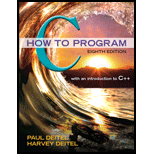
C How to Program (8th Edition)
8th Edition
ISBN: 9780133976892
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 6, Problem 6.25E
(Knight’s Tour: Brute-Force Approaches) In Exercise 6.24 we developed a solution to the Knights Tour problem. The approach used, called the ‘accessibility heuristic, generates many solutions and executes efficiently.
As computers continue increasing in power, we’ll be able to solve many problems with sheer computer power and relatively unsophisticated algorithms. Let’s call this approach brute-force problem solving.
- Use random number generation to enable the knight to walk around the chess board (in its legitimate L-shaped moves, of course) at random. Your
program should run one tour and print the final chessboard. How far did the knight get? - Most likely, the preceding program produced a relatively short tour. Now modify your program to attempt 1,000 tours. Use a one-dimensional array to keep track of the number of tours of each length. When your program finishes attempting the 1000 tours, it should print this information in a tabular format. What was the best result?
- Most likely, the preceding program gave you some “respectable tours but no full tours. Now “pull all the stops out” and simply let your program run until it produces a full tour. [Caution: This version of the program could run for hours on a powerful computer.] Once again, keep a table of the number of tours of each length and print this table when the first full tour is found. How many tours did your program attempt before producing a full tour? How much time did it take?
- Compare the brute-force version of the Knight’s Tour with the accessibility-heuristic version. Which required a more careful study of the problem? Which
algorithm was more difficult to develop? Which required more computer power? Could we be certain (in advance) of obtaining a full tour with the accessibility-heuristic approach? Could we be certain (in advance) of obtaining a full tour with the brute-force approach? Argue the pros and cons of brute-force problem solving in general.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Dijkstra's Algorithm (part 1). Consider the network shown below, and Dijkstra’s link-state algorithm. Here, we are interested in computing the least cost path from node E (note: the start node here is E) to all other nodes using Dijkstra's algorithm. Using the algorithm statement used in the textbook and its visual representation, complete the "Step 0" row in the table below showing the link state algorithm’s execution by matching the table entries (i), (ii), (iii), and (iv) with their values. Write down your final [correct] answer, as you‘ll need it for the next question.
4. |z + 5 - 5i| = 7
14.
dz,
C: |z❘
C: |z❘ = 0.6
ze² - 2iz
H
Chapter 6 Solutions
C How to Program (8th Edition)
Ch. 6 - Fill in the blanks in each of the following: C...Ch. 6 - State which of the following are true and which...Ch. 6 - Write statements to accomplish each of the...Ch. 6 - Consider a 2-by-5 integer array t. Write a...Ch. 6 - (Sales Commissions) Use a one-dimensional array to...Ch. 6 - (Bubble Sort) The bubble sort presented in Fig....Ch. 6 - Write loops that perform each of the following...Ch. 6 - Prob. 6.13ECh. 6 - (Mean, Median and Mode Program Modifications)...Ch. 6 - (Duplicate Elimination) Use a one-dimensional...
Ch. 6 - Label the elements of 3-by-5 two-dimensional array...Ch. 6 - What does the following program do?Ch. 6 - What does the following program do?Ch. 6 - (Dice Rolling) Write a program that simulates the...Ch. 6 - (Game of Craps) Write a program that runs 1000...Ch. 6 - Prob. 6.21ECh. 6 - (Total Sales) Use a two-dimensional array to solve...Ch. 6 - (Turtle Graphics) The Logo language made the...Ch. 6 - Prob. 6.24ECh. 6 - (Knights Tour: Brute-Force Approaches) In Exercise...Ch. 6 - (Eight Queens) Another puzzler for chess buffs is...Ch. 6 - (Eight Queens: Brute-Force Approaches) In this...Ch. 6 - (Duplicate Elimination) In Chapter 12, we explore...Ch. 6 - (Knights Tour: Closed Tour Test) In the Knights...Ch. 6 - (The Sieve of Eratosthenes) A prime integer is any...Ch. 6 - Prob. 6.31RECh. 6 - (Linear Search) Modify the program of Fig. 6.18 to...Ch. 6 - (Binary Search) Modify the program of Fig. 6.19 to...Ch. 6 - Prob. 6.35RECh. 6 - Prob. 6.36RECh. 6 - Prob. 6.37RE
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
The file produced by the Java compiler containsthat are executed by the Java Virtual Machine.
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
This is a method that gets a value from a classs field, but does not change it. a. accessor b. constructor c. v...
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
A _______ is a diagram that graphically depicts the steps that take place in a program. a. flowchart b. step ch...
Starting Out with Python (4th Edition)
Draw the three Mohrs circles that describe each of the following states of stress.
Mechanics of Materials (10th Edition)
Explain the benefits of marking transaction boundaries, declaring lock characteristics, and letting a DBMS plac...
Database Concepts (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningOperations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks Cole
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
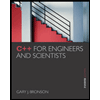
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
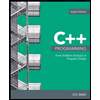
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
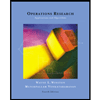
Operations Research : Applications and Algorithms
Computer Science
ISBN:9780534380588
Author:Wayne L. Winston
Publisher:Brooks Cole
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
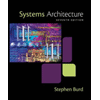
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
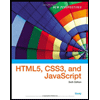
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Computational Software for Intelligent System Design; Author: Cadence Design Systems;https://www.youtube.com/watch?v=dLXZ6bM--j0;License: Standard Youtube License