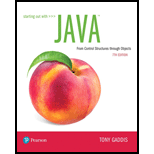
Concept explainers
Celsius Temperature Table
The formula for converting a temperature from Fahrenheit to Celsius is
where F is the Fahrenheit temperature and C is the Celsius temperature. Write a method named celsius that accepts a Fahrenheit temperature as an argument. The method should return the temperature, converted to Cetsius. Demonstrate the method by calling it in a loop that displays a table of the Fahrenheit temperatures 0 through 20 and their Celsius equivalents.

Trending nowThis is a popular solution!

Chapter 5 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Starting Out with Programming Logic and Design (4th Edition)
Artificial Intelligence: A Modern Approach
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Starting Out with C++ from Control Structures to Objects (8th Edition)
Software Engineering (10th Edition)
- In this exercise, you modify the Grade Calculator application from this chapter’s Apply lesson. Use Windows to make a copy of the Grade Solution folder. Rename the copy Grade Solution-Intermediate. Open the Grade Solution.sln file contained in the Grade Solution-Intermediate folder. Open the CourseGrade.vb file. The DetermineGrade method should accept an integer that represents the total number of points that can be earned in the course. (Currently, the total number of points is 200: 100 points per test.) For an A grade, the student must earn at least 90% of the total points. For a B, C, and D grade, the student must earn at least 80%, 70%, and 60%, respectively. If the student earns less than 60% of the total points, the grade is F. Make the appropriate modifications to the DetermineGrade method and then save the solution. Unlock the controls on the form. Add a label control and a text box to the form. Change the label control’s Text property to “&Maximum points:” (without the quotation marks). Change the text box’s name to txtMax. Lock the controls and then reset the tab order. Open the form’s Code Editor window. The txtMax control should accept only numbers and the Backspace key. Code the appropriate procedure. The grade should be cleared when the user makes a change to the contents of the txtMax control. Code the appropriate procedure. Modify the frmMain_Load procedure so that each list box displays numbers from 0 through 200. Locate the btnDisplay_Click procedure. If the txtMax control does not contain a value, display an appropriate message. The maximum number allowed in the txtMax control should be 400; if the control contains a number that is more than 400, display an appropriate message. The statement that calculates the grade should pass the maximum number of points to the studentGrade object’s DetermineGrade method. Make the necessary modifications to the procedure. Save the solution and then start and test the application.arrow_forward// Question 4: // Declare an integer variable named "variableQ4" with an initial value of 10. // Write a method named "SetToOne" that takes one out parameter. // The method should set the out parameter to 1. // Call the method SetToOne passing variableQ4 in the btnQ4 click method. // Display the variable variableQ4 in the lblQ4. 1 reference private void btnQ4_Click(object sender, EventArgs e) { }arrow_forwardin c# please Write method QualityPoints that inputs a student’s average and returns 4 if the student’s average is 90–100, 3 if the average is 80–89, 2 if the average is 70–79, 1 if the average is 60–69 and 0 if the average is lower than 60. Incorporate the method into an app that reads a value from the user and displays the result.arrow_forward
- Please add commentsarrow_forwardA Java Practical Assignments.pdf - Adobe Acrobat Pro DC (32-bit) File Edit View E-Sign Window Help Home Tools Java Practical Assig. x Sign In 1 /1 139% Java Practical Assignments 1. Write an application that throws and catches an ArithmeticException when you attempt to take the square root of a negative value. Prompt the user for an input value and try the Math.sqrt() method on it. The application either displays the square root or catches the thrown Exception and displays an appropriate message. 2. Create a program that allows a user to input customer records (ID number, first name, last name, and balance owed) and save each record to a file. 3. Write an application for Limpopo's Car Care Shop that shows a user a list of available services: oil change, tire rotation, battery check, or brake inspection. Allow the user to enter a string that corresponds to one of the options, and display the option and its price as R250, R220, R150, or R50, accordingly. Display an error message if the…arrow_forward15. Even/Odd CounterYou can use the following logic to determine whether a number is even or odd: if ((number % 2) == 0){ // The number is even.}else{ // The number is odd.}Write a program with a method named isEven that accepts an int argument. The method should return true if the argument is even, or false otherwise. The program’s main method should use a loop to generate 100 random integers. It should use the isEven method to determine whether each random number is even, or odd. When the loop is finished, the program should display the number of even numbers that were generated, and the number of odd numbers.arrow_forward
- Create a method that randomly select a letter in the alphabetarrow_forwardPosted don't copy the existing code.arrow_forwardPYTHON PROGRAMMING Reza Enterprises sells tickets for buses, tours, and other travel services. Because Reza frequently mistypes long ticket numbers, Reza Enterprises has asked his students to write an application that shows if a ticket is invalid. Your application/program tells the ticket agent to enter a six-digit ticket number. Ticket numbers are designed so that if you lose the last digit of the number, then divide by 7, the remainder of the division is exactly the same to the last dropped digit. This process is shown below: Step 1: Enter the ticket number; for example 123454 Step 2: Remove the last digit, leaving 12345 Step 3: Determine the remainder when the ticket number from step 2 is divided by 7. In this case, 12345 divided by 7 leaves a remainder of 4. Step 4: Display a message to the ticket agent indicating whether the ticket number is valid or not. If the ticket number is valid, save the number to a .txt file called “tickets.txt” and continue to loop your program…arrow_forward
- Starting with the following code, add a loop that will prompt the user for the number of math questions the user would like to be presented with: import randomfirstnum = random.randrange(1,11) # return an int from 1 to 10secondnum = random.randrange(1, 11)compsum = firstnum + secondnum # adds the 2 random numbers together# print (compsum) # print for troubleshootingprint("What is the sum of", firstnum, " +", secondnum, "?") # presents problem to useradded = int(input("Your answer is: ")) # gets user inputif added == compsum: # compares user input to real answer print("You are correct!!!")else: print ("Sorry, you are incorrect")arrow_forwardpublic setUpTrip(double, double): void Car's state is set to hold the speed of travel and distance to travel at that speed Precondition: none Postcondition: Car's state holds information on distance to travel and speed to travel Parameters: Average Speed to be driven, Distance to drive Develop and use an algorithm that calculates the amount of fuel used and the actual distance driven in the drive() method. The algorithm must use a formula that gives proportionately poorer mileage when the Car is driven faster or slower than its optimal speed. When a new Car object is instantiated, it is initialized with an optimal speed variable. Your fuel usage algorithm should set limits on how poor of MPG your car will get. You may add other methods and fields as needed. When a new Car object is created, the car’s odometer is set to a random number between 0.0 and 5.99, the car’s trip odometer is set to 0.0, its best fuel economy (MPG) is set to a random number between 15.0 and 54.99, its…arrow_forwardThe weather generator methods you will be writing for this assignment will: predict future precipitation pattern for one month: oneMonthGenerator find the number of wet or dry days in a given month’s forecast: numberOfWetDryDays find the longest wet or dry spell in a given month’s forecast: lengthOfLongestWetDrySpellarrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
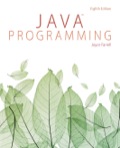
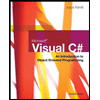
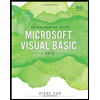