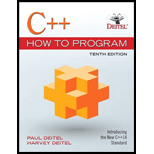
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 6, Problem 6.36E
Program Plan Intro
- In the program, we include the header files as needed.
- Function prototype will be declared.
- Declaring main() function as integer type.
- Variable declaration: declaring variablesuser_base_num, user_exponent_num as integer type which will take input from user.
- Calling the recursion_power_cal() in the main().
- recursion_power_cal() this function will help to calculate the recursive function of power.
- declaring the variable carry_base, carry_exponent as integer in recursion_power_cal() function definition as integer type which will carry the user input to the function to calculate the result.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
(GREATEST COMMON DIVISOR) The greatest common divisor of integers
x and y is the largest integer that evenly divides into both x and y. Write and
test a recursive function gcd that returns the greatest common divisor of x
and y. The gcd of x and y is defined recursively as follows: If y is equal to 0,
then gcd (x, y) is x; otherwise, gcd (x, y) is gcd (y, x % y), where % is the
remainder operator.
(C Language) Write a recursive function called DigitCount() that takes a non-negative integer as a parameter and returns the number of digits in the integer. Hint: The digit count increases by 1 whenever the input number is divided by 10.
lint power (int base, int exponent);
Define the recursive function power() that when invoked returns
baseonent
Assume that exponent is an integer greater than or equal to 1. Hint: The recursion step would use
the relationship
basenent = base * baseonert-
and the terminating condition occurs when exponent is equal to 1 because
base' = base
or when exponent is equal to o because
base = 1.
Chapter 6 Solutions
C++ How to Program (10th Edition)
Ch. 6 - Show the value of x after each of the following...Ch. 6 - (Parking Charges) A parking garage charges a...Ch. 6 - Prob. 6.13ECh. 6 - (Rounding Numbers) Function floor can be used to...Ch. 6 - Prob. 6.15ECh. 6 - (Random Numbers) Write statement that assign...Ch. 6 - (Random Numbers) Write a single statement that...Ch. 6 - Prob. 6.18ECh. 6 - Prob. 6.19ECh. 6 - Prob. 6.20E
Ch. 6 - Prob. 6.21ECh. 6 - Prob. 6.22ECh. 6 - Prob. 6.23ECh. 6 - (Separating Digits) Write program segments that...Ch. 6 - (Calculating Number of Seconds) Write a function...Ch. 6 - (Celsius and Fahrenheit Temperature) Implement the...Ch. 6 - (Find the Minimum) Write a program that inputs...Ch. 6 - Prob. 6.28ECh. 6 - (Prime Numbers) An integer is said to be prime if...Ch. 6 - Prob. 6.30ECh. 6 - Prob. 6.31ECh. 6 - (Quality Points for Numeric Grades) Write a...Ch. 6 - Prob. 6.33ECh. 6 - (Guess-the-Number Game) Write a program that plays...Ch. 6 - (Guess-the-Number Game Modification) Modify the...Ch. 6 - Prob. 6.36ECh. 6 - Prob. 6.37ECh. 6 - Prob. 6.38ECh. 6 - Prob. 6.39ECh. 6 - Prob. 6.40ECh. 6 - Prob. 6.41ECh. 6 - Prob. 6.42ECh. 6 - Prob. 6.43ECh. 6 - Prob. 6.44ECh. 6 - (Math Library Functions) Write a program that...Ch. 6 - (Find the Error) Find the error in each of the...Ch. 6 - (Craps Game Modification) Modify the craps program...Ch. 6 - (Circle Area) Write a C++ program that prompts the...Ch. 6 - (pass-by-Value vs. Pass-by-Reference) Write a...Ch. 6 - (Unary Scope Resolution Operator) What’s the...Ch. 6 - (Function Templateminimum) Write a program that...Ch. 6 - Prob. 6.52ECh. 6 - (Find the Error) Determine whether the following...Ch. 6 - (C++ Random Numbers: Modified Craps Game) Modify...Ch. 6 - (C++ Scoped enum) Create a scoped enum named...Ch. 6 - (Function Prototype and Definitions) Explain the...Ch. 6 - Prob. 6.57MADCh. 6 - Prob. 6.58MADCh. 6 - (Computer-Assisted Instruction: Monitoring Student...Ch. 6 - (Computer-Assisted Instruction: Difficulty Levels)...Ch. 6 - (Computer-Assisted Instruction: Varying the Types...
Knowledge Booster
Similar questions
- Identify the base case in this recursive function. Assume i> 0 when the function is invoked. (Line numbers are not part of the code.) 1. def add(i, j): 2. if i == 0: 3. return j 4. else: 5. return add(i - 1, j + 1) This function has no base case O line 5 line 1 line 4 O line 2 Question 6 The following recursive function is supposed to return a list that is the reverse of the list it was given. But it has a bug. What is the bug? (Line numbers are not part of the code.) MooP eok Drarrow_forwardProblem 1: Recursion to Generator You worked with these last homework. For this homework, you'll write the tail recursion, while, and generator. This is a reminder that in the starter code, we are providing you with the regular recursion code, and the functions you must implement must utilize tail recursion, a while loop, or a generator as specified. In your starter code, function names will end in _t, _w, or _g if the function needs to be implemented using tail recursion, a while loop, or a generator, respectively. Review the lecture slides to learn more about generators. p(0) p(n) = 10000 = p(n-1) + 0.02p(n − 1) c(1) = 9 c(n) = 9c(n-1) + 10-1 — c(n − 1) d(0) = 1 d(n) = 3d(n-1) + 1 Programming Problem 1: Recursion to Generators • For reach function you'll write the tail recursive form, while, and generator. • We have added the signature to help you-in particular, c(n) requres two accumula- tors. (1) (2) (3) (4) (5) (6) (7)arrow_forward(Towers of Hanoi: Iterative Solution) Any program that can be implemented recursivelycan be implemented iteratively, although sometimes with considerably more difficulty and considerably less clarity. Try writing an iterative version of the Towers of Hanoi. If you succeed, compareyour iterative version with the recursive version you developed in Exercise 5.36. Investigate issuesof performance, clarity, and your ability to demonstrate the correctness of the programs.arrow_forward
- Objective: Practice writing recursive functions in python3 Make the five recursive functions described below in python3 by using the starter code recursive_functions.py. For each function, figure out how to solve it conceptually : write down the base case (when recursion stops) and how each recursive function-call moves towards the base case. The functions should not print anything (except you may add temporary print statements to help debug them). You can test your program by using the provided program test_recursive_functions.py. Don't edit the test program. Put it into the same directory (folder) with your recursive_functions.py and run it. It will import your functions as a module, test your functions, and tell you when each function is returning correct results. 1. Factorial In math, if you have a number n, the factorial function (written n!) computes n x (n-1) x (n-2) x (n-3) x ... x 1. For example: 0! is defined to be 1 1! = 1 2! = 2 x 1=2 3! = 3 x 2 x 1=6 4! = 4 x 3…arrow_forwardFor glass box testing of a recursive function, you should test cases where: a) the function returns without a recursive call, ie using a base case b) the function makes exactly one recursive call c) the function makes more than one recursive call d) all of thesearrow_forward6. Write a recursive function that find the sum of the following series. 1+1/2+1/4+1/8+...+1/2"arrow_forward
- Python program (recursive function) A recursive function is a function defined in terms of itself via self-referential expressions. This means that the function will continue to call itself and repeat its behavior until some condition is met to return a result. Write a python recursive functionprod that takes x as an argument, and returns the result where, result=1*1/2*1/3*….*1/n Include a screenshot that shows a python program that uses the above function and prints the rounded result to three decimal placesafter prompting the user to enter a number, x. Use x=3. N.B: The code should be included please.arrow_forward(Replace strings) Write the following function that replaces the occurrence of a substring old_substring with a new substring new_substring in the string s. The function returns true if string s is changed, and otherwise, it returns false. bool replace_strings (string& s, const string& old_string, const string& new_string) Write a test program that prompts the user to enter three strings, i.e., s, old string, and new_string, and display the replaced string.arrow_forwardMULTIPLE FUNCTIONS AND RECURSIVE FUNCTIONS.arrow_forward
- (Please provide photos so the code is easier to understand) Write a small C++ program that defines a recursive function GCD based on the model of the Lisp function (defun gcd (n m)"Returns the gcd of two numbers" (let ((dividend n) (divisor m) (remainder 1)) (while (/= remainder 0) (setq remainder (mod dividend divisor)) (setq dividend divisor) (setq divisor remainder)) dividend)) In the main function, write a loop (while or do) that repeats the following actions: ask the user to enter two numbers, compute their GCD and output it, until the user enters 0 for one of the numbers.arrow_forward*C Language The greatest common divisor of integers x and y is the largest integer that divides both x and y. Write a recursive function GCD that returns the greatest common divisor of x and y. The GCD of x and y is defined as follows: If y is equal to zero, then GCD(x, y) is x; otherwise GCD(x, y) is GCD(y, x % y) where % is the remainder operator.arrow_forwardExponent y Catherine Arellano mplement a recursive function that returns he exponent given the base and the result. for example, if the base is 2 and the result is 3, then the output should be 3 because the exponent needed for 2 to become 8 is 3 (i.e. 23 = 8) nstructions: 1. In the code editor, you are provided with a main() function that asks the user for two integer inputs: 1. The first integer is the base 2. The second integer is the result 2. Furthermore, you are provided with the getExponent() function. The details of this function are the following: 1. Return type - int 2. Name - getExponent 3. Parameters 1. int - base 2. int - result 4. Description - this recursive function returns the exponent 5. Your task is to add the base case and the general case so it will work Score: 0/5 Overview 1080 main.c exponent.h 1 #include 2 #include "exponent.h" 3 int main(void) { 4 int base, result; 5 6 printf("Enter the base: "); scanf("%d", &base); 7 8 9 printf("Enter the result: ");…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
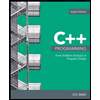
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning