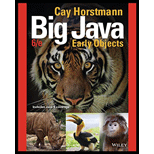
Given program:
//Define the class "BankAccount"
public class BankAccount
{
//Declare required variables
private double balance;
// Define a default constructor
public BankAccount
{
//Initialize the balance amount
balance=0;
}
// Define the parametrized constructor
public BankAccount(double initialBalance)
{
//Initialize the balance amount
balance=initialBalance;
}
//Give function definition to deposit amount
public void deposit(double amount)
{
//Compute the balance
balance=balance+amount;
}
//Give function definition to withdraw amount
public void withdraw(double amount)
{
//Compute the balance
balance=balance-amount;
}
//Give function definition to return the balance amount
public double getBalance()
{
//Return statement
return balance;
}
}
Explanation:
In the above program,
- Define a class “BankAccount”.
- Declare the variable “balance”.
- Define a default constructor.
- Initialize the balance amount.
- Define a parameterized constructor.
- Initialize the balance amount.
- Give the function definition “deposit()” to withdraw amount from the account.
- Compute the balance amount.
- Give the function definition “withdraw()” to withdraw amount from the account.
- Compute the balance amount
- Give function definition to return the balance amount.
- Return “balance”.

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Big Java, Binder Ready Version: Early Objects
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
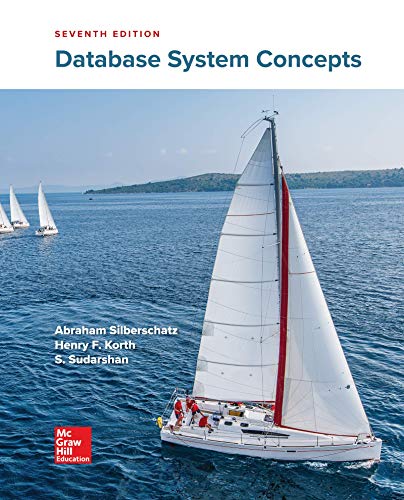
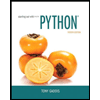
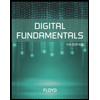
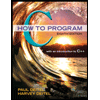
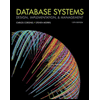
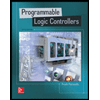