Concept explainers
For a function with prototype
long decoda2(long x, long y, long z);
GCC generates the following assembly code:
Parameters x, y, and z are passed in registers %rdi, %rsi, and %rdx. The code stores the return value in register %rax.
Write C code for decode2 that will have an effect equivalent to the assembly code shown.

Explanation of Solution
Given assembly code:
x in %rdi, y in %rsi and z in %rdx
decode2:
subq %rdx, %rsi
imulq %rsi, %rdi
movq %rsi, %rax
salq $63, %rax
sarq $63, %rax
xorq %rdi, %rax
ret
Load Effective Address:
- The load effective address instruction “leaq” is a variant of “movq” instruction.
- The instruction form reads memory to a register, but memory is not been referenced at all.
- The first operand of instruction is a memory reference; the effective address is been copied to destination.
- The pointers could be generated for later references of memory.
- The common arithmetic operations could be described compactly using this instruction.
- The operand in destination should be a register.
Data movement instructions:
- The different instructions are been grouped as “instruction classes”.
- The instructions in a class performs same operation but with different sizes of operand.
- The “Mov” class denotes data movement instructions that copy data from a source location to a destination.
- The class has 4 instructions that includes:
- movb:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 1 byte data size.
- movw:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 2 bytes data size.
- movl:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 4 bytes data size.
- movq:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 8 bytes data size.
- movb:
Comparison Instruction:
- The “CMP” instruction sets condition code according to differences of their two operands.
- The working pattern is same as “SUB” instruction but it sets condition code without updating destinations.
- The zero flag is been set if two operands are equal.
- The ordering relations between operands could be determined using other flags.
- The “cmpl” instruction compares values that are double word.
Unary and Binary Operations:
- The details of unary operations includes:
- The single operand functions as both source as well as destination.
- It can either be a memory location or a register.
- The instruction “incq” causes 8 byte element on stack top to be incremented.
- The instruction “decq” causes 8 byte element on stack top to be decremented.
- The details of binary operations includes:
- The first operand denotes the source.
- The second operand works as both source as well as destination.
- The first operand can either be an immediate value, memory location or register.
- The second operand can either be a register or a memory location.
Corresponding C code:
// Define method decode
long decode(long x, long y, long z)
{
// Declare variable
long tmp = y - z;
//Return
return (tmp * x)^(tmp << 63 >> 63);
}
Explanation:
- The register “%rdi” has value for “x”, register “%rsi” has value for “y” and register “%rdx” has value for “z”.
- The details of assembly code is shown below:
- The instruction “subq %rdx, %rsi” performs operation “y - z” and stores result in register “%rsi”.
- The statement “long tmp = y - z” corresponds to C code.
- The instruction “imulq %rsi, %rdi” multiplies result of operation with “x” and stores result in register “%rdi”.
- The statement “(tmp * x)” corresponds to C code.
- The instruction “movq %rsi, %rax” moves value in register “%rsi” to register “%rax”.
- The instruction “salq $63, %rax” performs left shift on value in register “%rax”.
- The statement “tmp << 63” corresponds to C code.
- The instruction “sarq $63, %rax” performs right shift on value in register “%rax”.
- The statement “tmp << 63 >> 63” corresponds to C code.
- The instruction “xorq %rdi, %rax” performs “XOR” operation on values in registers “%rax” and “%rdi”.
- The statement “return (tmp * x)^(tmp << 63 >> 63)” corresponds to C statement.
- The instruction “subq %rdx, %rsi” performs operation “y - z” and stores result in register “%rsi”.
Want to see more full solutions like this?
Chapter 3 Solutions
COMPUTER SYSTEMS&MOD MSGT/ET SA AC PKG
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Degarmo's Materials And Processes In Manufacturing
Starting Out with C++: Early Objects (9th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Java: An Introduction to Problem Solving and Programming (8th Edition)
- Question#2: Design and implement a Java program using Abstract Factory and Singleton design patterns. The program displays date and time in one of the following two formats: Format 1: Date: MM/DD/YYYY Time: HH:MM:SS Format 2: Date: DD-MM-YYYY Time: SS,MM,HH The following is how the program works. In the beginning, the program asks the user what display format that she wants. Then the program continuously asks the user to give one of the following commands, and performs the corresponding task. Note that the program gets the current date and time from the system clock (use the appropriate Java date and time operations for this). 'd' display current date 't': display current time 'q': quit the program. • In the program, there should be 2 product hierarchies: "DateObject” and “TimeObject”. Each hierarchy should have format and format2 described above. • Implement the factories as singletons. • Run your code and attach screenshots of the results. • Draw a UML class diagram for the program.arrow_forward#include <linux/module.h> #include <linux/kernel.h> // part 2 #include <linux/sched.h> // part 2 extra #include <linux/hash.h> #include <linux/gcd.h> #include <asm/param.h> #include <linux/jiffies.h> void print_init_PCB(void) { printk(KERN_INFO "init_task pid:%d\n", init_task.pid); printk(KERN_INFO "init_task state:%lu\n", init_task.state); printk(KERN_INFO "init_task flags:%d\n", init_task.flags); printk(KERN_INFO "init_task runtime priority:%d\n", init_task.rt_priority); printk(KERN_INFO "init_task process policy:%d\n", init_task.policy); printk(KERN_INFO "init_task task group id:%d\n", init_task.tgid); } /* This function is called when the module is loaded. */ int simple_init(void) { printk(KERN_INFO "Loading Module\n"); print_init_PCB(); printk(KERN_INFO "Golden Ration Prime = %lu\n", GOLDEN_RATIO_PRIME); printk(KERN_INFO "HZ = %d\n", HZ); printk(KERN_INFO "enter jiffies = %lu\n", jiffies); return 0; } /* This function is called when the…arrow_forwardList at least five Operating Systems you know. What is the difference between the kernel mode and the user mode for the Linux? What is the system-call? Give an example of API in OS that use the system-call. What is cache? Why the CPU has cache? What is the difference between the Static Linking and Dynamic Linking when compiling the code.arrow_forward
- In the GoF book, List interface is defined as follows: interface List { int count(); //return the current number of elements in the list Object get(int index); //return the object at the index in the list Object first(); //return the first object in the list Object last(); //return the last object in the list boolean include(Object obj); //return true is the object in the list void append(Object obj); //append the object to the end of the list void prepend(Object obj); //insert the object to the front of the list void delete(Object obj); //remove the object from the list void deleteLast(); //remove the last element of the list void deleteFirst(); //remove the first element of the list void deleteAll(); //remove all elements of the list (a) Write a class adapter to adapt Java ArrayList to GoF List interface. (b) Write a main program to test your adapters through List interface. (c) Same requirement as (a) and (b), but write an object adapter to adapt Java ArrayList to GoF List…arrow_forwardIn modern packet-switched networks, including the Internet, the source host segments long, application-layer messages (for example, an image or a music file) into smaller packets and sends the packets into the network. The receiver then reassembles the packets back into the original message. We refer to this process as message segmentation. Figure 1.27 (attached) illustrates the end-to-end transport of a message with and without message segmentation. Consider a message that is 106 bits long that is to be sent from source to destination in Figure 1.27. Suppose each link in the figure is 5 Mbps. Ignore propagation, queuing, and processing delays. a. Consider sending the message from source to destination without message segmentation. How long does it take to move the message from the source host to the first packet switch? Keeping in mind that each switch uses store-and-forward packet switching, what is the total time to move the message from source host to destination host? b. Now…arrow_forwardConsider a packet of length L that begins at end system A and travels over three links to a destination end system. These three links are connected by two packet switches. Let di, si, and Ri denote the length, propagation speed, and the transmission rate of link i, for i = 1, 2, 3. The packet switch delays each packet by dproc. Assuming no queuing delays, in terms of di, si, Ri, (i = 1, 2, 3), and L, what is the total end-to-end delay for the packet? Suppose now the packet is 1,500 bytes, the propagation speed on all three links is 2.5 * 10^8 m/s, the transmission rates of all three links are 2.5 Mbps, the packet switch processing delay is 3 msec, the length of the first link is 5,000 km, the length of the second link is 4,000 km, and the length of the last link is 1,000 km. For these values, what is the end-to-end delay?arrow_forward
- how to know the weight to data and data to weight also weight by infomraion gain in rapid miner , between this flow diagram retrieve then selecte attrbuite then set role and split data and decision tree and apply model and peformance ,please show how the operators should be connected:arrow_forwardusing rapid miner how to creat decison trea for all attribute and another one with delete one or more of them also how i know the weight of each attribute and what that mean in impact the resultarrow_forwardQ.1. Architecture performance [10 marks] Answer A certain microprocessor requires either 2, 4, or 6 machine cycles to perform various operations. ⚫ (40+g+f)% require 2 machine cycles, ⚫ (30-g) % require 4 machine cycles, and ⚫ (30-f)% require 6 machine cycles. (a) What is the average number of machine cycles per instruction for this microprocessor? Answer (b) What is the clock rate (machine cycles per second) required for this microprocessor to be a "1000 MIPS" processor? Answer (c) Suppose that 35% of the instructions require retrieving an operand from memory which needs an extra 8 machine cycles. What is the average number of machine cycles per instruction, including the instructions that fetch operands from memory?arrow_forward
- Q.2. Architecture performance [25 marks] Consider two different implementations, M1 and M2, of the same instruction set. M1 has a clock rate of 2 GHz and M2 has a clock rate of 3.3 GHz. There are two classes of instructions with the following CPIs: Class A CPI for M1 CPI for M2 2.f 1.g B 5 3 C 6 4 Note that the dots in 2 fand 1.g indicate decimal points and not multiplication. a) What are the peak MIPS performances for both machines? b) Which implementation is faster, if half the instructions executed in a certain program are from class A, while the rest are divided equally among classes B and C. c) What speedup factor for the execution of class-A instructions would lead to 20% overall speedup? d) What is the maximum possible speedup that can be achieved by only improving the execution of class-A instructions? Explain why. e) What is the clock rate required for microprocessor M1 to be a "1000 MIPS" (not peak MIPS) processor?arrow_forwardPLEASE SOLVE STEP BY STEP WITHOUT ARTIFICIAL INTELLIGENCE OR CHATGPT I don't understand why you use chatgpt, if I wanted to I would do it myself, I need to learn from you, not from being a d amn robot. SOLVE STEP BY STEP I WANT THE DIAGRAM PERFECTLY IN SIMULINKarrow_forwardI need to develop and run a program that prompts the user to enter a positive integer n, and then calculate the value of n factorial n! = multiplication of all integers between 1 and n, and print the value n! on the screen. This is for C*.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
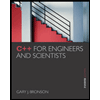
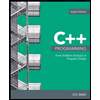
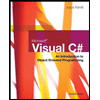
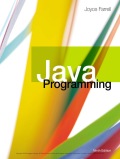
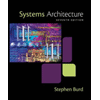