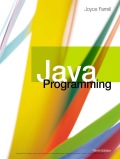
Explanation of Solution
a.
Program code:
Lease.java
//define the class Lease
public class Lease
{
//declare class members
private static final double PET_FEE = 10;
private String tenantName;
private int apartmentNumber;
private double monthlyRent;
private int leasePeriod;
//Default constructor
public Lease()
{
//initialize the class members
tenantName ="XXX";
apartmentNumber =0;
monthlyRent = 1000;
leasePeriod =12;
}
//getters and setters
//define a method getTenantName()
public String getTenantName()
{
//return the variable tenantName
return tenantName;
}
//define a method setTenantName()
public void setTenantName(String tenantName)
{
//set the value of tenantName
this.tenantName = tenantName;
}
//define a method getApartmentNumber()
public int getApartmentNumber()
{
//return the variable apartmentNumber
return apartmentNumber;
}
//define a method setApartmentNumber()
public void setApartmentNumber(int apartmentNumber)
{
//set the value of apartmentNumber
this.apartmentNumber = apartmentNumber;
}
//define a method getMonthlyRent()
public double getMonthlyRent()
{
//return the variable monthlyRent
return monthlyRent;
}
//define a method setMonthlyRent()
public void setMonthlyRent(double monthlyRent)
{
//set the value of monthlyRent
this.monthlyRent = monthlyRent;
}
//define a method getLeasePeriod()
public int getLeasePeriod()
{
//return the variable leasePeriod
return leasePeriod;
}
//define a method setLeasePeriod()
public void setLeasePeriod(int leasePeriod)
{
//set the value of leasePeriod
this.leasePeriod = leasePeriod;
}
//define a method addPetFee()
public void addPetFee()
{
//adds $10
monthlyRent+=PET_FEE;
}
//define a method explainPetPolicy()
public static void explainPetPolicy()
{
//print the statement
System.out.println("Add $10 to rent as pet fee.");
}
}
Explanation:
The above snippet of code is used create a class “Lease”. The class contain different static methods for store the details of a lease. In the code,
- Define a class “Lease”
- Declare the class members.
- Define the constructor “Lease()” method.
- Initialize the class members.
- Define the “getTenantName()” method.
- Return the value of the variable “tenantName”.
- Define the “setTenantName()” method.
- Set the value of the variable “tenantName”.
- Define the “getApartmentNumber()” method.
- Return the value of the variable “apartmentNumber”.
- Define the “setApartmentNumber()” method.
- Set the value of the variable “ApartmentNumber”.
- Define the “getMonthlyRent()” method.
- Return the value of the variable “MonthlyRent”.
- Define the “setMonthlyRent ()” method.
- Set the value of the variable “MonthlyRent”.
- Define the “getLeasePeriod()” method.
- Return the value of the variable “leasePeriod”.
- Define the “setLeasePeriod()” method.
- Set the value of the variable “leasePeriod”.
- Define the “addPetFree()” method.
- Set the value of “monthlyRent”.
- Define the “explainPetPolicy()” method.
- Print the statement.
b.
TestLease.java
//import the packages
import java.text.NumberFormat;
import java.util.Scanner;
//define a class TestLease
public class TestLease
{
//define main() method
public static void main(String[] args)
{
//declare the objects of the class Lease
Lease lease1 = new Lease();
Lease lease2 = new Lease();
Lease lease3 = new Lease();
Lease lease4 = new Lease();
//Call three times getdata()
lease1 = getData();
lease2 = getData();
lease3 = getData();
System.out.print("Display info of tenants\n\n");
//Print info
showValues(lease1);
showValues(lease2);
showValues(lease3);
showValues(lease4);
System...

Trending nowThis is a popular solution!

Chapter 3 Solutions
EBK JAVA PROGRAMMING
- Instructions This assignment must follow directions exactly. Create a class Lab02 with a main method, and put all of the following code into the main method: Print the prompt shown below and ask the user for the number of exemptions. The number of exemptions is an integer. Print the prompt shown below and ask the user for their gross salary. The gross salary represents dollars, which can be entered with or without decimal points. Print the prompt shown below and ask the user for their interest income. The interest income represents dollars, which can be entered with or without decimal points. Print the prompt shown below and ask the user for their capital gains income. The capital gains represents dollars, which can be entered with or without decimal points. Print the prompt shown below and ask the user for the amount of charitable contributions. The charitable contributions represents dollars, which can be entered with or without decimal points. Perform the calculation of…arrow_forwardPart A Create an Automobile class for a dealership. Include fields for an ID number (id), make (make), model (model), color (color), year (year), and miles per gallon (mpg). Include get and set methods for each field. Do not allow the ID to be negative or more than 9999; if it is, set the ID to 0. Do not allow the year to be earlier than 2005 or later than 2024; if it is, set the year to 0. Do not allow the miles per gallon to be less than 10 or more than 60; if it is, set the miles per gallon to 0. Include a default constructor that accepts no arguments and an overloaded constructor that accepts arguments for each field value and uses the set methods to assign the values. Part B Write an application called TestAutomobiles that declares two Automobile objects. Write a function called enterData() that prompts the user for the data values for an Automobile object and returns that object. When you test the program, be sure to enter some invalid data to demonstrate that all the methods…arrow_forwardIt has been realized that hackers have started hacking and changing the account detains of some banks, to stop this practice, you have been tasked to create a class called Abaaneke, your class should contain three variables (deposit, oldBalance, newBalace) all of type float and a variable of type String (name of the account holder) Your class should also have a method that takes three arguments – name of account holder, amount deposited and old balance. The method should add the deposit to the oldBalance and call it new balance. Using the right setters and getters, initialize the private variables and call the method to display the following in main a. Account holders name b. Amount deposited c. Old balance d. And new balancearrow_forward
- A. Build a class named car. This class is defined as follows: It has the fields: Car ID, Car model, Car make, Car color, Car year. Build a constructor that accepts the five parameters (Car ID, Car model, Car make, Car color, Car year). Override the method toString() to return the string representation of Car ID, Car model, Car make, Car color, Car year. Build a method named display to print the values returned by toString() method. Override the equals() method to compare the Car model, Car make, Car color of two Car objects. The method returns true if all the values of these fields are the same, and false otherwise. In the equals method you must check the following: a. If you compare the Car object to itself, return true.! b. If you compare car object with another car object return true, otherwise return false. Hint use : instanceof operator for this purpose. Override the hashcode() method to return same hash codes for objects that are equal according to the equals() method you built…arrow_forwardCreate a Class Pet with the following data members Identification: String species : String (e.g. cat, dog, fish etc) breed: String Age (in days): int Weight: float Dead: boolean 1. Provide a constructor with parameters for all instance variables. 2. Provide getters for all and setters for only breed, and weight 3. Provide a method growOld() that increases the age of the pet by one day. A dead pet wont grow old 4. Provide a method growHealthy(float w) that increases the weight of the pet by the given amount w. A dead pet cannot grow healthy. 5. Provide a method fallSick(float w) that reduces the weight of the pet by the given amount. The least weight a pet can have is 0 which will mean that the pet has died. If the value of weigh is 10 kg and the method is called with an argument of 11 kg then you will set it to 0 and set the dead to an appropriate value to mark the death of the pet 6. Provide a toString method that shows an appropriate well formatted string…arrow_forwardWrite a program that test methods in the class. Hint: the employee deserved retirement if he has year of Service >25 or his age >=65. 5. Design a class named Mylnteger. The class contains: An int data field named value(int) Methods isEven() and isOdd(0 that return true if the value is even or odd respectively. Write a program that test methods in the class.arrow_forward
- Create a class named Person.a. This class must have two variables to store name (string) and age (int). These variables should not be accessible from outside the class.b. Encapsulate the variables defined in item a with a property (an intermediate variable) so that they can be accessed from other classes. And after this step, use only intermediate variables that you defined for encapsulation within methods and other classes.c. Define a constructor method for this class. Code the constructor method to initialize the properties of the class at the time of object definition.D. Define a method called Print Info that will print the information of the class object on the screen in a proper format.arrow_forwardModify this class by: 1. Suppose the bank wants to keep track of how many accounts exist. A. Do you need a static variable or a regular variable to save how many accounts have been created? Declare it and name the variable as numAccounts. It should be initialized to 0 (since no account exists initially). B. Update this variable in the constructor. (Ask the instructor if you do not know how) C. Add a method getNumAccounts that returns the total number of accounts. Decide if this method should be static – is the information related to a single account or is it for the entire class? Does this method manipulate a static variable? Complete this method. 2. Use the driver class TestAccounts1.java to test your modified Account class. Complete the code as instructed. 3. Add a method void close() to your Account class. This method should close the current account by appending “CLOSED” to the account name and setting the balance to 0. Also, decrement the total number of accounts. Should this…arrow_forwardPlease help me with this Java Labarrow_forward
- Create a class named Purchase. Each Purchase contains an invoice number (private int), amount of sale (private double), and amount of sales tax (private double). Include set methods for the invoice number and sale amount. Within the set() method for the sale amount, calculate the sales tax as 5% of the sale amount. Also include a display method that displays a purchase’s details (i.e invoice, sale amount and tax). Save the file as Purchase.java. 2. Create an application (with main ()) that declares a Purchase object (using class defined in previous question) and prompts the user if he/she wants to enter an invoice number (1-yes, 0 – no). If user enters 1 then the program will prompt for an invoice number, do not let the user proceed until a number between 1,000 and 8,000 has been entered. Next, the program prompts for a sale amount, do not proceed until the user has entered a nonnegative value. Using the values entered by the user create a Purchase object, display the object’s…arrow_forwardCreate a class named Lease with fields that hold an apartment tenant’s name, apartmentnumber, monthly rent amount, and term of the lease in months. Include a constructor thatinitializes the name to “XXX”, the apartment number to 0, the rent to 1000, and the term to12. Also include methods to get and set each of the fields. Include a nonstatic methodnamed addPetFee() that adds $10 to the monthly rent value and calls a static methodnamed explainPetPolicy() that explains the pet fee. Save the class asLeaseYourlastname.java. Replace ‘Yourlastname’ with your real last name.b) Create a class named TestLease whose main() method declares four Lease objects. Call agetData() method three times. Within the method, prompt a user for values for each fieldfor a Lease, and return a Lease object to the main() method where it is assigned to one ofmain()’s Lease objects. Do not prompt the user for values for the fourth Lease object, but letit continue to hold the default values. Then, in main(), pass…arrow_forwardConstruct a class named Circle with attributes named radius, diameter and area. Include a constructor that sets the radius to 1 and calculates the other two values. Include get and set methods for all the attributes. The setRadius(0 method not only sets the radius, it also calculates the other two values. The diameter of a circle is twice the radius and the area of a circle is pi multiplied by the square of the radius. Use the Math class PI constant for this calculation.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
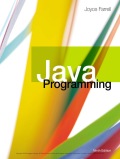
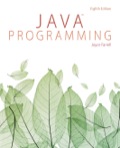
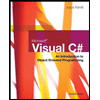