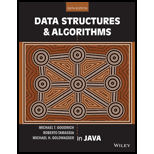
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 5, Problem 17C
Write a short recursive Java method that takes a character string s and outputs its reverse. For example, the reverse of 'pots&pans' would be 'snap&stop'.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Using the notation
you can select multipy options
For each of the following, decide whether the claim is True or False and select the True ones:
Suppose we discover that the 3SAT can be solved in worst-case cubic time. Then it would
mean that all problems in NP can also be solved in cubic time.
If a problem can be solved using Dynamic Programming, then it is not NP-complete.
Suppose X and Y are two NP-complete problems. Then, there must be a polynomial-time
reduction from X to Y and also one from Y to X.
Chapter 5 Solutions
Data Structures and Algorithms in Java
Ch. 5 - Prob. 1RCh. 5 - Prob. 2RCh. 5 - Prob. 3RCh. 5 - Prob. 4RCh. 5 - Prob. 5RCh. 5 - Draw the recursion trace for the execution of...Ch. 5 - Prob. 7RCh. 5 - Describe a recursive algorithm for converting a...Ch. 5 - Prob. 9RCh. 5 - Prob. 10R
Ch. 5 - Prob. 11CCh. 5 - Prob. 12CCh. 5 - Give a recursive algorithm to compute the product...Ch. 5 - In Section 5.2 we prove by induction that the...Ch. 5 - Write a recursive method that will output all the...Ch. 5 - In the Towers of Hanoi puzzle, we are given a...Ch. 5 - Write a short recursive Java method that takes a...Ch. 5 - Write a short recursive Java method that...Ch. 5 - Use recursion to write a Java method for...Ch. 5 - Write a short recursive Java method that...Ch. 5 - Prob. 21CCh. 5 - Prob. 22CCh. 5 - Prob. 23CCh. 5 - Isabel has an interesting way of summing up the...Ch. 5 - Prob. 25CCh. 5 - Prob. 26CCh. 5 - Prob. 27PCh. 5 - Write a program for solving summation puzzles by...Ch. 5 - Prob. 29PCh. 5 - Write a program that can solve instances of the...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Write an equals method for the class Person described in Self-Test Question 16.
Java: An Introduction to Problem Solving and Programming (8th Edition)
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
Define each of the following terms: supertype subtype specialization entity cluster completeness constraint enh...
Modern Database Management
Write statements that assign random integers to the variable n in the following ranges: 1 1n2. 2 1n100. 3 0n9. ...
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
What does the throw statement do?
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
ICA 8-57
A 100-watt [W] motor (60% efficient) is available to raise a load 5 meters [m] into the air. If the ta...
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Maximum Independent Set problem is known to be NP-Complete. Suppose we have a graph G in which the maximum degree of each node is some constant c. Then, is the following greedy algorithm guaranteed to find an independent set whose size is within a constant factor of the optimal? 1) Initialize S = empty 2) Arbitrarily pick a vertex v, add v to S delete v and its neighbors from G 3) Repeat step 2 until G is empty Return S Yes Noarrow_forwardPlease help me answer this coding question in the images below for me(it is not a graded question):write the code using python and also provide the outputs requiredarrow_forwardWhat does the reduction showing Vertex Cover (VC) is NP-Complete do: Transforms any instance of VC to an instance of 3SAT Transforms any instance of 3SAT to an instance of VC Transforms any instance of VC to an instance of 3SAT AND transforms any instance of 3SAT to an instance of VC none of the abovearrow_forward
- Please assist me by writing out the code with its output (in python) using the information provided in the 2 images below.for the IP Address, it has been changed to: 172.21.5.204the serve code has not been open yet though but the ouput must be something along these lines(using command prompt):c:\Users\japha\Desktop>python "Sbongakonke.py"Enter the server IP address (127.0.0.1 or 172.21.5.199): 172.21.5.204Enter your student number: 4125035Connected to server!It's your turn to pour! Enter the amount to your pour (in mL):Please work it out until it gets the correct outputsNB: THIS QUESTION IS NOT A GRADED QUESTIONarrow_forwardneed help with a html code and css code that will match this image.arrow_forwardneed help with a html code and css code that will match this image. Part B - A Navigation Part B is the navigation component of a page. Information you need includes: Color Codes: Visiting links: #ff6666 Unvisited links: #ccff66 Hovered links: white Search box: #2ec4b6 rebeccapurple white Font: Google Font (Roboto) Icons: Font Awesome (fa-quidditch, fa-search) This is a flexbox based navigation menu. Other then padding, all spacing/positioning should be controlled using flex properties. The home link in the nav should point to your assignment file (to triggers visited styling). In the "state" screenshot below, Home is visited, Services is hovered (the mouse doesn't show up in the screenshot) and Products is unvisited.arrow_forward
- MGMT SS STATS, an umbrella body that facilitates and serves various Social Security Organizations/Departments within the Caribbean territories, stood poised to meet the needs of its stakeholders by launching an online database. The database will provide members and the public access to the complete set of services that can (also) be initiated face-to-face, and it will provide managed, private, secure access to a repository of public and/or personal information. Ideally, the database will have basic details of pension plans recorded in the registry, member plan statistics, and cash inflows and outflows from pension funds.For example, insured persons accumulate contributions. Records for these persons will include information on the insured persons able to acquire various benefits once work is interrupted due to sickness, death, retirement, and maternity or employment injury. They will also include information on pensions such as invalidity, disability, and survivors that stem from one…arrow_forwardWhy all appvif i want to sign in its required phone number why not using google or apple its make me frustratedarrow_forwardWhy is the accuracy of time important in data visualizations? Detail a scenario from your professional experience in which time was structured poorly in a data visualization. How did this affect the understanding of the data presented? How do you think this error or oversight occurred?arrow_forward
- Write the KeanStudent class. The UML diagram of the class is represented below: KeanStudent - fullName: String - keanID: int -keanEmailAddress: String cellPhoneNumber: String + numberOfStudent: int + KeanStudent() + KeanStudent(fullName: String, keanID: int, keanEmailAddress: String, cellPhoneNumber: String) +getFullName(): String +setFullName(newFullName: String): void +getKeanIDO): int +getKeanEmailAddress(): String +getCellPhoneNumber(): String + setCellPhoneNumber(newCellPhoneNumber: String): void +toString(): String 1. Implement the KeanStudent class strictly according to its UML one-to-one (do not include anything extra, do not miss any data fields or methods) 2. Implement a StudentTest class to test the class KeanStudent you just created. • Create two KeanStudent objects using a no-args constructor and one from the constructor with all fields. o Print the contents of both objects. 。 Print numberOfStudent. 3. Add comments to your program (mark where data fields, constructors,…arrow_forwardMGMT SS STATS, an umbrella body that facilitates and serves various Social SecurityOrganizations/Departments within the Caribbean territories, stoodpoised to meet the needs of its stakeholders by launching an onlinedatabase at www.SSDCI.gov. The database will provide membersand the public access to the complete set of services that can (also)be initiated face-to-face, and it will provide managed, private, secure access to a repository ofpublic and/or personal information. Ideally, the database will have basic details of pensionplans recorded in the registry, member plan statistics, and cash inflows and outflows frompension funds.For example, insured persons accumulate contributions. Records for these persons will includeinformation on the insured persons able to acquire various benefits once work is interrupteddue to sickness, death, retirement, and maternity or employment injury. They will also includeinformation on pensions such as invalidity, disability, and survivors that stem from…arrow_forward(c) Consider the following set of processes: Process ID Arrival Time Priority Burst Time A 2 3 100 B 6 C 10 1 (highest) 2 40 80 D 16 4 (lowest) 20arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengagePrinciples of Information Systems (MindTap Course...Computer ScienceISBN:9781285867168Author:Ralph Stair, George ReynoldsPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
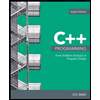
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
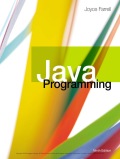
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
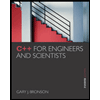
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
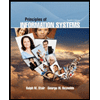
Principles of Information Systems (MindTap Course...
Computer Science
ISBN:9781285867168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
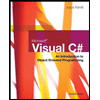
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Introduction to Big O Notation and Time Complexity (Data Structures & Algorithms #7); Author: CS Dojo;https://www.youtube.com/watch?v=D6xkbGLQesk;License: Standard YouTube License, CC-BY