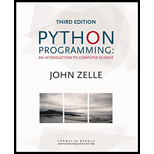
Python Programming: An Introduction to Computer Science, 3rd Ed.
3rd Edition
ISBN: 9781590282755
Author: John Zelle
Publisher: Franklin, Beedle & Associates
expand_more
expand_more
format_list_bulleted
Question
Chapter 5, Problem 8PE
Program Plan Intro
Program to encrypt a message in circular fashion
Program plan
- Inside the “main()” function,
- Declare “plaintext” and “key” and get input from the user.
- Create a string of letters and then convert plaintext to ciphertext.
- Print the encoded message.
- Call the function “main()”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Example 2
Enter message to be encrypted: Dèyè mòn, gen mòn.
Enter step size: -1
ORIGINAL MESSAGE
Dèyè mòn, gen mòn.
ENCRYPTED MESSAGE
Cçxç lim, fdm lim.
Enter message to be encrypted: Dèyè mòn, gen mòn.
Enter step size: 0
ORIGINAL MESSAGE
Dèyè mòn, gen mòn.
ENCRYPTED MESSAGE
Dèyè mòn, gen mòn.
Enter message to be encrypted: QUIT
Example 3
Enter message to be encrypted: Perfer et obdura, dolor hic tib:
proderit olim.
Enter step size: 8
ORIGINAL MESSAGE
Perfer et obdura, dolor hic tibi proderit olim.
ENCRYPTED MESSAGE
Xmznmz m| wjl)zi, lwtwz pąk |qją xzwlmzą| wtqu.
Enter message to be encrypted: QUIT
Write a program to read dates from input, one date per line. Each date's format must be as follows: March 1, 1990. Any date not following that format is incorrect and should be ignored. The input ends with -1 on a line alone. Output each correct date as: 3/1/1990.
Hint: Use string[start:end] to get a substring when parsing the string and extracting the date. Use the split() method to break the input into tokens.
Ex: If the input is:
March 1, 1990 April 2 1995 7/15/20 December 13, 2003 -1
then the output is:
3/1/1990 12/13/2003
use PYTHON
starting with :
months = { "January": 1, "February": 2, "March": 3, "April": 4, "May": 5, "June": 6, "July": 7, "August": 8, "September": 9, "October": 10, "November": 11, "December": 12,}
import refor data in ['March 1, 1990', 'April 2 1995', '7/15/20', 'December 13, 2003', -1]: if data == -1: break if re.match(r'(\w+) (\d{1,2}), (\d{4})', data) == None: continue output = data.split(" ")…
A simple and very old method of sending secret messages is the substitution cipher.
You might have used this cipher with your friends when you were a kid.
Basically, each letter of the alphabet gets replaced by another letter of the alphabet.
For example, every 'a' get replaced with an 'X', and every 'b' gets replaced with a 'Z', etc.
Write a program thats ask a user to enter a secret message.
Encrypt this message using the substitution cipher and display the encrypted message.
Then decryped the encrypted message back to the original message.
You may use the 2 strings below for your subsitition.
For example, to encrypt you can replace the character at position n in alphabet
with the character at position n in key.
To decrypt you can replace the character at position n in key
with the character at position n in alphabet.
Have fun! And make the cipher stronger if you wish!
Currently the cipher only substitutes letters - you could easily add digits, puncuation, whitespace and so
forth.…
Chapter 5 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
Ch. 5 - Prob. 1TFCh. 5 - Prob. 2TFCh. 5 - Prob. 3TFCh. 5 - Prob. 4TFCh. 5 - Prob. 5TFCh. 5 - Prob. 6TFCh. 5 - Prob. 7TFCh. 5 - Prob. 8TFCh. 5 - Prob. 9TFCh. 5 - Prob. 10TF
Ch. 5 - Prob. 1MCCh. 5 - Prob. 2MCCh. 5 - Prob. 3MCCh. 5 - Prob. 4MCCh. 5 - Prob. 5MCCh. 5 - Prob. 6MCCh. 5 - Prob. 7MCCh. 5 - Prob. 8MCCh. 5 - Prob. 9MCCh. 5 - Prob. 10MCCh. 5 - Prob. 1DCh. 5 - Prob. 2DCh. 5 - Prob. 3DCh. 5 - Prob. 4DCh. 5 - Prob. 5DCh. 5 - Prob. 1PECh. 5 - Prob. 2PECh. 5 - Prob. 3PECh. 5 - Prob. 4PECh. 5 - Prob. 5PECh. 5 - Prob. 6PECh. 5 - Prob. 7PECh. 5 - Prob. 8PECh. 5 - Prob. 9PECh. 5 - Prob. 10PECh. 5 - Prob. 11PECh. 5 - Prob. 12PECh. 5 - Prob. 13PECh. 5 - Prob. 14PECh. 5 - Prob. 15PECh. 5 - Prob. 16PE
Knowledge Booster
Similar questions
- Implement a mechanism for performing basic string compression using repeated character counts. The string aabccccaaa, for example, would become a2blc5a3. Your method should return the original string if the "compressed" string does not grow smaller than the original string. You can assume that the string only contains uppercase and lowercase letters (a-z).arrow_forwardThe Reflection algorithm can be used to encrypt a C-string (character array terminated with the null character) before it is transmitted over the Internet. The Reflection algorithm works as follows: Each letter in the message is converted to the letter in that is 13 positions to the right of that letter in the English alphabet. If the end of the alphabet is reached, counting continues from the first letter in the alphabet. The case of the letters must be maintained. For example,'M' →'Z', 'x' → 'k', 'A' → 'N'The numeric characters ('0' to '9') are shifted 5 positions to the right of that number in the character set '0' to '9'. If ‘9’ is reached, counting continues from ‘0’. For example,'0' → '5', '2' →'7', '8' → '3' All other characters are left as they are. a) What would the following string be encrypted to by the Reflection algorithm?“Call me at 662-2002 Ext 85393” b) Write a function, getPosition, which finds the position of a letter in the alphabet regardless of the case of the…arrow_forwardWrite a program that creates an input function to enter a string. Then use .isalpha method to find ifthe first character in the string starts with a letter or a non-letter. (Hint: use indexing and if and if notfunction). Make sure to print the two statements ‘Your string starts with a letter’ and ‘Your stringcontains a non-letter’)arrow_forward
- Print the total number of words in the file. Print the total number of different words (case sensitive, meaning “We” and “we” are two different words) in the file. Print all words in ascending order (based on the ASCII code) without duplication. Write a pattern match method to find the location(s) of a specific word (a character string up to twelve characters, e.g. system). This method should return all line number(s) and location(s) of the word(s) found in the file. Print all line(s) with line number(s) of the file where the word is funds by invoking the method of 4). Under each output line indicate the location(s) of the first character of the matched word (refer to the output example below). Empty lines are lines, they also have their own unique line numbers. java Project mytext.txt The total number of word in the file is: 501 The total number of different words in the file is: 465 Words of the input file in ascending order without duplication: // here are output of all words in…arrow_forwardA Caesar cipher is a simple substitution cipher based on the idea of shifting each letter of the plaintext message a fixed number (called the key) of positions in the alphabet. For example, if the key value is 2, the word "Sourpuss" would be encoded as "Uqwtrwuu." The original message can be recovered by “reencoding" it using the negative of the key. Write a program that can encode and decode Caesar ciphers. The in- put to the program will be a string of plaintext and the value of the key. The output will be an encoded message where each character in the orig- inal message is replaced by shifting it key characters in the Unicode char- acter set. For example, if ch is a character in the string and key is the amount to shift, then the character that replaces ch can be calculated as: chr (ord (ch) + key). 62arrow_forwardImplement a method to perform basic string compression using the counts of repeated characters. For example, the string aabcccccaaa would become a2blc5a3. If the "compressed" string would not become smaller than the original string, your method should return the original string. You can assume the string has only uppercase and lowercase letters (a - z).arrow_forward
- You are given a string. Get input from the user. In the first line, print the third character of this string. In the second line, print the second to last character of this string. In the third line, print the first five characters of this string. In the fourth line, print all but the last two characters of this string. In the fifth line, print all the characters of this string with even indices (remember indexing starts at 0, so the characters are displayed starting with the first). In the sixth line, print all the characters of this string with odd indices (i.e. starting with the second character in the string). In the seventh line, print all the characters of the string in reverse order. 8. In the eighth line, print every second character of the string in reverse order, starting from the last one. In the ninth line, print the length of the given string.arrow_forwardDevelop a program that gets a string from the user and prints it in the reverse order. Use a safe gets/scanf to make sure the user doesn’t mess up memory. Assume that the maximum characters allowed for the string is 200 character (use c code)arrow_forwardWrite a Program using any programming language you are comfortable with to implement Ceasar Shift cipher technique. The algorithm should take “plaint text” and key as input and gives the cipher text as output.arrow_forward
- This is a substitution cypherEach letter is replaced by another letter with no repetitionIgnore spaces and punctuationYou must account for spaces (I ignored them, but there are other legitimate responses.)The best program will also account for punctuation and other symbols. (Ignoring them is fine.) Your program can ignore these special characters when they occur, but it should not crash if it encounters a strange character.Manage CapitalizationIn most cryptographic applications, all letters are converted to uppercase. Please follow this convention or deal with potential case conversion problems in another way.Use FunctionsYou may not make significant changes to the main function. Determine which functions are necessary and create them. (The blackbelt project does allow for one slight modification, noted below.) Do not turn in a program with only a main() function!! Write the code necessary for Python to run the main function as expectedPass data between functionsYour functions may…arrow_forwardGiven two strings, s1 and s2, select only the characters in each string where the character in the same position in the other string is in uppercase. Return these as a single string. To illustrate, given the strings s1 = "heLLo" and s2 = "GUlp", we select the letters "he" from s1, because "G" and "U" are uppercase. We then select "Ip" from s2, because "LL" is in uppercase. Finally, we join these together and return "help". Examples selectLetters("heLLO", "GUlp") "GUlp") → "help" selectLetters("1234567", "XxXxX") → "135" wwwwwwwwwww. selectLetters("EVERYTHING", "SomeThings")→ "EYSomeThings"arrow_forwardgiven the strings s1 and s2, not necessarily of the same lenght, create a new string consisting of alternating characters of s1 and s2 (that is, the first character of s1 followed by the first character of s2, followed by the second characters of s1, followed by the second character of s2, and so on. Once the end of either string is reached, the reminder of the longer string is added to the end of the new string. For example, if s1 contained "abc" and s2 contained"uvqxyz", then the new string should contain "aubvcwxyz". Assign the new string to the variable s3. using python and loopsarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
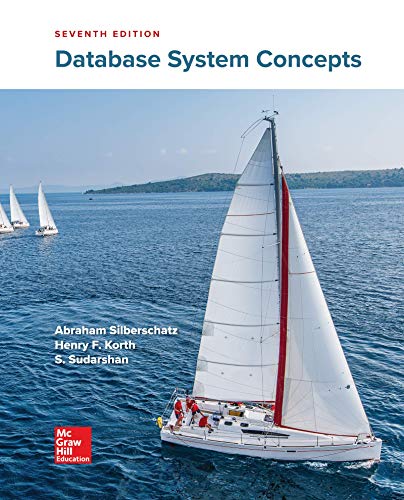
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
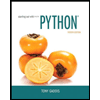
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
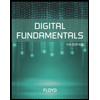
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
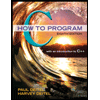
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
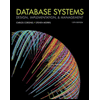
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
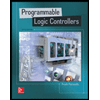
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education