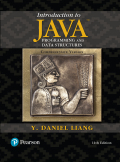
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134700144
Author: Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 6.6, Problem 6.6.2CP
Explanation of Solution
//Method definition "isPrime"
public static boolean isPrime(int number)
{
//For loop to check the condition
for (int divisor = 2; divisor <=number/2; divisor++)
{
/*Check whether "number" mod "divisor" equals to 0*/
if (number % divisor==0)
{
//Return false
return false;
}
}
//Return true
return true;
}
}
...Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write the SQL code that permits to implement the tables: Student and Transcript. NB: Add the constraints on the attributes – keys and other.
Draw an ERD that will involve the entity types: Professor, Student, Department and Course. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Draw an ERD that represents a book in a library system. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Chapter 6 Solutions
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
Ch. 6.4 - What are the benefits of using a method?Ch. 6.4 - Prob. 6.4.2CPCh. 6.4 - How do you simplify the max method in Listing 6.1...Ch. 6.4 - Prob. 6.4.4CPCh. 6.4 - Prob. 6.4.5CPCh. 6.4 - Prob. 6.4.6CPCh. 6.4 - Prob. 6.4.7CPCh. 6.4 - Write method headers (not the bodies) for the...Ch. 6.4 - Identify and correct the errors in the following...Ch. 6.4 - Prob. 6.4.10CP
Ch. 6.5 - Prob. 6.5.1CPCh. 6.5 - Identify and correct the errors in the following...Ch. 6.5 - Prob. 6.5.3CPCh. 6.5 - Prob. 6.5.4CPCh. 6.6 - Prob. 6.6.1CPCh. 6.6 - Prob. 6.6.2CPCh. 6.7 - What is hexCharToDecimal ( B)) ? What is...Ch. 6.8 - What is method overloading? Is it permissible to...Ch. 6.8 - What is wrong in the following program? public...Ch. 6.8 - Given two method definitions, public static double...Ch. 6.9 - Prob. 6.9.1CPCh. 6.9 - What is the scope of a local variable?Ch. 6 - (Math: pentagonal numbers) A pentagonal number is...Ch. 6 - (Sum the digits in an integer) Write a method that...Ch. 6 - (Palindrome integer) Write the methods with the...Ch. 6 - (Display an integer reversed) Write a method with...Ch. 6 - (Sort three numbers) Write a method with the...Ch. 6 - (Display patterns) Write a method to display a...Ch. 6 - (Financial application: compute the future...Ch. 6 - (Conversions between Celsius and Fahrenheit) Write...Ch. 6 - Prob. 6.9PECh. 6 - (Use the isPrime Method) Listing 6.7,...Ch. 6 - (Financial application: compute commissions) Write...Ch. 6 - (Display characters) Write a method that prints...Ch. 6 - (Sum series) Write a method to compute the...Ch. 6 - (Estimate ) can be computed using the following...Ch. 6 - (Financial application: print a tax table) Listing...Ch. 6 - Prob. 6.16PECh. 6 - Sections 6.10 and 6.11 6.17 (Display matrix of 0s...Ch. 6 - (Check password) Some Websites impose certain...Ch. 6 - (Triangles) Implement the following two methods: /...Ch. 6 - (Count the letters in a string) Write a method...Ch. 6 - (Phone keypads) The international standard...Ch. 6 - (Math: approximate the square root) There are...Ch. 6 - (Occurrences of a specified character) Write a...Ch. 6 - (Display current date and time) Listing 2.7,...Ch. 6 - Prob. 6.25PECh. 6 - (Palindromic prime) A palindromic prime is a prime...Ch. 6 - (Emirp) An emirp (prime spelled backward) is a...Ch. 6 - (Mersenne prime) A prime number is called a...Ch. 6 - (Twin primes) Twin primes are a pair of prime...Ch. 6 - (Game: craps) Craps is a popular dice game played...Ch. 6 - (Financial: credit card number validation) Credit...Ch. 6 - (Game: chance of winning at craps) Revise...Ch. 6 - (Current date and time) Invoking System....Ch. 6 - (Print calendar) Programming Exercise 3.21 uses...Ch. 6 - (Geometry: area of a pentagon) The area of a...Ch. 6 - (Geometry: area of a regular polygon) A regular...Ch. 6 - (Format an integer) Write a method with the...Ch. 6 - (Generate random characters) Use the methods in...Ch. 6 - (Geomentry: point position) Programming Exercise...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forward
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
- A Guide to SQLComputer ScienceISBN:9781111527273Author:Philip J. PrattPublisher:Course Technology PtrProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
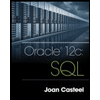
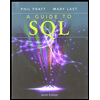
A Guide to SQL
Computer Science
ISBN:9781111527273
Author:Philip J. Pratt
Publisher:Course Technology Ptr
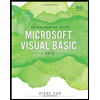
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning