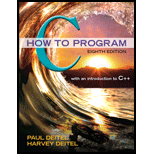
C How to Program (8th Edition)
8th Edition
ISBN: 9780133976892
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 6, Problem 6.11E
(Bubble Sort) The bubble sort presented in Fig. 6.15 is inefficient for large arrays. Make the following simple modifications to improve its performance.
- After the first pass, the largest number is guaranteed to be ¡n the highest-numbered element of the array; after the second pass, the two highest numbers are “in place,” and so on. Instead of making nine comparisons on every pass, modify the bubble sort to make eight comparisons on the second pass, seven on the third pass and so on.
- The data in the array may already be in the proper or near-proper order, so why make nine passes if fewer will suffice? Modify the sort to check at the end of each pass whether any swaps have been made. If none has been made, then the data must already be in the proper order, so the
program should terminate. If swaps have been made, then at least one more pass is needed.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
This battle room is focused on entry level tasks for a network analyst where you will be given trials and reconnaissance, sensor tuning, log aggregation, SIEM queries, and network analysis.
For this week’s project, complete the following tasks:
From your Project Ares portal, LOG IN
Click on LAUNCH GAME.
Select the region NORTH AMERICA
Click on Battle School
Under the BATTLE SCHOOL pop-up window, click on START TRAINING.
Under the BATTLE ROOMS tile, click on ENTER.
Under the NETWORK ANALYST tile, click on PLAY.
Wait for the Battle Room to load. While loading, the BATTLE ROOM button will display red. Once the Battle Room is loaded, the BATTLE ROOM button will turn yellow and the center of the disk display will indicate CONNECTED. Click on the BATTLE ROOM button to enter the Battle Room.
Below the TASKS folder, make sure you click on INSTRUCTIONS to download the Network Analyst Fundamentals material.
In the Battle Room, under the TASKS menu select task INTRUSION DETECTION.
Complete…
Create a relationship between the common field (Technician Number) of the two tables. Make sure that each client must have 1 and only 1 technician assigned, and each technician can have multiple clients.
2. Create a query to show the Client Number, Client Name, Billed, Paid for clients in Anderson city. Save the query.
3. Create a query to show the Technician Number, Last Name, First Name, YTD Earnings for technicians whose Hourly Rate is greater than or equal to 30. Save the query.
4. Create a query to show Client Number, Client Name, Billed, Paid for clients whose technician number is 22 and whose Billed is over 300. Save the query.
5. Create a query to show the Technician Number, Last Name, First Name, Client Number, Client Name for clients whose technician number 23. Save the query.
6. Create a query to show the Technician Number, Last Name, First Name, Client Number, Client Name for clients whose technician number 23 or 29. Save the query Help please Microsoft office access
Dijkstra's Algorithm (part 1). Consider the network shown below, and Dijkstra’s link-state algorithm. Here, we are interested in computing the least cost path from node E (note: the start node here is E) to all other nodes using Dijkstra's algorithm. Using the algorithm statement used in the textbook and its visual representation, complete the "Step 0" row in the table below showing the link state algorithm’s execution by matching the table entries (i), (ii), (iii), and (iv) with their values. Write down your final [correct] answer, as you‘ll need it for the next question.
Chapter 6 Solutions
C How to Program (8th Edition)
Ch. 6 - Fill in the blanks in each of the following: C...Ch. 6 - State which of the following are true and which...Ch. 6 - Write statements to accomplish each of the...Ch. 6 - Consider a 2-by-5 integer array t. Write a...Ch. 6 - (Sales Commissions) Use a one-dimensional array to...Ch. 6 - (Bubble Sort) The bubble sort presented in Fig....Ch. 6 - Write loops that perform each of the following...Ch. 6 - Prob. 6.13ECh. 6 - (Mean, Median and Mode Program Modifications)...Ch. 6 - (Duplicate Elimination) Use a one-dimensional...
Ch. 6 - Label the elements of 3-by-5 two-dimensional array...Ch. 6 - What does the following program do?Ch. 6 - What does the following program do?Ch. 6 - (Dice Rolling) Write a program that simulates the...Ch. 6 - (Game of Craps) Write a program that runs 1000...Ch. 6 - Prob. 6.21ECh. 6 - (Total Sales) Use a two-dimensional array to solve...Ch. 6 - (Turtle Graphics) The Logo language made the...Ch. 6 - Prob. 6.24ECh. 6 - (Knights Tour: Brute-Force Approaches) In Exercise...Ch. 6 - (Eight Queens) Another puzzler for chess buffs is...Ch. 6 - (Eight Queens: Brute-Force Approaches) In this...Ch. 6 - (Duplicate Elimination) In Chapter 12, we explore...Ch. 6 - (Knights Tour: Closed Tour Test) In the Knights...Ch. 6 - (The Sieve of Eratosthenes) A prime integer is any...Ch. 6 - Prob. 6.31RECh. 6 - (Linear Search) Modify the program of Fig. 6.18 to...Ch. 6 - (Binary Search) Modify the program of Fig. 6.19 to...Ch. 6 - Prob. 6.35RECh. 6 - Prob. 6.36RECh. 6 - Prob. 6.37RE
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Suppose that both a program and the hardware that executes it have been formally verified to be accurate. Does ...
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
TestScores Modification for Serialization Modify the TestScores class that you created for Programming Challeng...
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Find the no-load value of υo in the circuit shown.
Find υo when RL is 150 Ω.
How much power is dissipated in th...
Electric Circuits. (11th Edition)
Before the uniform distributed load is applied to the beam, there is a small gap of 0.2 mm between the beam and...
Mechanics of Materials (10th Edition)
1.2 Explain the difference between geodetic and plane
surveys,
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Why might some form of protective atmosphere be required when using a laser and powdered material?
Degarmo's Materials And Processes In Manufacturing
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
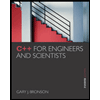
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
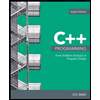
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
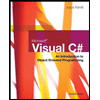
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
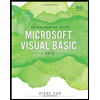
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
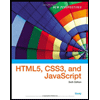
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
1.1 Arrays in Data Structure | Declaration, Initialization, Memory representation; Author: Jenny's lectures CS/IT NET&JRF;https://www.youtube.com/watch?v=AT14lCXuMKI;License: Standard YouTube License, CC-BY
Definition of Array; Author: Neso Academy;https://www.youtube.com/watch?v=55l-aZ7_F24;License: Standard Youtube License