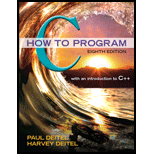
C How to Program (8th Edition)
8th Edition
ISBN: 9780133976892
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 6, Problem 6.9E
Consider a 2-by-5 integer array t.
- Write a definition for t.
- How many rows does t have?
- How many columns does t have?
- How many elements does t have?
- Write the names of all the elements in the second row of t.
- Write the names of all the elements in the third column of t.
- Write a single statement that sets the element of t in row 1 and column 2 to zero.
- Write a series of statements that initialize each element of t to zero. Do not use an iteration statement.
- Write a nested for statement that initializes each element of t to zero.
- Write a statement that inputs the values for the elements of t from the terminal.
- Write a series of statements that determine and print the smallest value in array t.
- Write a statement that displays the elements of the first row of t.
- Write a statement that totals the elements of the fourth column of t.
- Write a series of statements that print the array t in tabular format. List the column indices as headings across the top and list the row indices at the left of each row.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
This battle room is focused on entry level tasks for a network analyst where you will be given trials and reconnaissance, sensor tuning, log aggregation, SIEM queries, and network analysis.
For this week’s project, complete the following tasks:
From your Project Ares portal, LOG IN
Click on LAUNCH GAME.
Select the region NORTH AMERICA
Click on Battle School
Under the BATTLE SCHOOL pop-up window, click on START TRAINING.
Under the BATTLE ROOMS tile, click on ENTER.
Under the NETWORK ANALYST tile, click on PLAY.
Wait for the Battle Room to load. While loading, the BATTLE ROOM button will display red. Once the Battle Room is loaded, the BATTLE ROOM button will turn yellow and the center of the disk display will indicate CONNECTED. Click on the BATTLE ROOM button to enter the Battle Room.
Below the TASKS folder, make sure you click on INSTRUCTIONS to download the Network Analyst Fundamentals material.
In the Battle Room, under the TASKS menu select task INTRUSION DETECTION.
Complete…
Create a relationship between the common field (Technician Number) of the two tables. Make sure that each client must have 1 and only 1 technician assigned, and each technician can have multiple clients.
2. Create a query to show the Client Number, Client Name, Billed, Paid for clients in Anderson city. Save the query.
3. Create a query to show the Technician Number, Last Name, First Name, YTD Earnings for technicians whose Hourly Rate is greater than or equal to 30. Save the query.
4. Create a query to show Client Number, Client Name, Billed, Paid for clients whose technician number is 22 and whose Billed is over 300. Save the query.
5. Create a query to show the Technician Number, Last Name, First Name, Client Number, Client Name for clients whose technician number 23. Save the query.
6. Create a query to show the Technician Number, Last Name, First Name, Client Number, Client Name for clients whose technician number 23 or 29. Save the query Help please Microsoft office access
Dijkstra's Algorithm (part 1). Consider the network shown below, and Dijkstra’s link-state algorithm. Here, we are interested in computing the least cost path from node E (note: the start node here is E) to all other nodes using Dijkstra's algorithm. Using the algorithm statement used in the textbook and its visual representation, complete the "Step 0" row in the table below showing the link state algorithm’s execution by matching the table entries (i), (ii), (iii), and (iv) with their values. Write down your final [correct] answer, as you‘ll need it for the next question.
Chapter 6 Solutions
C How to Program (8th Edition)
Ch. 6 - Fill in the blanks in each of the following: C...Ch. 6 - State which of the following are true and which...Ch. 6 - Write statements to accomplish each of the...Ch. 6 - Consider a 2-by-5 integer array t. Write a...Ch. 6 - (Sales Commissions) Use a one-dimensional array to...Ch. 6 - (Bubble Sort) The bubble sort presented in Fig....Ch. 6 - Write loops that perform each of the following...Ch. 6 - Prob. 6.13ECh. 6 - (Mean, Median and Mode Program Modifications)...Ch. 6 - (Duplicate Elimination) Use a one-dimensional...
Ch. 6 - Label the elements of 3-by-5 two-dimensional array...Ch. 6 - What does the following program do?Ch. 6 - What does the following program do?Ch. 6 - (Dice Rolling) Write a program that simulates the...Ch. 6 - (Game of Craps) Write a program that runs 1000...Ch. 6 - Prob. 6.21ECh. 6 - (Total Sales) Use a two-dimensional array to solve...Ch. 6 - (Turtle Graphics) The Logo language made the...Ch. 6 - Prob. 6.24ECh. 6 - (Knights Tour: Brute-Force Approaches) In Exercise...Ch. 6 - (Eight Queens) Another puzzler for chess buffs is...Ch. 6 - (Eight Queens: Brute-Force Approaches) In this...Ch. 6 - (Duplicate Elimination) In Chapter 12, we explore...Ch. 6 - (Knights Tour: Closed Tour Test) In the Knights...Ch. 6 - (The Sieve of Eratosthenes) A prime integer is any...Ch. 6 - Prob. 6.31RECh. 6 - (Linear Search) Modify the program of Fig. 6.18 to...Ch. 6 - (Binary Search) Modify the program of Fig. 6.19 to...Ch. 6 - Prob. 6.35RECh. 6 - Prob. 6.36RECh. 6 - Prob. 6.37RE
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Each repetition of a loop is known as a(n) ______. a. cycle b. revolution c. orbit d. iteration
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Assume a telephone signal travels through a cable at two-thirds the speed of light. How long does it take the s...
Electric Circuits. (11th Edition)
What does the following code print? System.out.println(""); System.out.println(""); System.out.println(""); Sys...
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
What is a reference type? Are class types reference types? Are primitive types, such as int, reference types?
Java: An Introduction to Problem Solving and Programming (8th Edition)
Porter’s competitive forces model: The model is used to provide a general view about the firms, the competitors...
Management Information Systems: Managing The Digital Firm (16th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
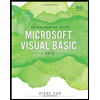
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
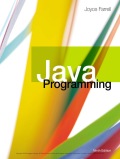
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
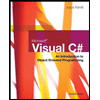
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
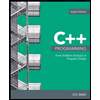
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
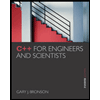
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
9.1: What is an Array? - Processing Tutorial; Author: The Coding Train;https://www.youtube.com/watch?v=NptnmWvkbTw;License: Standard Youtube License