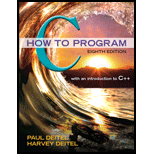
C How to Program (8th Edition)
8th Edition
ISBN: 9780133976892
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 6, Problem 6.35RE
Program Plan Intro
- Include the header files and initialize the main() function.
- Prompt the user to take the input of integer array.
- To define the recursive function printArray (int [], int) that takes integer array as input and its size to print the array.
- Call the function to display the output.
- Define the function role in the respective function definition.
Summary Introduction- The program prints the array using recursive function.
Program Description- The purpose of the program is to take the integer array as input and print it using the following recursive function and returns nothing.
voidprintArray (int [], int)
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
(Recursive Greatest Common Divisor) The greatest common divisor of integers x and y isthe largest integer that evenly divides both x and y. Write a recursive function gcd that returns thegreatest common divisor of x and y. The gcd of x and y is defined recursively as follows: If y is equalto 0, then gcd(x, y) is x; otherwise gcd(x, y) is gcd(y, x % y), where % is the remainder operator.
Question 4: (Find the minimum value in an array) Write a program that include a
recursive function "recursiveMinimnm" that takes an integer array and the array size as
arguments and returns the smallest element of the array. The function should stop
processing and return when it receives an array of one element.
Answer
(2.5)
[In c#]
Write a class with name Arrays . This class has an array which should be initialized by user.Write a method Sum that should sum even numbers in array and return sum. write a function with name numFind in this class with working logic as to find the mid number of an array. After finding this number calculate its factorial.Write function that should display sum and factorial.Don’t use divide operator
Chapter 6 Solutions
C How to Program (8th Edition)
Ch. 6 - Fill in the blanks in each of the following: C...Ch. 6 - State which of the following are true and which...Ch. 6 - Write statements to accomplish each of the...Ch. 6 - Consider a 2-by-5 integer array t. Write a...Ch. 6 - (Sales Commissions) Use a one-dimensional array to...Ch. 6 - (Bubble Sort) The bubble sort presented in Fig....Ch. 6 - Write loops that perform each of the following...Ch. 6 - Prob. 6.13ECh. 6 - (Mean, Median and Mode Program Modifications)...Ch. 6 - (Duplicate Elimination) Use a one-dimensional...
Ch. 6 - Label the elements of 3-by-5 two-dimensional array...Ch. 6 - What does the following program do?Ch. 6 - What does the following program do?Ch. 6 - (Dice Rolling) Write a program that simulates the...Ch. 6 - (Game of Craps) Write a program that runs 1000...Ch. 6 - Prob. 6.21ECh. 6 - (Total Sales) Use a two-dimensional array to solve...Ch. 6 - (Turtle Graphics) The Logo language made the...Ch. 6 - Prob. 6.24ECh. 6 - (Knights Tour: Brute-Force Approaches) In Exercise...Ch. 6 - (Eight Queens) Another puzzler for chess buffs is...Ch. 6 - (Eight Queens: Brute-Force Approaches) In this...Ch. 6 - (Duplicate Elimination) In Chapter 12, we explore...Ch. 6 - (Knights Tour: Closed Tour Test) In the Knights...Ch. 6 - (The Sieve of Eratosthenes) A prime integer is any...Ch. 6 - Prob. 6.31RECh. 6 - (Linear Search) Modify the program of Fig. 6.18 to...Ch. 6 - (Binary Search) Modify the program of Fig. 6.19 to...Ch. 6 - Prob. 6.35RECh. 6 - Prob. 6.36RECh. 6 - Prob. 6.37RE
Knowledge Booster
Similar questions
- (Recursive Binary Search) Write a recursive method RecursiveBinarySearch to perform abinary search of the array. The method should receive the search key, starting index, endingindex and array A as arguments. If the search key is found, return its index in the array. If thesearch key is not found, return -1.int RecursiveBinarySearch(int search, int start, int end, int[] A)arrow_forward(Check test scores) The answers to a true-false test are as follows: T T F F T. Given a two-dimensional answer array, in which each row corresponds to the answers provided on one test, write a function that accepts the two-dimensional array and number of tests as parameters and returns a one-dimensional array containing the grades for each test. (Each question is worth 5 points so that the maximum possible grade is 25.) Test your function with the following data: int score = 0;arrow_forward(find the minimum valuein an array)write a programthat include a recrusive function "recrusiveMinimum" that takes an integer array and the array size as arguments an return the smallest element of the arraythe function should stop processing and return when it receivesan array of one elementarrow_forward
- (Towers of Hanoi: Iterative Solution) Any program that can be implemented recursivelycan be implemented iteratively, although sometimes with considerably more difficulty and considerably less clarity. Try writing an iterative version of the Towers of Hanoi. If you succeed, compareyour iterative version with the recursive version you developed in Exercise 5.36. Investigate issuesof performance, clarity, and your ability to demonstrate the correctness of the programs.arrow_forwardstate the statement either true or false.arrow_forward(Recursion and Backtracking) Write the pseudo code for a recursive method called addB2D that takes two binary numbers as strings, adds the numbers and returns the result as a decimal integer. For example, addB2D(‘‘101’’, ‘‘11’’) should return 8 (because the strings are binary representation of 5 and 3, respectively). Your solution should not just simply convert the binary numbers to decimal numbers and add the re- sults. An acceptable solution tries to find the sum by just directly processing the binary representations without at first converting them to decimal values.arrow_forward
- Question 4: (Find the minimum value in an array) Write a program that include arecursive function “recursiveMinimum” that takes an integer array and the array size asarguments and returns the smallest element of the array. The function should stopprocessing and return when it receives an array of one element.arrow_forward4. CodeW X For func X C Solved b Answer x+ https://codeworkou... ... [+) CodeWorkout X271: Recursion Programming Exercises: Minimum of array For function recursiveMin, write the missing part of the recursive call. This function should return the minimum element in an array of integers. You should assume that recursiveMin is initially called with startIndex = 0. Examples: recursiveMin({2, 4, 8}, 0) -> 2 Your Answer: 1 public int recursiveMin(int numbers[], int startIndex) { numbers.length - 1) { if (startIndex 2. return numbers[startIndex]; } else { return Math. min(numbers[startIndex], >); 5. { 1:11 AM 50°F Clear 12/4/2021arrow_forward(Airplane Seating Assignment) | Write a program that can be used to assign seats for a commercial airplane. The airplane has 13 rows, with six seats in each row. Rows 1 and 2 are first class, rows 3 through 7 are business class, and rows 8 through 13 are economy class. Use two parallel arrays: a one-dimensional array to store the row number of the seats (Row #) a two-dimensional array of 13 rows and 6 columns to store the seat assignments (*) and seat letters (A-F) Your program must prompt the user to enter the following information: Reserve a seat (Yes (Y/y) or No (N/n)) Assign ticket type (first class (F/f), business class (B/b), or economy class (E/e)) Select desired seat (1-13 and A-F) Your program must contain at least the following functions: a function to initialize the seat plan. a function to show the seat assignments. a function to show the menu to assign a seat. a function to assign and select your desired seat. a function for each ticket type that determines if a seat…arrow_forward
- (Airplane Seating Assignment) | Write a program that can be used to assign seats for a commercial airplane. The airplane has 13 rows, with six seats in each row. Rows 1 and 2 are first class, rows 3 through 7 are business class, and rows 8 through 13 are economy class. Use two parallel arrays: a one-dimensional array to store the row number of the seats (Row #) a two-dimensional array of 13 rows and 6 columns to store the seat assignments (*) and seat letters (A-F) Your program must prompt the user to enter the following information: Reserve a seat (Yes (Y/y) or No (N/n)) Assign ticket type (first class (F/f), business class (B/b), or economy class (E/e)) Select desired seat (1-13 and A-F) Your program must contain at least the following functions: a function to initialize the seat plan. a function to show the seat assignments. a function to show the menu to assign a seat. a function to assign and select your desired seat. a function for each ticket type that determines if a seat…arrow_forward(Airplane Seating Assignment) | Write a program that can be used to assign seats for a commercial airplane. The airplane has 13 rows, with six seats in each row. Rows 1 and 2 are first class, rows 3 through 7 are business class, and rows 8 through 13 are economy class. Use two parallel arrays: a one-dimensional array to store the row number of the seats (Row #) a two-dimensional array of 13 rows and 6 columns to store the seat assignments (*) and seat letters (A-F) Your program must prompt the user to enter the following information: Reserve a seat (Yes (Y/y) or No (N/n)) Assign ticket type (first class (F/f), business class (B/b), or economy class (E/e)) Select desired seat (1-13 and A-F) Your program must contain at least the following functions: a function to initialize the seat plan. a function to show the seat assignments. a function to show the menu to assign a seat. a function to assign and select your desired seat. a function for each ticket type that determines if a seat…arrow_forwardIn java there must be at least two calls to the function with different arguments and the output must clearly show the task being performed. (ONLY ARRAYS or ARRAYLIST) Develop a function that accepts an array and returns true if the array contains any duplicate values or false if none of the values are repeated. Develop a function that returns true if the elements are in decreasing order and false otherwise. A “peak” is a value in an array that is preceded and followed by a strictly lower value. For example, in the array {2, 12, 9, 8, 5, 7, 3, 9} the values 12 and 7 are peaks. Develop a function that returns the number of peaks in an array of integers. Note that the first element does not have a preceding element and the last element is not followed by anything, so neither the first nor last elements can be peaks. Develop a function that finds the starting index of the longest subsequence of values that is strictly increasing. For example, given the array {12, 3, 7, 5, 9, 8,…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
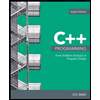
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning