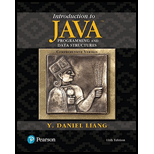
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 25, Problem 25.7PE
Program Plan Intro
Program Plan:
Include the required packages. Define the class “Test”.
- Define the driver method “main”.
- Declare the object for the “BST” class.
- Call the method “insert” to add string values to the tree.
- Call the “getNumberOfNonLeaves” method and display the result.
- Define the “BST” class.
- Define the “getNumberOfNonLeaves” method.
- Return the number of non-leaves node.
- Define the overridden method “getNumberOfNonLeaves”.
- If the “root” is “null”,
-
- return “0”.
- If the “root.left” or “root.right” is not “null”,
-
- Return the number of non-leaves node.
- Otherwise,
-
- return “0”.
- Declare the required variables.
- Create a constructor for “BST” class.
- Create a constructor for “BST” by passing array of objects.
- Define the “search” method to search the required data in the binary search tree.
- Define the “insert” method.
- If the root is null create the tree otherwise insert the value into left or right subtree.
- Define the “createNewNode”
- Return the result of new node creations.
- Define the “inorder”
- Inorder traverse from the root.
- Define the protected “inorder” method
- Traverse the tree according to the inorder traversal concept.
- Define the “postorder”
- Postorder traverse from the root.
- Define the protected “postorder” method
- Traverse the tree according to the postorder traversal concept.
- Define the “preorder”
- Preorder traverse from the root.
- Define the protected “preorder” method
- Traverse the tree according to the preorder traversal concept.
- Define the “TreeNode” class
- Declare the required variables.
- Define the constructor.
- Define the “getSize” method.
- Return the size.
- Define the “getRoot” method
- Return the root.
- Define the “java.util.ArrayList” method.
- Create an object for the array list.
- If the “current” is not equal to null, add the value to the list.
- If the “current” is less than 0, set the “current” as left subtree element otherwise set the “current” as right subtree element.
- Return the list.
- Define the “delete” method.
- If the “current” is not equal to null, add the value to the list.
- If the “current” is less than 0, delete the “current” as left subtree element otherwise delete the “current” as right subtree element.
- Return the list.
- Define the “iterator” method.
- Call the “inorderIterator” and return the value.
- Define the “inorderIterator”
- Create an object for that method and return the value
- Define the “inorderIterator” class.
- Declare the variables.
- Define the constructor.
-
- Call the “inorder” method.
- Define the “inorder” method.
-
- Call the inner “inorder” method with the argument.
- Define the TreeNode “inorder” method.
-
- If the root value is null return the value, otherwise add the value into the list.
- Define the “hasNext” method
-
- If the “current” value is less than size of the list return true otherwise return false.
- Define the “next” method
-
- Return the list.
- Define the “remove” method.
-
- Call the delete method.
- Clear the list then call the “inorder” method.
- Define the “clear” method
- Set the values to the variables
- Define the overridden method “getNumberOfNonLeaves”.
- Return the number of non-leaves node.
- Define the “getNumberOfNonLeaves” method.
- Define the interface.
- Declare the required methods.
- Define the required methods.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
PLEASE SOLVE STEP BY STEP WITHOUT ARTIFICIAL INTELLIGENCE OR CHATGPT
I don't understand why you use chatgpt, if I wanted to I would do it myself, I need to learn from you, not from being a d amn robot.
SOLVE STEP BY STEP I WANT THE DIAGRAM PERFECTLY IN SIMULINK
I need to develop and run a program that prompts the user to enter a positive integer n, and then calculate the value of n factorial n! = multiplication of all integers between 1 and n, and print the value n! on the screen. This is for C*.
I need to develop and run a C* program to sum up integers from 1 to 100, and print out the sum value on the screen. Can someone help please?
Chapter 25 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 25.2 - Prob. 25.2.1CPCh. 25.2 - Prob. 25.2.2CPCh. 25.2 - Prob. 25.2.3CPCh. 25.2 - Prob. 25.2.4CPCh. 25.2 - Prob. 25.2.5CPCh. 25.3 - Prob. 25.3.1CPCh. 25.3 - Prob. 25.3.2CPCh. 25.3 - Prob. 25.3.3CPCh. 25.3 - Prob. 25.3.4CPCh. 25.4 - Prob. 25.4.1CP
Ch. 25.4 - Prob. 25.4.2CPCh. 25.4 - Prob. 25.4.3CPCh. 25.4 - Prob. 25.4.4CPCh. 25.4 - Prob. 25.4.5CPCh. 25.5 - Prob. 25.5.1CPCh. 25.5 - Prob. 25.5.2CPCh. 25.5 - Prob. 25.5.3CPCh. 25.5 - Prob. 25.5.4CPCh. 25.5 - Prob. 25.5.5CPCh. 25.6 - Prob. 25.6.1CPCh. 25.6 - Prob. 25.6.2CPCh. 25.6 - Prob. 25.6.3CPCh. 25.6 - How do you replace lines 9499 in Listing 25.11...Ch. 25 - Prob. 25.1PECh. 25 - (Implement inorder traversal without using...Ch. 25 - (Implement preorder traversal without using...Ch. 25 - (Implement postorder traversal without using...Ch. 25 - Prob. 25.6PECh. 25 - Prob. 25.7PECh. 25 - (Implement bidirectional iterator) The...Ch. 25 - Prob. 25.9PECh. 25 - Prob. 25.10PECh. 25 - Prob. 25.11PECh. 25 - (Test BST) Design and write a complete test...Ch. 25 - (Modify BST using Comparator) Revise BST in...Ch. 25 - Prob. 25.15PECh. 25 - (Data compression: Huffman coding) Write a program...Ch. 25 - Prob. 25.17PECh. 25 - (Compress a file) Write a program that compresses...Ch. 25 - (Decompress a file) The preceding exercise...
Knowledge Booster
Similar questions
- Given the schema below for the widgetshop, provide a schema diagram. Schema name Attributes Widget-schema Customer-schema (stocknum, manufacturer, description, weight, price, inventory) (custnum, name, address) Purchased-schema (custnum, stocknum, pdate) Requestedby-schema (stocknum, custnum) Newitem-schema (stocknum, manufacturer, description) Employee-schema (ssn, name, address, salary) You can remove the Newitem-schema (red).arrow_forwardTrue or False: Given the sets F and G with F being an element of G, is it always ture that P(F) is an element of P(G)? (P(F) and P(G) mean power sets). Why?arrow_forwardCan you please simplify (the domain is not empty) ∃xF (x) → ¬∃x(F (x) ∨ ¬G(x)). Foarrow_forward
- HistogramUse par(mfrow=c(2,2)) and output 4 plots with different argument settings.arrow_forward(use R language)Scatter plot(a). Run the R code example, and look at the help file for plot() function. Try different values for arguments:type, pch, lty, lwd, col(b). Use par(mfrow=c(3,2)) and output 6 plots with different argument settings.arrow_forward1. Draw flow charts for each of the following;a) A system that reads three numbers and prints the value of the largest number.b) A system reads an employee name (NAME), overtime hours worked (OVERTIME), hours absent(ABSENT) and determines the bonus payment (PAYMENT).arrow_forward
- Scenario You work for a small company that exports artisan chocolate. Although you measure your products in kilograms, you often get orders in both pounds and ounces. You have decided that rather than have to look up conversions all the time, you could use Python code to take inputs to make conversions between the different units of measurement. You will write three blocks of code. The first will convert kilograms to pounds and ounces. The second will convert pounds to kilograms and ounces. The third will convert ounces to kilograms and pounds. The conversions are as follows: 1 kilogram = 35.274 ounces 1 kilogram = 2.20462 pounds 1 pound = 0.453592 kilograms 1 pound = 16 ounces 1 ounce = 0.0283 kilograms 1 ounce = 0.0625 pounds For the purposes of this activity the template for a function has been provided. You have not yet covered functions in the course, but they are a way of reusing code. Like a Python script, a function can have zero or more parameters. In the code window you…arrow_forwardmake a screen capture showing the StegExpose resultsarrow_forwardWhich of the following is not one of the recommended criteria for strategic objectives? Multiple Choice a) realistic b) appropriate c) sustainable d) measurablearrow_forward
- Management innovations such as total quality, benchmarking, and business process reengineering always lead to sustainable competitive advantage because everyone else is doing them. a) True b) Falsearrow_forwardVision statements are more specific than strategic objectives. a) True b) Falsearrow_forwardThe three components of the __________ approach to corporate accounting include financial, environmental, and social performance measures. Multiple Choice a) stakeholder b) triple dimension c) triple bottom line d) triple efficiencyarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
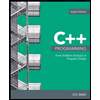
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
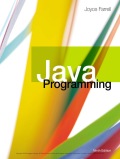
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
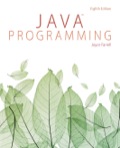
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
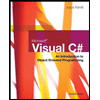
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
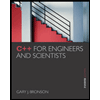
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr