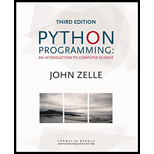
Python Programming: An Introduction to Computer Science, 3rd Ed.
3rd Edition
ISBN: 9781590282755
Author: John Zelle
Publisher: Franklin, Beedle & Associates
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 5, Problem 15PE
Program Plan Intro
Horizontal bar graph
Program plan:
- Define the main method
- Get the file name from the user
- Read the number of students and assign to “numStud” variable
- Read the names, grades from the file and store it in the variables
- Read the names from the file line by line
- Split the line
- Strip the numbers
- Append the student names and grades
- Display the student name and grade
- Set graph win size
- Set the coords of the graph
- Iterate “i” till it reaches “numStud”
- Set the name
- Set the text
- Iterate “i” till it reaches “numStud”
- Draw a rectangle box
- Draw the line
- Close the file
- Call the function “main()”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
A python function opens file data.csv, reads the data from the file and spits the data into three columns, x, y and z. The data is then printed out.
Function:
f = open("data.csv ", "r")
print('x\ty\tz'.format())
for row in f:
temp = row.split(',')
for cell in temp:
print(cell,end='\t')
print()
data.csv contains:
1.2,2.1,1.1
2.3,3.2,0.6
0.7,1.9,0.1
1.8,2.5,0.3
4.6,2.7,0.9
pic for input and output
pic link: fname = "https://runestone.academy/runestone/static/instructorguide/_static/arch.jpg
In Python Write a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements:
Each row contains the title, rating, and all showtimes of a unique movie.
A space is placed before and after each vertical separator ('|') in each row.
Column 1 displays the movie titles and is left justified with a minimum of 44 characters.
If the movie title has more than 44 characters, output the first 44 characters only.
Column 2 displays the movie ratings and is right justified with a minimum of 5 characters.
Column 3 displays all the showtimes of the same movie, separated by a space.
Each row of the CSV file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows.
Ex: If the input of the program is:
movies.csv
and the contents…
Chapter 5 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
Ch. 5 - Prob. 1TFCh. 5 - Prob. 2TFCh. 5 - Prob. 3TFCh. 5 - Prob. 4TFCh. 5 - Prob. 5TFCh. 5 - Prob. 6TFCh. 5 - Prob. 7TFCh. 5 - Prob. 8TFCh. 5 - Prob. 9TFCh. 5 - Prob. 10TF
Ch. 5 - Prob. 1MCCh. 5 - Prob. 2MCCh. 5 - Prob. 3MCCh. 5 - Prob. 4MCCh. 5 - Prob. 5MCCh. 5 - Prob. 6MCCh. 5 - Prob. 7MCCh. 5 - Prob. 8MCCh. 5 - Prob. 9MCCh. 5 - Prob. 10MCCh. 5 - Prob. 1DCh. 5 - Prob. 2DCh. 5 - Prob. 3DCh. 5 - Prob. 4DCh. 5 - Prob. 5DCh. 5 - Prob. 1PECh. 5 - Prob. 2PECh. 5 - Prob. 3PECh. 5 - Prob. 4PECh. 5 - Prob. 5PECh. 5 - Prob. 6PECh. 5 - Prob. 7PECh. 5 - Prob. 8PECh. 5 - Prob. 9PECh. 5 - Prob. 10PECh. 5 - Prob. 11PECh. 5 - Prob. 12PECh. 5 - Prob. 13PECh. 5 - Prob. 14PECh. 5 - Prob. 15PECh. 5 - Prob. 16PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a code segment that searches a Grid object for a negative integer. The loop should terminate at the first instance of a negative integer in the grid, and the variables row and column should be set to the position of that integer. Otherwise, the variables row and column should equal the number of rows and columns in the grid. *Pythonarrow_forwardYou have been asked to develop the new system. You are to write an application to assign seats in an airline. Assume a small airplane with 28 seats (7 rows 4 wide) as follows: 1 A B C D 2 A B C D 3 A B C D 4 A B C D 5 A B C D 6 A B C D 7 A B CD The program should display the seat pattern, with an X marking the seats already assigned. 1 X B C D 2 A X C D 3 A B C D 4 A B X D 5 A B C D 6 A B C D 7 A B C D The program then requests a choice of seat. If the seat if available,then the seating display is updated. Otherwise, the program requests another choice of seat. If the user types in a seat that is already assigned, the program should say that that seat is occupied and ask for another choice. This continues until all seats are filled or until the user says that the program should end.arrow_forwardDesign a program that will use a pair of nested loop to read the company's sales amounts. The details are as follows: -KDS Company has 3 departments: 1. Dept X 2. Dept Y 3. Dept Z. Design a program that will read as input each department's sales for each quarter of the year, and display the result for all divisions. Use a two dimensional array that will have 3 rows and 4 columns and show how the data will be organized. Please provide solution using Python. Thank you.arrow_forward
- In this assignment you will write a program that shows the valid moves of chess pieces. Your program will draw a board with 64 squares using the traditional layout, next ask the user to choose a move, and then, depending on the user's choice, redraw the board with the selected chess piece and its valid moves. Please see the examples of valid moves of chess pieces and the traditional chess board layout below:arrow_forwardprogram that uses 2 arrays - one array containing the high temperature per day for the last 10 days, and one array containing the daily low temperature for the last 10 days. using the weather channel tempts to calculate and output the average. high and average low temperature over the period.arrow_forwardIn python, Old-fashioned photographs from the nineteenth century are not quite black and white and not quite color, but seem to have shades of gray, brown, and blue. This effect is known as sepia, as shown in the figures below. Original Sepia Original Image & Sepia Image Write and test a function named sepia that converts a color image to sepia. This function should first call grayscale to convert the color image to grayscale. A code segment for transforming the grayscale values to achieve a sepia effect follows.Note that the value for green does not change. (red, green, blue) = image.getPixel(x, y)if red < 63: red = int(red * 1.1) blue = int(blue * 0.9)elif red < 192: red = int(red * 1.15) blue = int(blue * 0.85)else: red = min(int(red * 1.08), 255) blue = int(blue * 0. 1. GUI TEST 2. CUSTOM TEST 3. IMAGE TESTarrow_forward
- Assignment Write a program that moves a forester unit and a desert corps unit to a given target location and prints the number of hours it took for each unit to reach the target. First, the user will give the starting X and Y positions of the forester unit as well as its base speed (all ints). Then, the starting X and Y positions of the desert corps unit as well as its base speed (all ints). Finally, the target X and Y positions (both ints) and the terrain type (std::string). Speeds are given as distance traveled in one full day (i.e., 24 hours) and is affected by the terrain type using a multiplier over the base speed. Also, after 1 full day, each unit rests for a certain number of hours. Speed multiplier and rest amount of each type of unit depending on the terrain type are as below. Speed Multiplier Rest Duration After 1 day Terrain type Forester Desert Corps Forester Desert Corps Forest 1.3 0.6 4 12 Desert 0.4 1.2 15 6 Hills 0.7 0.8 6 8 Others 1.0 0.8 8 6 After getting all this…arrow_forwardExtend the program below so that after closing the files, program asks the user to enter a name. The program should store this input as a string, and then search the name array for the ‘search name’ just entered by the user. If the search name is found in the name array, the program will print the name, age and wage corresponding to the search name to the screen. If the search name is not found in the name array, program prints “Name not found” to the screen. The search would need to use string comparison functions. (b) #include<stdio.h>#include<stdlib.h> char c;char name[10][50];int roll[10];float marks[10]; int main (int argc, char **argv) { FILE* f; if (argc != 2) { printf("No filename in the argument"); return 1; } f = fopen(argv[1], "r"); if (f == NULL) { printf("The file cannot be opened successfully: %s\n",argv[1]); return 1; } printf("The file has been opened successfully\n\n"); int lines = 0;…arrow_forwardWrite a program that uses an array of string objects to hold the five student names, an array of five characters to hold the five students' letter grades, and five arrays of four doubles to hold each student's set of test scores. The program should allow the user to enter each student's name and his or her four test scores. It should then calculate and display each student's average test score and a letter grade based on the average. Input Validation: Do not accept test scores less than 0 or greater than 100. Use the folloiwing test score grades Test Score Letter Grade 80-100 -> A 70-79 -> B 60-69 -> C 50-59 -> Darrow_forward
- Write a program that uses an array of string objects to hold the five student names, an array of five characters to hold the five students’ letter grades, and five arrays of four doubles to hold each student’s set of test scores. The program should allow the user to enter each student’s name and his or her four test scores. It should then calculate and display each student’s average test score and a letter grade based on the average. Input Validation: Do not accept test scores less than 0 or greater than 100. Use the folloiwing test score grades Test Score Letter Grade80–100 –> A70–79 –> B60–69 –> C50–59 –> D i was also told to create a database for this projectarrow_forward3. Randomly generate a number in range [1, 12]. Map this number to a season: if the number is 3, 4, or 5, map it to “Spring", if the number is 6, 7, or 8, map it to "Summer", if the number is 9, 10, or 11, map it to "Fall", and if the number 12, 1, or 2, map it to "Winter". Display season namearrow_forwardExtend the program belowso that after closing the files, program asks the user to enter a name. The program should store this input as a string, and then search the name array for the ‘search name’ just entered by the user. If the search name is found in the name array, the program will print the name, age and wage corresponding to the search name to the screen. If the search name is not found in the name array, your program prints “Name not found” to the screen. The search would need to use string comparison functions. #include<stdio.h>#include<stdlib.h> char c;char name[10][50];int roll[10];float marks[10]; int main (int argc, char **argv) { FILE* f; if (argc != 2) { printf("No filename in the argument"); return 1; } f = fopen(argv[1], "r"); if (f == NULL) { printf("The file cannot be opened successfully: %s\n",argv[1]); return 1; } printf("The file has been opened successfully\n\n"); int lines = 0;…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
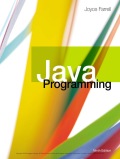
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
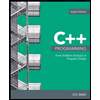
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Constants, Variables, Data types, Keywords in C Programming Language Tutorial; Author: LearningLad;https://www.youtube.com/watch?v=d7tdL-ZEWdE;License: Standard YouTube License, CC-BY