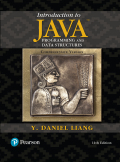
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134700144
Author: Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 6.4, Problem 6.4.10CP
Program Plan Intro
Block styles:
In
Example of next-line style:
public class Sample
{
public static void main(String[] args)
{
System.out.println("Sample");
}
}
Example of End-of-line style:
public class Sample{
public static void main(String[] args){
System.out.println("Sample");
}
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's
cssProperty value, and return the new "px" value.
Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px =
"200px".
SHOW EXPECTED
HTML JavaScript
1 function addPixels (element, cssProperty, pixelAmount) {
2
3
/* Your solution goes here *1
4 }
5
6 const helloElem = document.querySelector("# helloMessage");
7 const newVal = addPixels (helloElem, "width", 50);
8 helloElem.style.setProperty("width", newVal);
[
Solve in MATLAB
Hello please look at the attached picture. I need an detailed explanation of the architecture
Chapter 6 Solutions
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
Ch. 6.4 - What are the benefits of using a method?Ch. 6.4 - Prob. 6.4.2CPCh. 6.4 - How do you simplify the max method in Listing 6.1...Ch. 6.4 - Prob. 6.4.4CPCh. 6.4 - Prob. 6.4.5CPCh. 6.4 - Prob. 6.4.6CPCh. 6.4 - Prob. 6.4.7CPCh. 6.4 - Write method headers (not the bodies) for the...Ch. 6.4 - Identify and correct the errors in the following...Ch. 6.4 - Prob. 6.4.10CP
Ch. 6.5 - Prob. 6.5.1CPCh. 6.5 - Identify and correct the errors in the following...Ch. 6.5 - Prob. 6.5.3CPCh. 6.5 - Prob. 6.5.4CPCh. 6.6 - Prob. 6.6.1CPCh. 6.6 - Prob. 6.6.2CPCh. 6.7 - What is hexCharToDecimal ( B)) ? What is...Ch. 6.8 - What is method overloading? Is it permissible to...Ch. 6.8 - What is wrong in the following program? public...Ch. 6.8 - Given two method definitions, public static double...Ch. 6.9 - Prob. 6.9.1CPCh. 6.9 - What is the scope of a local variable?Ch. 6 - (Math: pentagonal numbers) A pentagonal number is...Ch. 6 - (Sum the digits in an integer) Write a method that...Ch. 6 - (Palindrome integer) Write the methods with the...Ch. 6 - (Display an integer reversed) Write a method with...Ch. 6 - (Sort three numbers) Write a method with the...Ch. 6 - (Display patterns) Write a method to display a...Ch. 6 - (Financial application: compute the future...Ch. 6 - (Conversions between Celsius and Fahrenheit) Write...Ch. 6 - Prob. 6.9PECh. 6 - (Use the isPrime Method) Listing 6.7,...Ch. 6 - (Financial application: compute commissions) Write...Ch. 6 - (Display characters) Write a method that prints...Ch. 6 - (Sum series) Write a method to compute the...Ch. 6 - (Estimate ) can be computed using the following...Ch. 6 - (Financial application: print a tax table) Listing...Ch. 6 - Prob. 6.16PECh. 6 - Sections 6.10 and 6.11 6.17 (Display matrix of 0s...Ch. 6 - (Check password) Some Websites impose certain...Ch. 6 - (Triangles) Implement the following two methods: /...Ch. 6 - (Count the letters in a string) Write a method...Ch. 6 - (Phone keypads) The international standard...Ch. 6 - (Math: approximate the square root) There are...Ch. 6 - (Occurrences of a specified character) Write a...Ch. 6 - (Display current date and time) Listing 2.7,...Ch. 6 - Prob. 6.25PECh. 6 - (Palindromic prime) A palindromic prime is a prime...Ch. 6 - (Emirp) An emirp (prime spelled backward) is a...Ch. 6 - (Mersenne prime) A prime number is called a...Ch. 6 - (Twin primes) Twin primes are a pair of prime...Ch. 6 - (Game: craps) Craps is a popular dice game played...Ch. 6 - (Financial: credit card number validation) Credit...Ch. 6 - (Game: chance of winning at craps) Revise...Ch. 6 - (Current date and time) Invoking System....Ch. 6 - (Print calendar) Programming Exercise 3.21 uses...Ch. 6 - (Geometry: area of a pentagon) The area of a...Ch. 6 - (Geometry: area of a regular polygon) A regular...Ch. 6 - (Format an integer) Write a method with the...Ch. 6 - (Generate random characters) Use the methods in...Ch. 6 - (Geomentry: point position) Programming Exercise...
Knowledge Booster
Similar questions
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
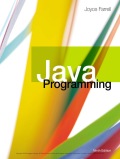
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
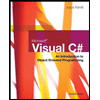
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
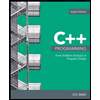
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
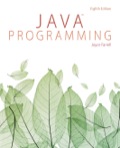
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
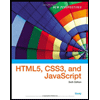
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning