPCh. 6.4 - Prob. 6.4.5CPCh. 6.4 - Prob. 6.4.6CPCh. 6.4 - Prob. 6.4.7CPCh. 6.4 - Write method headers (not the bodies) for the...Ch. 6.4 - Identify and correct the errors in the following...Ch. 6.4 - Prob. 6.4.10CP
Ch. 6.5 - Prob. 6.5.1CPCh. 6.5 - Identify and correct the errors in the following...Ch. 6.5 - Prob. 6.5.3CPCh. 6.5 - Prob. 6.5.4CPCh. 6.6 - Prob. 6.6.1CPCh. 6.6 - Prob. 6.6.2CPCh. 6.7 - What is hexCharToDecimal ( B)) ? What is...Ch. 6.8 - What is method overloading? Is it permissible to...Ch. 6.8 - What is wrong in the following program? public...Ch. 6.8 - Given two method definitions, public static double...Ch. 6.9 - Prob. 6.9.1CPCh. 6.9 - What is the scope of a local variable?Ch. 6 - (Math: pentagonal numbers) A pentagonal number is...Ch. 6 - (Sum the digits in an integer) Write a method that...Ch. 6 - (Palindrome integer) Write the methods with the...Ch. 6 - (Display an integer reversed) Write a method with...Ch. 6 - (Sort three numbers) Write a method with the...Ch. 6 - (Display patterns) Write a method to display a...Ch. 6 - (Financial application: compute the future...Ch. 6 - (Conversions between Celsius and Fahrenheit) Write...Ch. 6 - Prob. 6.9PECh. 6 - (Use the isPrime Method) Listing 6.7,...Ch. 6 - (Financial application: compute commissions) Write...Ch. 6 - (Display characters) Write a method that prints...Ch. 6 - (Sum series) Write a method to compute the...Ch. 6 - (Estimate ) can be computed using the following...Ch. 6 - (Financial application: print a tax table) Listing...Ch. 6 - Prob. 6.16PECh. 6 - Sections 6.10 and 6.11 6.17 (Display matrix of 0s...Ch. 6 - (Check password) Some Websites impose certain...Ch. 6 - (Triangles) Implement the following two methods: /...Ch. 6 - (Count the letters in a string) Write a method...Ch. 6 - (Phone keypads) The international standard...Ch. 6 - (Math: approximate the square root) There are...Ch. 6 - (Occurrences of a specified character) Write a...Ch. 6 - (Display current date and time) Listing 2.7,...Ch. 6 - Prob. 6.25PECh. 6 - (Palindromic prime) A palindromic prime is a prime...Ch. 6 - (Emirp) An emirp (prime spelled backward) is a...Ch. 6 - (Mersenne prime) A prime number is called a...Ch. 6 - (Twin primes) Twin primes are a pair of prime...Ch. 6 - (Game: craps) Craps is a popular dice game played...Ch. 6 - (Financial: credit card number validation) Credit...Ch. 6 - (Game: chance of winning at craps) Revise...Ch. 6 - (Current date and time) Invoking System....Ch. 6 - (Print calendar) Programming Exercise 3.21 uses...Ch. 6 - (Geometry: area of a pentagon) The area of a...Ch. 6 - (Geometry: area of a regular polygon) A regular...Ch. 6 - (Format an integer) Write a method with the...Ch. 6 - (Generate random characters) Use the methods in...Ch. 6 - (Geomentry: point position) Programming Exercise...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Find the no-load value of υo in the circuit shown.
Find υo when RL is 150 Ω.
How much power is dissipated in th...
Electric Circuits. (11th Edition)
The programmer intended the following pseudocode to display the numbers 1 through 60, and then display the mess...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
What is an algorithm?
Starting Out With Visual Basic (8th Edition)
State whether each of the following is true or false. If false, explain why. The remainder operator (%) can be ...
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
The following is an old word puzzle: Name a common word, besides tremendous, stupendous and horrendous, that en...
Problem Solving with C++ (10th Edition)
T F It is not necessary to initialize counter variables.
Starting Out with C++ from Control Structures to Objects (9th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Exercise 3: A. Write static methods: public static double sphereVolume(double r) :Tr3 public static double sphereSurface(double r) : 4ar2 public static double coneVolume(double r, double h): – thr² public static double coneSurface(double r, double h) :7r(h+r) 1 3 that compute the volume and surface area of a sphere with radius r, and a cone with circular base with radius r and height h. Place them into a class "Geometry2.java". Then write a program with main class called "Lab7_yourID.java" that prompts the user for the values of r and h, calls the four methods, and prints the results. B. (Optional) Reconsider your previous solution by implementing classes Sphere, and Cone separately instead of a single class "Geometry2" (part A). Which approach is more object-oriented? Extra Geometry to try: Area of Plane Shapes Area is the size of a surface! Learn more about Area, or try the Area Calculator. Triangle Area = 2 x b x h b = base h = vertical height Square Area = a? a = length of side a b…arrow_forward1. Write a program that takes three numbers (a,b,c) from the user as input and finds the median of the three. The program has a method called median that takes 3 numbers as the parameter and returns the median. Use if-then-else statements to find the median. You can assume the three numbers are not the same. Sample Example: Enter 3 numbers 10 13 The median is 10 public class Median{ public static void main(String[] args){ Scanner input = new int a = int b = int c =arrow_forwardConsider the following method: public static void mystery2(int n) { if (n > 100) { System.out.print(n); } else { mystery2(2 * n); System.out.print(", " + n); } } For each of the following calls, indicate the output that is produced by the method: a. mystery2(113); b. mystery2(70); c. mystery2(42); d. mystery2(30); e. mystery2(10);arrow_forward
- What is the correct method signature of the below function/methods public double myTotal(float x, int y, double z){ return (X + Y + Z); } Select one: a. public double myTotal(float x, int y, double z) b. myTotal(float, int, double) c. myTotal(int, double , float) d. myTotal(float x, int y, double z) CLEAR MY CHOICEarrow_forwarda Java program that defines and uses 3 overloaded methods (the Java file nameshould be “MethodOverload.java” and all the 3 methods should have the same methodname max). The 3 method headers are given below with their definitions:• int max(int n1, int n2, int n3):o to return the maximum of the passed 3 integer arguments.• double max(double d1, double d2, double d3):o to return the maximum of the passed 3 double arguments with use ofMath.max() method—that is, do not use if or if-else statement incomparing the numbers to find the smaller/smallest numbers.• int max(int n):In the main method of the application, a loop with switch-statement inside is required fortesting the different method calls and you need to generate random numbers for the inputsas the arguments for the methods (except for die-rolling case where an input is enteredfrom the keyboard.). Specifically:• int max(int n1, int n2, int n3): the 3 random integers should be from the sequence2, 5, 8, 11, 14.• double max(double d1,…arrow_forwardWrite the JAVA method for the following a): Window to View Conversion using equations:x_v= x_vmin+(x_w-x_wmin)s_x y_v= y_vmin+(y_w-y_wmin)s_y Wheres_x= (x_vmax-x_vmin)/(x_wmax-x_wmin )s_y= (y_vmax-y_vmin)/(y_wmax-y_wmin ) b): Point clipping, method will return Boolean (True/False) for the following conditions:xwmin ≤ x ≤ xwmaxywmin ≤ y ≤ ywmaxarrow_forward
- Java Scriptarrow_forwardwrite a c++ program Write a class Date that represents a date consisting of a year, month, and day. A Date classshould have the following methods:# Date(int year, int month, int day)Constructs a new Date object to represent the given date.# void add(int &days)Moves this Date object forward by the given number of days. hint:* you should decideon the basis of month and year that given month ends 30,31,28,29 days.# void add(int &month , int &days) Moves this Date object forward by the given number of months and days. Monthsshould be within 1 to 12 and days in 1 to 31. For Example Date 2003/12/31andadd(1,29) =>Date will be 2004/02/29# void add(Date & other)Moves this Date object forward by the given Date.# void addWeeks(int &weeks)Moves this Date object forward by the given number of seven-day weeks.# int daysTo(Date & other)Returns the number of days that this Date must be adjusted to make it equal to the givenother Date.# void subtract(int &days)Moves this…arrow_forwardExercise 3: A. Write static methods: • public static double sphereVolume(double r) :r3 • public static double sphereSurface(double r) : 47tr? • public static double coneVolume(double r, double h): – thr² public static double coneSurface(double r, double h) :7r(h +r) that compute the volume and surface area of a sphere with radius r, and a cone with circular base with radius r and height h. Place them into a class “Geometry2.java". Then write a program with main class called “Lab7_yourlD.java" that prompts the user for the values of r and h, calls the four methods, and prints the results.arrow_forward
- Write static methods* public static double cubeVolume(double h)* public static double cubeSurface(double h)* public static double sphereVolume(double h)* public static double sphereSurface(double h)* public static double cylinderVolume(double h)* public static double cylinderSurface(double h)that compute the volume and surface area of a cube, sphere, and cylinderPlace them in a class GeometryUse the given tester file to test your calculationsarrow_forward1. Write the following method that returns the intersecting point between two lines (p1, p2) and (p3, p4): public static Point 2D getIntersecting Point ( Point 2D pl, Point 2D p2, Point 2D p3, Point2D p4); The method returns null if the two lines are parallel. Write a test program that prompts the user to enter three points and displays the center point. Here is a sample run. (Hint: for finding the intersecting point between two lines, see Programming Exercise 8.31.) Enter x1, yl, x2, y2, x3, y3, x4, y4: 225 -1.0 4.0 2.0 -1.0 -2.0 The intersecting point is at (2.88889, 1.1111) The two lines are parallelarrow_forwardThe method half shown below, takes a variable of type int and returns the result of dividing the variable by 2. Write the overloaded method for half that accomplishes the same task for a variable of type double. public static int half(int val) { return val/2;}arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
Java Math Library; Author: Alex Lee;https://www.youtube.com/watch?v=ufegX5o8uc4;License: Standard YouTube License, CC-BY