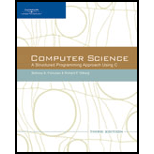
Explanation of Solution
Given: The following code:
// Header files.
#include
// Defining the function.
int main()
{
//Function declaration
int funA (int x);
int funB (int x);
//calling the main Function
int main(void)
{
//Local declarations
int a;
int b;
int c;
//Statements
a=10;
funB(a);
b=5;
c=funA(b);
funB(c);
printf("%3d %3d %3d", a, b, c);
return 0;
}
int funA (int x)
{
//Statements
return x*x;
}
void funB (int x)
{
//Local declarations
int y;
//Statements
y=x%2;
x/=2;
printf("\n%3d %3d\n", x, y);
return;
}
To find:Â The structure chart for the following code.
#include

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Computer Science: A Structured Programming Approach Using C, Third Edition
- Show all the workarrow_forwardShow all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forward
- Question 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forwardGenerate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forward
- I need help to solve the following, thank youarrow_forwardreminder it an exercice not a grading work GETTING STARTED Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website. Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”. If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically. With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet. If cell B6 does not display your name, delete the file and download a new copy from the SAM website. Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…arrow_forwardNeed help completing this algorithm here in coding! 2arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
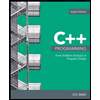
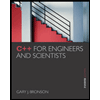
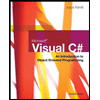
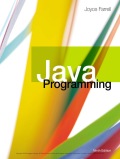
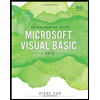