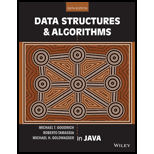
In the Towers of Hanoi puzzle, we are given a platform with three pegs, a, b, and c, sticking out of it. On peg a is a stack of n disks, each larger than the next, so that the smallest is on the top and the largest is on the bottom. The puzzle is to move all the disks from peg a to peg c, moving one disk at a time, so that we never place a larger disk on top of a smaller one. See Figure 5.15 for an example of the case n =4. Describe a recursive
Figure 5.15: An illustration of the Towers of Hanoi puzzle.

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Data Structures and Algorithms in Java
Additional Engineering Textbook Solutions
Concepts Of Programming Languages
Starting Out with C++ from Control Structures to Objects (9th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Electric Circuits. (11th Edition)
Starting Out With Visual Basic (8th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
- you can select multipy optionsarrow_forwardFor each of the following, decide whether the claim is True or False and select the True ones: Suppose we discover that the 3SAT can be solved in worst-case cubic time. Then it would mean that all problems in NP can also be solved in cubic time. If a problem can be solved using Dynamic Programming, then it is not NP-complete. Suppose X and Y are two NP-complete problems. Then, there must be a polynomial-time reduction from X to Y and also one from Y to X.arrow_forwardMaximum Independent Set problem is known to be NP-Complete. Suppose we have a graph G in which the maximum degree of each node is some constant c. Then, is the following greedy algorithm guaranteed to find an independent set whose size is within a constant factor of the optimal? 1) Initialize S = empty 2) Arbitrarily pick a vertex v, add v to S delete v and its neighbors from G 3) Repeat step 2 until G is empty Return S Yes Noarrow_forward
- Please help me answer this coding question in the images below for me(it is not a graded question):write the code using python and also provide the outputs requiredarrow_forwardWhat does the reduction showing Vertex Cover (VC) is NP-Complete do: Transforms any instance of VC to an instance of 3SAT Transforms any instance of 3SAT to an instance of VC Transforms any instance of VC to an instance of 3SAT AND transforms any instance of 3SAT to an instance of VC none of the abovearrow_forwardPlease assist me by writing out the code with its output (in python) using the information provided in the 2 images below.for the IP Address, it has been changed to: 172.21.5.204the serve code has not been open yet though but the ouput must be something along these lines(using command prompt):c:\Users\japha\Desktop>python "Sbongakonke.py"Enter the server IP address (127.0.0.1 or 172.21.5.199): 172.21.5.204Enter your student number: 4125035Connected to server!It's your turn to pour! Enter the amount to your pour (in mL):Please work it out until it gets the correct outputsNB: THIS QUESTION IS NOT A GRADED QUESTIONarrow_forward
- need help with a html code and css code that will match this image.arrow_forwardneed help with a html code and css code that will match this image. Part B - A Navigation Part B is the navigation component of a page. Information you need includes: Color Codes: Visiting links: #ff6666 Unvisited links: #ccff66 Hovered links: white Search box: #2ec4b6 rebeccapurple white Font: Google Font (Roboto) Icons: Font Awesome (fa-quidditch, fa-search) This is a flexbox based navigation menu. Other then padding, all spacing/positioning should be controlled using flex properties. The home link in the nav should point to your assignment file (to triggers visited styling). In the "state" screenshot below, Home is visited, Services is hovered (the mouse doesn't show up in the screenshot) and Products is unvisited.arrow_forwardMGMT SS STATS, an umbrella body that facilitates and serves various Social Security Organizations/Departments within the Caribbean territories, stood poised to meet the needs of its stakeholders by launching an online database. The database will provide members and the public access to the complete set of services that can (also) be initiated face-to-face, and it will provide managed, private, secure access to a repository of public and/or personal information. Ideally, the database will have basic details of pension plans recorded in the registry, member plan statistics, and cash inflows and outflows from pension funds.For example, insured persons accumulate contributions. Records for these persons will include information on the insured persons able to acquire various benefits once work is interrupted due to sickness, death, retirement, and maternity or employment injury. They will also include information on pensions such as invalidity, disability, and survivors that stem from one…arrow_forward
- Why all appvif i want to sign in its required phone number why not using google or apple its make me frustratedarrow_forwardWhy is the accuracy of time important in data visualizations? Detail a scenario from your professional experience in which time was structured poorly in a data visualization. How did this affect the understanding of the data presented? How do you think this error or oversight occurred?arrow_forwardWrite the KeanStudent class. The UML diagram of the class is represented below: KeanStudent - fullName: String - keanID: int -keanEmailAddress: String cellPhoneNumber: String + numberOfStudent: int + KeanStudent() + KeanStudent(fullName: String, keanID: int, keanEmailAddress: String, cellPhoneNumber: String) +getFullName(): String +setFullName(newFullName: String): void +getKeanIDO): int +getKeanEmailAddress(): String +getCellPhoneNumber(): String + setCellPhoneNumber(newCellPhoneNumber: String): void +toString(): String 1. Implement the KeanStudent class strictly according to its UML one-to-one (do not include anything extra, do not miss any data fields or methods) 2. Implement a StudentTest class to test the class KeanStudent you just created. • Create two KeanStudent objects using a no-args constructor and one from the constructor with all fields. o Print the contents of both objects. 。 Print numberOfStudent. 3. Add comments to your program (mark where data fields, constructors,…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningOperations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks ColeC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
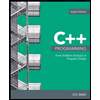
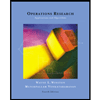
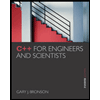
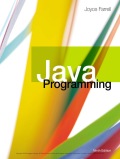
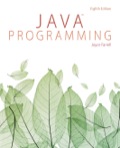