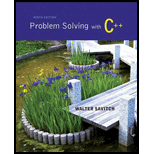
Concept explainers
What output will be produced by the following code, when embedded in a complete
int x = SOME_CONSTANT;
cout << “Start\n”;
if (x < 100)
cout << “First Output. \n”;
else if (x > 100)
cout << “Second Output. \n”;
else
cout << x << endl;
cout << “End\n”;
SOME_CONSTANT is a constant of type int. Assume that neither “First Output” nor “Second Output” is output. So, you know the value of x is output.

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Introduction To Programming Using Visual Basic (11th Edition)
Degarmo's Materials And Processes In Manufacturing
Mechanics of Materials (10th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
SURVEY OF OPERATING SYSTEMS
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
- 14. Show that the language L= {wna (w) < Nь (w) < Nc (w)} is not context free.arrow_forward7. What language is accepted by the following generalized transition graph? a+b a+b* a a+b+c a+b 8. Construct a right-linear grammar for the language L ((aaab*ab)*).arrow_forward5. Find an nfa with three states that accepts the language L = {a^ : n≥1} U {b³a* : m≥0, k≥0}. 6. Find a regular expression for L = {vwv: v, wЄ {a, b}*, |v|≤4}.arrow_forward
- 15. The below figure (sequence of moves) shows several stages of the process for a simple initial configuration. 90 a a 90 b a 90 91 b b b b Represent the action of the Turing machine (a) move from one configuration to another, and also (b) represent in the form of arbitrary number of moves.arrow_forward12. Eliminate useless productions from Sa aA BC, AaBλ, B→ Aa, C CCD, D→ ddd Cd. Also, eliminate all unit-productions from the grammar. 13. Construct an npda that accepts the language L = {a"b":n≥0,n‡m}.arrow_forwardYou are given a rope of length n meters and scissors that can cut the rope into any two pieces. For simplification, only consider cutting the rope at an integer position by the meter metric. Each cut has a cost associated with it, c(m), which is the cost of cutting the rope at position m. (You can call c(m) at any time to return the cost value.) The goal is to cut the rope into k smaller pieces, minimizing the total cost of cutting. B Provide the pseudo-code of your dynamic programming algorithm f(n,k) that will return the minimum cost of cutting the rope of length n into k pieces. Briefly explain your algorithm. What is the benefit of using dynamic programming for this problem? What are the key principles of dynamic programming used in your algorithm?arrow_forward
- Determine whether each of the problems below is NP-Complete or P A. 3-SAT B. Traveling Salesman Problem C. Minimum Spanning Tree D. Checking if a positive integer is prime or not. E. Given a set of linear inequalities with integer variables, finding a set of values for the variables that satisfies all inequalities and maximizes or minimizes a given linear objective function.arrow_forward1. Based on our lecture on NP-Complete, can an NP-Complete problem not have a polynomial-time algorithm? Explain your answer. 2. Prove the conjecture that if any problem in NP is not polynomial-time solvable, then no NP-Complete problem is polynomial-time solvable. (You can't use Theorem 1 and 2 directly) 3. After you complete your proof in b), discuss how this conjecture can be used to solve the problem of whether P=NP.arrow_forwardBased on our lectures and the BELLMAN-FORD algorithm below, answer the following questions. BELLMAN-FORD (G, w, s) 1 INITIALIZE-SINGLE-SOURCE (G, s) 2 for i = 1 to |G. VI - 1 3 4 5 6 7 8 for each edge (u, v) = G.E RELAX(u, v, w) for each edge (u, v) = G.E if v.d> u.d+w(u, v) return FALSE return TRUE 1. What does the algorithm return? 2. Analyze the complexity of the algorithm.arrow_forward
- (Short-answer) b. Continue from the previous question. Suppose part of the data you extracted from the data warehouse is the following. Identify the missing values you think exist in the dataset. Use Column letter and Row number to refer to each missing value in the dataset. Please write down how you want to address each particular missing value (you can group them if they receive same treatment). For imputation, you do not need to calculate the exact imputed values but just describe what kind of value you want to use to impute.arrow_forwardPlease original work Locate data warehousing solutions offered by IBM, Oracle, Microsoft, and Amazon Compare and contrast the capabilities of each solution and provide several names of some organizations that utilize each of these solutions. Please cite in text references and add weblinksarrow_forwardNeed Help: Which of the following statements about confusion matrix is wrong A) Confusion matrix is a performance measure for probability prediction techniques B) Confusion matrix is derived based on classification rules with cut-off value 0.5 C) Confusion matrix is derived based on training partition to measure a model’s predictive performance D) None of the abovearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
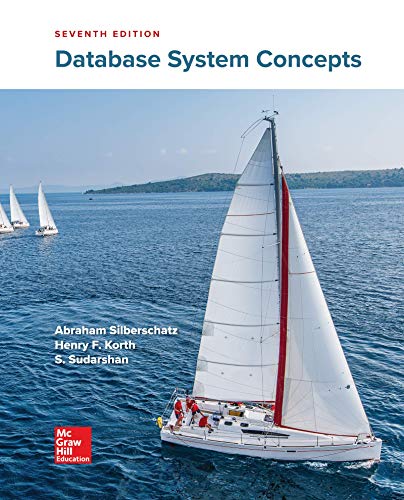
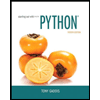
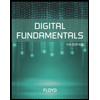
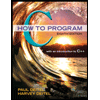
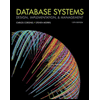
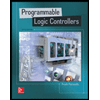