Write a recursive method in java public static long numPaths(int r, int c) which can solve the following scenario: a checker is placed on a checkerboard. What are the fewest moves to get to the upper left corner(0,0)? You can only move sideways or vertically. No diagonal moves. Once you have that calculation, how many different ways are there to get to the upper left corner? this is my code but I am getting stock overflow, I'm not reaching my base case. public static long numPaths(int r, int c) { //base case if (r==0 && c==0 || r==0 || c==0) return 1; else { return numPaths(r, c--) + numPaths(r--, c); } }
Write a recursive method in java public static long numPaths(int r, int c)
which can solve the following scenario: a checker is placed on a checkerboard.
What are the fewest moves to get to the upper left corner(0,0)? You can only move sideways or
vertically. No diagonal moves.
Once you have that calculation, how many different ways are there to get to the
upper left corner?
this is my code but I am getting stock overflow, I'm not reaching my base case.
public static long numPaths(int r, int c)
{
//base case
if (r==0 && c==0 || r==0 || c==0)
return 1;
else {
return numPaths(r, c--) + numPaths(r--, c);
}
}


The approach of this problem is right. The recursive call to move one column up then one row is also correct.
Step by step
Solved in 3 steps with 1 images

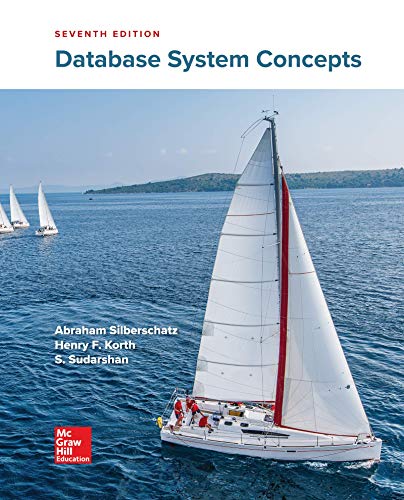
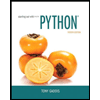
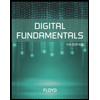
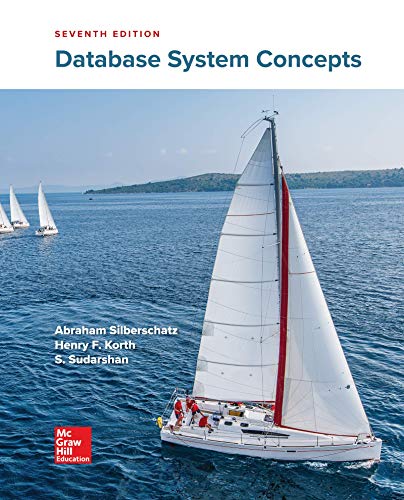
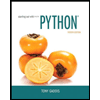
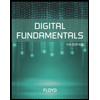
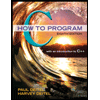
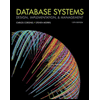
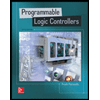