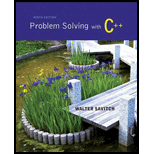
Write a

Program plan:
- Include necessary header files.
- Declare the namespace.
- Define the class “Player”.
- Declare the necessary functions within the “public” access specifier.
- Declare the necessary variables with the “private” access specifier.
- The initializer sets “Player::totWins” to “0”.
- Define the function “play()”.
- Print the statement.
- Get the input “choice” from the user.
- Call the function “toupper()” and assign the result into the variable “choice”.
- Define the function “Ch()”.
- Return the value of the variable “choice”.
- Define the function “AccWins()”.
- Return the value of the variable “totWins”
- Define the function “IncWins()”.
- Increment the value of the variable “totWins” by “1”.
- Declare the function “wins()”.
- Define the function “wins()”.
- The “if” loop check the expression.
- True, user 1 wins by calling the function “IncWins()”.
- Return “1”.
- The “else if” loop check the expression.
- True, user 2 wins by calling the function “IncWins()”.
- Return “2”.
- Otherwise, return zero.
- The “if” loop check the expression.
- Define the “main()” function.
- Create objects for the class “Player”.
- Initialize the variable.
- The “while” loop check the condition.
- True, objects call the function “play()”.
- Define the “switch” case.
- Define “case 0” for no winner.
- Define “case 1” for player 1 wins.
- Define “case 2” for player 2 wins.
- Call the function “toupper()” and assign the result in the variable “answer”.
- Return “0”.
Program to score the paper-rock-scissor game.
Explanation of Solution
Program:
//Include necessary header files
#include <iostream>
#include <cctype>
//Declare the namespace
using namespace std;
//Define the class Player
class Player
{
//Access specifier
public:
//Constructor, declare the function Player()
Player();
//Declare the function play()
void play();
//Declare the function Ch()
char Ch();
//Declare the function AccumulatedWins
int AccWins();
//Deeclare the function IncWins()
void IncWins();
//Access specifier
private:
//Variable declaration
char choice;
int totWins;
};
//Initializer sets Player::totWins to 0
Player::Player():totWins(0)
{
}
//Define the function play()
void Player::play()
{
//Print the statement
cout << "Please enter either R)Rock, P)Paper, or S)Scissor." << endl;
//Get the input from the user
cin >> choice;
//Call the function toupper() and assign the result in choice
choice = toupper(choice);
}
//Define the function Ch()
char Player::Ch()
{
//Return the value of the variable choice
return choice;
}
//Define the function AccWins()
int Player::AccWins()
{
//Return the value of the variable totWins
return totWins;
}
//Define the function IncWins()
void Player::IncWins()
{
//Increment the value of the variable totWins by 1
totWins++;
}
//Declare the function wins()
int wins(Player& user1, Player& user2);
//Define the function wins()
int wins(Player& user1, Player& user2 )
{
//Check, the expression
if( ( 'R' == user1.Ch() && 'S' == user2.Ch() )||
( 'P' == user1.Ch() && 'R' == user2.Ch() )||
( 'S' == user1.Ch() && 'P' == user2.Ch() ) )
{
//True, user 1 wins by calling the function IncWins()
user1.IncWins();
//Return 1
return 1;
}
//Check, the expression
else if( ( 'R' == user2.Ch() && 'S' == user1.Ch() )
|| ( 'P' == user2.Ch() && 'R' == user1.Ch() )
|| ( 'S' == user2.Ch() && 'P' == user1.Ch() ) )
{
//True, user 2 wins by calling the function IncWins()
user2.IncWins();
//Return 1
return 2;
}
//Otherwise
else
//Return zero, no winner
return 0;
}
//Define the main() function
int main()
{
//Create objects for the class Player
Player player1;
Player player2;
//Initialize the variable answer as Y
char answer = 'Y';
//Check, Y is equal to answer
while ('Y' == answer)
{
//True, the objects call the function play()
player1.play();
player2.play();
//Swich case
switch( wins(player1, player2) )
{
//Case 0 for no winner
case 0:
//Print the result
cout << "No winner. " << endl
<< "Totals to this move: " << endl
<< "Player 1: " << player1.AccWins()
<< endl
<< "Player 2: " << player2.AccWins()
<< endl
<< "Play again? Y/y continues other quits";
//Get the input from the user
cin >> answer;
//Print the statement
cout << "Thanks " << endl;
//Break the statement
break;
//Case 1 for player 1 wins
case 1:
//Pint the result
cout << "Player 1 wins." << endl
<< "Totals to this move: " << endl
<< "Player 1: " << player1.AccWins()
<< endl
<< "Player 2: " << player2.AccWins()
<< endl
<< "Play Again? Y/y continues, other quits. ";
//Get the input from the user
cin >> answer;
//Print the statement
cout << "Thanks " << endl;
//Break the statement
break;
//Case 2 for player 2 wins
case 2:
//Pint the result
cout << "Player 2 wins." << endl
<< "Totals to this move: " << endl
<< "Player 1: " << player1.AccWins()
<< endl
<< "Player 2: " << player2.AccWins()
<< endl
<< "Play Again? Y/y continues, other quits.";
//Get the input from the user
cin >> answer;
//Print the statement
cout << "Thanks " << endl;
//Break the statement
break;
}
/*Call the function toupper() and assign the result in the variable answer*/
answer = toupper(answer);
}
//Return zero
return 0;
}
Output:
Please enter either R)Rock, P)Paper, or S)Scissor.
R
Please enter either R)Rock, P)Paper, or S)Scissor.
S
Player 1 wins.
Total to this move:
Player 1: 1
Player 2: 0
Play Again? Y/y continues, other quits. Y
Thanks
Please enter either R)Rock, P)Paper, or S)Scissor.
P
Please enter either R)Rock, P)Paper, or S)Scissor.
S
Player 2 wins.
Total to this move:
Player 1: 1
Player 2: 1
Play Again? Y/y continues, other quits. Y
Thanks
Please enter either R)Rock, P)Paper, or S)Scissor.
S
Please enter either R)Rock, P)Paper, or S)Scissor.
R
Player 2 wins.
Total to this move:
Player 1: 1
Player 2: 2
Play Again? Y/y continues, other quits. N
Thanks
Want to see more full solutions like this?
Chapter 3 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Mechanics of Materials (10th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting Out with Python (4th Edition)
Database Concepts (8th Edition)
- Could you help me to know features of the following concepts: - defragmenting. - dynamic disk. - hardware RAIDarrow_forwardwhat is a feature in the Windows Server Security Compliance Toolkit, thank you.arrow_forwardYou will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forward
- Show all the workarrow_forwardShow all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forward
- Question 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forwardGenerate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
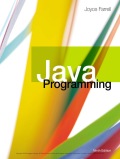
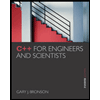
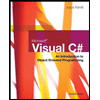
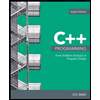
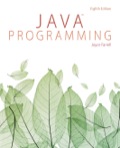