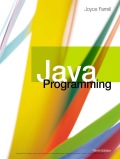
EBK JAVA PROGRAMMING
9th Edition
ISBN: 9781337671385
Author: FARRELL
Publisher: CENGAGE LEARNING - CONSIGNMENT
expand_more
expand_more
format_list_bulleted
Question
Chapter 3, Problem 1PE
Program Plan Intro
Java Method calling:
- The java method is a collection of statements used to perform certain operations.
- The method should be called in order to use it. The methods can be called in two ways,
- Method returning a value
- Methods returning nothing
- The method calling process is very simple. When calling a method, the program control gets transferred to the called method.
- The above stated called method returns control to the caller in two separate conditions. That are,
- The return statement is executed.
- It reaches the closing brace of the method.
- The arguments of the method call should be same as in the definition of the method.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
11
use only C# programming
Write a method call CubeIt(int x, ref int cube) that takes two arguments and does not return a value. The method will cube the first argument and assign it to the second argument.In your main, call this method twice and print the value of the parameters after each method call.
Write a method with the following header: static void CalculateTuitionFee(int numberOfCourses, double costPerCourse, ref double fees). This method will calculate and assign the required fees amount to the third argument. [Fees = number of courses * cost per course + 15.25].From your program Main() method, call the CalculateTuitionFee () method four times supplying different arguments each time and display the value of the third argument after each method call.
Write a method that takes four parameter of type int. The method will assign the sum of the first two arguments to the third and the difference of the first two to the fourth. This method should be coded so that the calling…
3) The following method should return true if the int parameter is even and either positive or 0, and false otherwise.Which set of code should you use to replace … so that the method works appropriately?public boolean question3(int x) { … }a) if (x = = 0) return true; else if (x < 0) return false; else return question3(x – 1);b) if (x = = 0) return false; else if (x < 0) return true; else return question3(x – 1);c) if (x = = 0) return true; else if (x < 0) return false; else return question3(x – 2);d) if (x = = 0) return false; else if (x < 0) return true; else return question3(x – 2);e) return(x = = 0);
Chapter 3 Solutions
EBK JAVA PROGRAMMING
Ch. 3 - Prob. 1RQCh. 3 - Prob. 2RQCh. 3 - Prob. 3RQCh. 3 - Prob. 4RQCh. 3 - Prob. 5RQCh. 3 - Prob. 6RQCh. 3 - Prob. 7RQCh. 3 - Prob. 8RQCh. 3 - Prob. 9RQCh. 3 - Prob. 10RQ
Ch. 3 - Prob. 11RQCh. 3 - Prob. 12RQCh. 3 - Prob. 13RQCh. 3 - Prob. 14RQCh. 3 - Prob. 15RQCh. 3 - Prob. 16RQCh. 3 - Prob. 17RQCh. 3 - Prob. 18RQCh. 3 - Prob. 19RQCh. 3 - Prob. 20RQCh. 3 - Prob. 1PECh. 3 - Prob. 2PECh. 3 - Prob. 3PECh. 3 - Prob. 4PECh. 3 - Prob. 5PECh. 3 - Prob. 6PECh. 3 - Prob. 7PECh. 3 - Prob. 8PECh. 3 - Prob. 9PECh. 3 - Prob. 10PECh. 3 - Prob. 11PECh. 3 - Prob. 12PECh. 3 - Prob. 13PECh. 3 - Prob. 1GZCh. 3 - Prob. 2GZCh. 3 - Prob. 1CP
Knowledge Booster
Similar questions
- Where do I place a return method for finding the area of a square, triangle, and rectangle? Also are the math equations correct for each shape? //PSEUDOCODE //Write a program that demonstrates method overloading by defining and calling methods that return the area //of a triangle //a rectangle //a square private static void area(float a) { System.out.println("The area of the square is " + String.format("%,.2f", Math.pow(a,2)) + " sq units"); } private static void area1 (float a, float b) { System.out.println("The area a of the rectangle is " + String.format("%,.2f", a * b) + " sq units"); } private static void area (float a, float b) { double c = 0.5 * (a * b); System.out.println("The area of the triangle is " + String.format("%,.2f", c) + " sq units"); } public static void main(String[] args) { area(5); area1(05,21); area(05,05); } }arrow_forwardExamine the following method header; then write an example call to the method. private void ResetValue(ref int value)arrow_forward5. If you have the following methods in a class, will the code compile? public void drawHex (int x, double y, String s) public void drawHex (double x, int y, String s) public void drawHex (String x, double y, int s) public void drawHex (int y, int x, int s) A. B. C. No Yes There is no way to tellarrow_forward
- QUESTION 10 Multiple Choice: What is the return type of the following method? public static void widget(double a, String b){ ... } String void int double QUESTION 11 Multiple Choice: Which statement is true about the method below? public static String doStuff(int x, double n, boolean b){ ... } String is the return type of this method int x, doublen, and boolean b will cause a syntax error and should be moved to the method body public and static are identifiers for the method O doStuff is a modifier for the method QUESTION 12arrow_forwardJAVA PROGRAM SEE ATTACHED PHOTO FOR THE PROBLEMarrow_forwardpublic static int cube(int num { return num num num; } What is the correct statement to pass value 8 to this method and assigns its return value to a variable named result? O cube(8) = int result; int result = cube(8) O int result = O int result = int cube(8);arrow_forward
- Examine the method header below, then write a call to the method as an example.a personal void ShowValue()arrow_forwardA return statement is the same as a print statement. Anything that a method returns will automatically get printed. True Falsearrow_forwardConsider the following methods: public void inchesToCentimeters(double i) { double c = i * 2.54; printInCentimeters(i, c); } public void printInCentimeters(double inches, double centimeters) { System.out.print(inches + "-->" + centimeters); } Assume that the method call inchesToCentimeters(10) appears in a method in the same class. What is printed as a result of the method call? inches -> centimeters 10 -> 25 25.4 -> 10 10 -> 12.54 10.0 -> 25.4arrow_forward
- In Java languagearrow_forwardGiven the method header as follow: public static void myMethod(int a, int b, int c) The following statement is correct, EXCEPT O This type of method must not have the 'return' keyword O When the following call to "myMethod" is execute: "myMethod(3.5, 2.1, 1.0);" value a will store 3.5, value b will store 2.1, and value c will store 1.0 O To display the value of a, b, and c, the body of myMethod should have System.out.print(a+" "+ "+b+" "+c); O When the following call to "myMethod" is execute: "myMethod(3, 2, 1);" value a will store 3, value b will store 2, and value c will store 1arrow_forward1- question Given the following method; public static void mystery(int x) { int z = 10; if (Z <= x) Z--; z++; System.out.println(z); } What is the output of mystery(30)? a. 9 b. 10 C. 29 d. 30arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
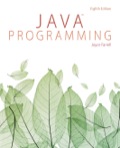
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
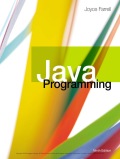
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
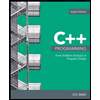
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning