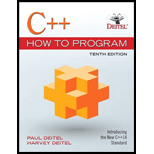
Program Plan:
The following variables and functions have been used in the program:
struct Stack : A structure to represent a stack with a specific capacity.
struct Stack* createStack(unsigned capacity) : function to create a stack of given capacity.
int isFull(struct Stack* stack) : function returns 1 when Stack is full when top is equal to the last index .
int isEmpty(struct Stack* stack) : function returns 1 when Stack is empty when top is equal to -1 .
void push(struct Stack *stack, int item) : adds an item to stack. It increases top by 1.
int pop(struct Stack* stack) : removes an item from stack. It decreases top by 1.
void moveDisk(char fromPeg, char toPeg, int disk) : Prints the movement of disks between the poles .
void moveDisksBetweenTwoPoles(struct Stack *src,struct Stack *dest, char s, char d) : implements the movement between the disks. It internally calls the function moveDisk() defined above.
void tohIterative(int num_of_disks, struct Stack *src, struct Stack *aux, struct Stack *dest) : Implements the Tower Of Hanoi puzzle by calling the moveDisksBetweenTwoPoles() function internally.
unsigned num_of_disks : stores the number of disks used in the puzzle, as entered by the user.

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
C++ How to Program (10th Edition)
- (GREATEST COMMON DIVISOR) The greatest common divisor of integers x and y is the largest integer that evenly divides into both x and y. Write and test a recursive function gcd that returns the greatest common divisor of x and y. The gcd of x and y is defined recursively as follows: If y is equal to 0, then gcd (x, y) is x; otherwise, gcd (x, y) is gcd (y, x % y), where % is the remainder operator.arrow_forward(Will report abuse if you just copy the previous answer) Write a tail recursive procedure in Scheme for the bang-bang operator: n!! Here 1!! is 1, 2!! = 2, and if n>2, n!!=(n−2)!!×n. Put this in the file bang-bang.scm.arrow_forwardIs the recursive solution to the triangle number the best way to obtain the triangle number? A. Yes, because recursive solutions are always efficient. B. Yes, because the shape of the triangle number diagram lends itself to a recursive solution. C. No, the triangle number can be found in a more efficient manner. D. None of the above are true.arrow_forward
- Please help me with this one. Just choose the letter with the correct answer. Thank you very much in advance :)arrow_forwardIdentify the base case in this recursive function. Assume i> 0 when the function is invoked. (Line numbers are not part of the code.) 1. def add(i, j): 2. if i == 0: 3. return j 4. else: 5. return add(i - 1, j + 1) This function has no base case O line 5 line 1 line 4 O line 2 Question 6 The following recursive function is supposed to return a list that is the reverse of the list it was given. But it has a bug. What is the bug? (Line numbers are not part of the code.) MooP eok Drarrow_forward(Recursive Greatest Common Divisor) The greatest common divisor of integers x and y isthe largest integer that evenly divides both x and y. Write a recursive function gcd that returns thegreatest common divisor of x and y. The gcd of x and y is defined recursively as follows: If y is equalto 0, then gcd(x, y) is x; otherwise gcd(x, y) is gcd(y, x % y), where % is the remainder operator.arrow_forward
- (Recursion and Backtracking) Write the pseudo code for a recursive method called addB2D that takes two binary numbers as strings, adds the numbers and returns the result as a decimal integer. For example, addB2D(‘‘101’’, ‘‘11’’) should return 8 (because the strings are binary representation of 5 and 3, respectively). Your solution should not just simply convert the binary numbers to decimal numbers and add the re- sults. An acceptable solution tries to find the sum by just directly processing the binary representations without at first converting them to decimal values.arrow_forwardPlease provide step by step solution. And provide screen shoot of running code Thank youarrow_forwardPlease can be handwritten. Question 2: Implementing a Recursive Function . Write recursive function, recursionprob(n), which takes a positive number as its argument and returns the output as shown below. The solution should clearly write the steps as shown in an example in slide number 59 and slide number 60 in lecture slides. After writing the steps, trace the function for “recursiveprob(5)” as shown in an example slide number 61. Function Output: >> recursionprob(1) 1 >> recursionprob(2) 1 4 >> recursionprob(3) 1 4 9 >>recrusionprob(4) 1 4 9 16arrow_forward
- Using Python Recursion is the concept of a function calling itself until the problem is solved when the Base Case is met. Study Recursion: See slides in Modules, Practice Slides, lab12 and also Recursion is in chapter 9 of the textbook. Some examples of recursion are: compute [ factorial of a number, towers of Hanoi, fractals (as shown in the textbook), and many more]. Example: See slides Page 2 For this assignment we'll use Collatz Cojecture (see Wikipedia). Collatz Conjecture algorithm: Given a number n, first call to the function: f(n): In the function: if n == 1 return 1: if n is even then f(n/2), i.e. call self with the new value. else (n is odd) call self with the new value, f((n*3)+1) and repeat. All numbers eventually end up with 1. The program should test for the base case which is: if n == 1, in which case it returns to the caller with 1. This problem is perfect to demonstrate Recursion.Create a list with random numbers (you can just do this part manually) in the list as 1,…arrow_forward2, Towers of Hanoi Problem. (10 points) The Towers of Hanoi is a famous problem for studying recursion in computer science and searching in artificial intelligence. We start with N discs of varying sizes on a peg (stacked in order according to size), and two empty pegs. We are allowed to move a disc from one peg to another, but we are never allowed to move a larger disc on top of a smaller disc. The goal is to move all the discs to the rightmost peg (see figure). To solve the problem by using search methods, we need first formulate the problem. Supposing there are K pegs and N disk. Answer the following questions. (1) Determine a state representation for this problem. (4points) (2) What is the size of the state space? (3 points) (3) Supposing K=3, N=4, what is the start state by using your proposed state representation method and what is the goal state? (3 points)arrow_forwardEx. 01 : Recursion About So far, we have learned that we can perform repetitive tasks using loops. However, another way is by creating methods that call themselves. This programming technique is called recursion and, a method that calls itself within it's own body is called a recursive method. One use of recursion is to perform repetitive tasks instead of using loops, since some problems seem to be solved more naturally with recursion than with loops. To solve a problem using recursion, it is broken down into sub-problems. Each sub-problem is similar to the original problem, but smaller in size. You can apply the same approach to each sub-problem to solve it recursively. All recursive methods use conditional tests to either 1. stop or 2. continue the recursion. Each recursive method has the following characteristics: 1. end/terminating case: One or more end cases to stop the recursion. 2. recursive case: reduces the problem in to smaller sub-problems, until it reaches (becomes) the end…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
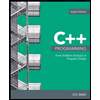