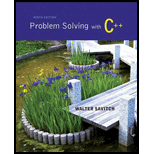
A liter is 0.264179 gallons. Write a

- Include required header files.
- Declare and initialize a constant value “lpg = 0.264179”.
- Define a function named “calc()” to calculate “milage”.
- Declare a variable “gal”.
- Compute “gal” and “milage”
- Function to return “milage”.
- Define a “main()” function.
- Declare the variables “lit” and “miles”.
- Declare a variable “ch”.
- “do… while” loop to get the user input repeatedly.
- Get the “lit” and “miles” from the user.
- Call “calc()” with an arguments “lit” and “miles” values and print the “milage”.
- Get the user input to repeat the program or not.
- The input is checked with the condition and repeat or exit the program.
Explanation of Solution
Program to compute number of miles per gallon the car delivered:
// Include required header files
#include <iostream>
using namespace std;
// Assign const value
float const lpg=0.264179;
// Function definition of calc()
float calc(float liters, float miles)
{
// Declare gal
float gal;
// Compute gal
gal = lpg * liters;
// Compute milage
float milage = miles/gal;
// Return milage
return(milage);
}
// Function definition of main()
int main()
{
// Declare lit and miles
float lit, miles;
// Declare ch
char ch;
// do... while loop
do{
// Get the liters
cout<<"\nEnter the number of liters of gasoline: ";
// Assign the user input to lit
cin>>lit;
// Get the miles
cout<<"\nEnter the number of miles Travelled: ";
// Assign the user input to miles
cin>>miles;
// Display the miles per gallon the car delivered
cout<<"\nNumber of miles per gallon the car delivered: ";
// Call the function cal() and return the result
cout<< calc(lit, miles) << endl;
// Get the user input
cout<<"\nDo you want to repeat(y/n)??: ";
// Assign the user input to ch
cin>>ch;
// While loop condition to check ch is equal to y
}while(ch=='y' || ch=='Y');
// Return 0
return 0;
}
Enter the number of liters of gasoline: 5
Enter the number of miles Travelled: 30
Number of miles per gallon the car delivered: 22.7119
Do you want to repeat(y/n)??: n
Want to see more full solutions like this?
Chapter 4 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
SURVEY OF OPERATING SYSTEMS
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Java: An Introduction to Problem Solving and Programming (8th Edition)
- Please answer Java OOP Questions.arrow_forward.NET Interactive Solving Sudoku using Grover's Algorithm We will now solve a simple problem using Grover's algorithm, for which we do not necessarily know the solution beforehand. Our problem is a 2x2 binary sudoku, which in our case has two simple rules: •No column may contain the same value twice •No row may contain the same value twice If we assign each square in our sudoku to a variable like so: 1 V V₁ V3 V2 we want our circuit to output a solution to this sudoku. Note that, while this approach of using Grover's algorithm to solve this problem is not practical (you can probably find the solution in your head!), the purpose of this example is to demonstrate the conversion of classical decision problems into oracles for Grover's algorithm. Turning the Problem into a Circuit We want to create an oracle that will help us solve this problem, and we will start by creating a circuit that identifies a correct solution, we simply need to create a classical function on a quantum circuit that…arrow_forwardNeed help with this in python!arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
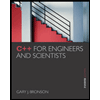
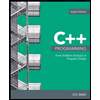
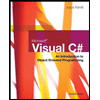